Yii AJAX Integration: Building Responsive Web Applications
AJAX (Asynchronous JavaScript and XML) is a powerful technology that allows web applications to communicate with the server asynchronously, without refreshing the entire page. This capability enables more dynamic, fast, and responsive user interfaces. Yii, a high-performance PHP framework, provides robust support for AJAX, making it easier to integrate dynamic functionalities into your applications. This article will guide you through implementing AJAX in Yii, using practical examples to illustrate how to enhance your web applications’ responsiveness.
Setting Up Yii for AJAX Integration
To get started with AJAX in Yii, you’ll need a basic Yii application set up and ready. Yii supports AJAX out of the box, but it’s essential to understand how to structure your controllers and views to handle AJAX requests effectively.
Example: Basic Yii Application Setup
1. Install Yii via Composer:
```bash composer create-project --prefer-dist yiisoft/yii2-app-basic myyiiapp ```
2. Set Up the Web Server:
Make sure your web server (like Apache or Nginx) is correctly configured to serve the Yii application.
3. Create a Controller for AJAX Handling:
```php namespace app\controllers; use Yii; use yii\web\Controller; use yii\web\Response; class SiteController extends Controller { public function actionIndex() { return $this->render('index'); } public function actionGetData() { Yii::$app->response->format = Response::FORMAT_JSON; $data = ['message' => 'Hello, this is your AJAX response!']; return $data; } } ```
Making AJAX Calls in Yii
The next step is to implement AJAX calls from the client side using JavaScript or jQuery. Yii provides built-in support for jQuery, making it straightforward to initiate AJAX requests.
Example: Making an AJAX Call with jQuery
In your view file (`index.php`), you can set up a button that triggers an AJAX call when clicked:
```php <?php use yii\helpers\Html; use yii\web\JsExpression; $this->title = 'Yii AJAX Integration'; ?> <div class="site-index"> <h1><?= Html::encode($this->title) ?></h1> <button id="ajax-button" class="btn btn-primary">Get AJAX Data</button> <div id="response"></div> </div> <?php $script = new JsExpression(" $('#ajax-button').on('click', function() { $.ajax({ url: '" . yii\helpers\Url::to(['site/get-data']) . "', type: 'GET', success: function(data) { $('#response').html('<p>' + data.message + '</p>'); }, error: function() { $('#response').html('<p>An error has occurred</p>'); } }); }); "); $this->registerJs($script); ?> ```
Handling AJAX Requests in Yii
When the client-side JavaScript triggers an AJAX request, Yii’s controller action (`actionGetData`) is invoked. You can return JSON, HTML, or any other data format that suits your application’s needs.
Example: Returning Data from a Controller Action
In the `SiteController`:
```php public function actionGetData() { Yii::$app->response->format = Response::FORMAT_JSON; $data = ['message' => 'This is your dynamically loaded data!']; return $data; } ```
Updating Part of the Page with AJAX
One of the primary benefits of using AJAX is the ability to update parts of a webpage without reloading the entire page. Yii provides convenient methods to render partial views.
Example: Rendering a Partial View with AJAX
1. Create a Partial View: Create a new file named `_data.php` in the `views/site` directory.
```php <p>This content was loaded dynamically with AJAX!</p> ```
2. Modify the AJAX Call to Load the Partial View:
In your view file (`index.php`):
```javascript $.ajax({ url: '" . yii\helpers\Url::to(['site/load-partial']) . "', type: 'GET', success: function(data) { $('#response').html(data); }, error: function() { $('#response').html('<p>An error has occurred</p>'); } }); ```
3. Create a Controller Action to Render the Partial View:
```php public function actionLoadPartial() { return $this->renderPartial('_data'); } ```
Handling AJAX Form Submissions
Yii also makes it easy to handle form submissions via AJAX. This approach is useful for enhancing user experience by providing real-time feedback without full-page reloads.
Example: Submitting a Form with AJAX
1. Create a Form in a View File (`_form.php`):
```php <?php use yii\widgets\ActiveForm; use yii\helpers\Html; ?> <?php $form = ActiveForm::begin(['id' => 'ajax-form']); ?> <?= $form->field($model, 'name')->textInput(['maxlength' => true]) ?> <?= $form->field($model, 'email')->input('email') ?> <div class="form-group"> <?= Html::submitButton('Submit', ['class' => 'btn btn-primary']) ?> </div> <?php ActiveForm::end(); ?> ```
2. Handle the Form Submission with JavaScript:
```javascript $('#ajax-form').on('beforeSubmit', function(e) { var form = $(this); $.ajax({ url: form.attr('action'), type: 'POST', data: form.serialize(), success: function(data) { $('#response').html('<p>Form submitted successfully!</p>'); }, error: function() { $('#response').html('<p>An error occurred while submitting the form.</p>'); } }); return false; // Prevent the form from submitting via the browser. }); ```
3. Process the AJAX Form Submission in the Controller:
```php public function actionSubmitForm() { $model = new MyModel(); if (Yii::$app->request->isAjax && $model->load(Yii::$app->request->post())) { Yii::$app->response->format = Response::FORMAT_JSON; return ActiveForm::validate($model); } if ($model->load(Yii::$app->request->post()) && $model->save()) { return $this->redirect(['view', 'id' => $model->id]); } return $this->render('create', ['model' => $model]); } ```
Conclusion
Integrating AJAX into your Yii applications allows you to build more dynamic and responsive web applications. By leveraging Yii’s built-in support for AJAX, you can create seamless user experiences that enhance your application’s interactivity without compromising performance. This guide has covered the basics of setting up AJAX in Yii, handling AJAX requests and responses, updating page content dynamically, and managing form submissions with AJAX.
Further Reading:
Table of Contents
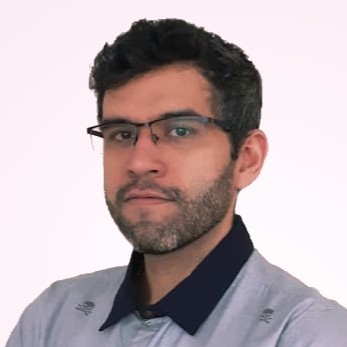
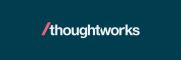