Yii and AngularJS: Creating Dynamic Web Apps
Dynamic web applications demand a responsive and interactive user experience, often requiring a robust backend and a responsive frontend. Combining Yii Framework, a powerful PHP framework, with AngularJS, a dynamic JavaScript framework, allows developers to create efficient and scalable web applications. This article explores how to integrate Yii and AngularJS to build dynamic web apps.
Setting Up Yii Framework
Yii is a high-performance PHP framework best suited for developing large-scale web applications. Start by setting up Yii and creating a basic application to serve as your backend.
Example: Setting Up a Yii Application
```bash composer create-project --prefer-dist yiisoft/yii2-app-basic basic-app ```
Once Yii is installed, configure the application by updating the configuration files, including database settings and any necessary components.
Example: Configuring Database in Yii
```php 'components' => [ 'db' => [ 'class' => 'yii\db\Connection', 'dsn' => 'mysql:host=localhost;dbname=your_database', 'username' => 'root', 'password' => '', 'charset' => 'utf8', ], // other components ], ```
Setting Up AngularJS
AngularJS, a JavaScript framework developed by Google, is ideal for building dynamic single-page applications. Create a basic AngularJS application and configure it to interact with your Yii backend.
Example: Setting Up an AngularJS Application
Include AngularJS in your project via CDN or download it and add it to your project:
```html <!DOCTYPE html> <html ng-app="myApp"> <head> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body ng-controller="MainController"> <h1>{{ message }}</h1> <script> angular.module('myApp', []) .controller('MainController', function($scope) { $scope.message = 'Hello from AngularJS!'; }); </script> </body> </html> ```
Integrating Yii with AngularJS
To create a dynamic web app, your AngularJS frontend needs to communicate with the Yii backend via RESTful APIs. Yii’s built-in support for REST APIs makes it easy to set up endpoints for AngularJS to consume.
Example: Creating a REST API in Yii
```php namespace app\controllers; use yii\rest\ActiveController; class UserController extends ActiveController { public $modelClass = 'app\models\User'; } ```
Update your Yii configuration to enable CORS (Cross-Origin Resource Sharing) so that your AngularJS frontend can make requests to the Yii backend.
Example: Enabling CORS in Yii
```php 'components' => [ 'corsFilter' => [ 'class' => \yii\filters\Cors::class, ], // other components ], ```
Example: Consuming Yii API from AngularJS
```javascript angular.module('myApp', []) .controller('UserController', function($scope, $http) { $http.get('http://localhost/basic-app/web/user') .then(function(response) { $scope.users = response.data; }); }); ```
Building Dynamic Features
With the backend and frontend integrated, you can now build dynamic features such as user authentication, data manipulation, and real-time updates.
Example: Implementing User Authentication
- Backend (Yii): Create endpoints for user login and registration.
```php namespace app\controllers; use yii\web\Controller; use Yii; class AuthController extends Controller { public function actionLogin() { $request = Yii::$app->request->post(); // Authentication logic here } } ```
- Frontend (AngularJS): Create a service to handle authentication.
```javascript angular.module('myApp') .service('AuthService', function($http) { this.login = function(credentials) { return $http.post('http://localhost/basic-app/web/auth/login', credentials); }; }); ```
Conclusion
Combining Yii and AngularJS provides a powerful stack for building dynamic web applications. Yii’s robust backend capabilities paired with AngularJS’s dynamic frontend features create a seamless and interactive user experience. By setting up Yii for API development and AngularJS for frontend interaction, you can build scalable and responsive web applications that meet modern user expectations.
Further Reading:
Table of Contents
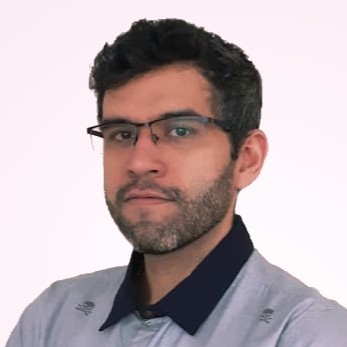
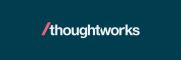