Code Your Way to E-Commerce Excellence with WooCommerce Plugins
WooCommerce, a popular open-source e-commerce platform for WordPress, powers millions of online stores worldwide. One of the reasons for its widespread adoption is the availability of plugins that extend its functionality. If you’re considering developing a WooCommerce plugin or you’re already knee-deep in plugin development, it’s crucial to follow best practices to ensure your plugin is reliable, secure, and easy to maintain. In this blog post, we’ll explore some WooCommerce plugin development best practices, complete with examples.
1. Start with a Solid Plugin Foundation
Every great plugin begins with a strong foundation. Use the following best practices:
1. Object-Oriented Programming (OOP): Adopt an OOP approach to ensure clean and maintainable code. Create classes and methods to encapsulate functionality.
```php class My_WooCommerce_Plugin { public function __construct() { // Initialize your plugin here } } ```
2. Use Hooks and Filters: Leverage WooCommerce’s hooks and filters to interact with the core functionality.
```php add_action('woocommerce_after_checkout_form', 'my_custom_function'); function my_custom_function() { // Your code here } ```
2. Follow WordPress and WooCommerce Coding Standards
Adhering to coding standards ensures consistency and makes your code more readable. Use tools like PHPCS to check for compliance.
```php // Example of clean code following coding standards if ( $variable_name === 'value' ) { // Do something } ```
3. Optimize for Performance
Efficient code is essential for a smooth user experience. Avoid unnecessary database queries and optimize your code for speed.
```php // Bad practice: Multiple database queries in a loop foreach ( $products as $product ) { $price = get_post_meta( $product->get_id(), '_price', true ); // Do something with $price } // Good practice: Fetch data in a single query $product_ids = wp_list_pluck( $products, 'get_id' ); $prices = wc_get_product_ids_and_prices( $product_ids ); foreach ( $prices as $product_id => $price ) { // Do something with $price } ```
4. Prioritize Security
Security should be a top priority in plugin development. Follow these best practices:
1. Input Validation: Sanitize and validate user input to prevent SQL injection, cross-site scripting (XSS), and other vulnerabilities.
```php $custom_input = sanitize_text_field( $_POST['custom_input'] ); ```
2. Escaping Output: Use proper escaping functions when rendering data to the frontend to prevent XSS attacks.
```php echo esc_html( $data_to_render ); ```
5. Keep the User Experience in Mind
User experience is paramount. Ensure your plugin is intuitive and user-friendly.
- Provide Clear Documentation: Include comprehensive documentation that guides users through installation, configuration, and usage.
- Use Native WooCommerce UI: Integrate your plugin seamlessly into WooCommerce’s user interface for a consistent look and feel.
```php // Example: Adding a custom tab to the product edit page add_action( 'woocommerce_product_data_tabs', 'my_custom_product_tab' ); function my_custom_product_tab( $tabs ) { $tabs['my_tab'] = array( 'label' => __( 'My Custom Tab', 'textdomain' ), 'target' => 'my_custom_tab_content', 'priority' => 50, ); return $tabs; } ```
6. Plan for Extensibility
Design your plugin to be extensible, allowing other developers to customize and extend its functionality easily.
- Action and Filter Hooks: Add action and filter hooks to key parts of your code to allow other plugins or themes to modify behavior.
```php // Example: Allowing other plugins to modify the plugin's output $output = apply_filters( 'my_plugin_output', $output ); ```
7. Test Thoroughly
Testing is essential to catch bugs and ensure your plugin works as expected.
1. Unit Testing: Write unit tests for critical parts of your code using tools like PHPUnit.
```php // Example: PHPUnit test for a function public function test_my_function() { $result = my_function(); $this->assertEquals( 'expected_value', $result ); } ```
2. Functional Testing: Test your plugin’s functionality in a real-world environment to identify and fix any issues.
8. Version Control and Release Management
Implement version control with Git and use a platform like GitHub or Bitbucket to manage your codebase. Follow semantic versioning (SemVer) when releasing updates.
```shell $ git commit -m "Version 1.0.0" $ git tag 1.0.0 $ git push origin master --tags ```
9. Provide Excellent Support
Offer responsive support to users. Respond to their questions and issues promptly.
- Support Forums: Participate in the WooCommerce support forums to assist users with problems related to your plugin.
10. Regularly Update Your Plugin
Continuously update your plugin to stay compatible with the latest versions of WooCommerce and WordPress.
Conclusion
Developing a WooCommerce plugin can be a rewarding endeavor, but it requires careful planning and adherence to best practices. By following the guidelines outlined in this post, you’ll create a plugin that is reliable, secure, and easy to maintain. Remember, the key to successful WooCommerce plugin development is not just about writing code but also about providing value to the WooCommerce ecosystem and its users. Happy coding!
Table of Contents
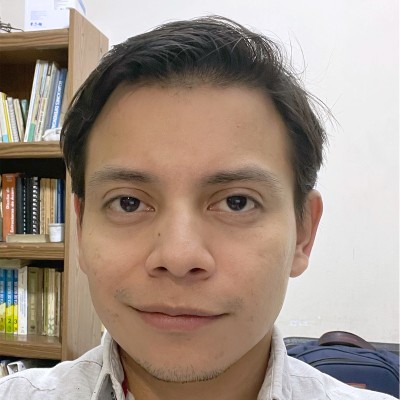
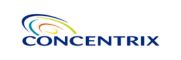