Yii Authentication and Authorization: Securing Your Application
Authentication and authorization are crucial components for securing any web application. Yii, a high-performance PHP framework, provides comprehensive tools for managing user authentication and authorization efficiently. This article will explore how to implement robust security mechanisms in Yii applications, ensuring both user data integrity and controlled access to resources.
Setting Up Authentication in Yii
To secure your Yii application, you’ll first need to set up a basic Yii project and configure user authentication. Yii offers a flexible identity management system, allowing you to define how user identities are authenticated and stored.
Example: Configuring User Identity in Yii
To begin, create a basic Yii application using Composer:
```bash composer create-project --prefer-dist yiisoft/yii2-app-basic my-app cd my-app ```
Next, configure the user identity in the `config/web.php` file by specifying the `user` component:
```php 'components' => [ 'user' => [ 'identityClass' => 'app\models\User', 'enableAutoLogin' => true, ], ], ```
You’ll also need to implement the `IdentityInterface` in your `User` model to define how users are authenticated.
Implementing User Authentication
Yii’s authentication process involves verifying a user’s identity using credentials such as a username and password. Once verified, Yii generates a session or token to track the user’s authentication state.
Example: User Login Action
In the `SiteController`, add a `login` action to handle user authentication:
```php public function actionLogin() { $model = new LoginForm(); if ($model->load(Yii::$app->request->post()) && $model->login()) { return $this->goBack(); } return $this->render('login', [ 'model' => $model, ]); } ``` In the `LoginForm` model, implement the `login` method to authenticate the user: ```php public function login() { if ($this->validate()) { return Yii::$app->user->login($this->getUser(), $this->rememberMe ? 3600*24*30 : 0); } return false; } ```
Implementing Authorization with Access Control
Authorization in Yii determines whether a user has permission to access a particular resource or perform a specific action. Yii provides Role-Based Access Control (RBAC) and Access Control Filters (ACF) to manage authorization.
Example: Setting Up Access Control Filters
In the `SiteController`, use ACF to restrict access to specific actions:
```php public function behaviors() { return [ 'access' => [ 'class' => \yii\filters\AccessControl::class, 'only' => ['logout', 'admin'], 'rules' => [ [ 'allow' => true, 'actions' => ['logout'], 'roles' => ['@'], ], [ 'allow' => true, 'actions' => ['admin'], 'roles' => ['admin'], ], ], ], ]; } ```
Here, `only` specifies the actions the filter applies to, while `rules` defines the access permissions based on user roles.
Implementing Role-Based Access Control (RBAC)
RBAC allows for more complex permission management by assigning roles to users and defining access rules for each role. Yii’s RBAC system provides a powerful way to manage user permissions in large applications.
Example: Configuring RBAC
To set up RBAC, configure the `authManager` component in `config/web.php`:
```php 'components' => [ 'authManager' => [ 'class' => 'yii\rbac\DbManager', ], ], ```
Next, create roles, permissions, and assign them to users using migration scripts or via the console:
```php use Yii; use yii\console\Controller; use app\models\User; class RbacController extends Controller { public function actionInit() { $auth = Yii::$app->authManager; // Add "admin" role $admin = $auth->createRole('admin'); $auth->add($admin); // Add "manageUsers" permission $manageUsers = $auth->createPermission('manageUsers'); $manageUsers->description = 'Manage users'; $auth->add($manageUsers); // Assign permission to role $auth->addChild($admin, $manageUsers); // Assign role to user $auth->assign($admin, User::findOne(['username' => 'admin'])->id); } } ```
Implementing Advanced Security Features
Yii also allows for additional security features such as two-factor authentication (2FA), rate limiting, and IP-based restrictions.
Example: Implementing Two-Factor Authentication
Yii does not have built-in support for 2FA, but you can integrate third-party libraries like `yiisoft/yii2-authclient` or custom implementations using OTP libraries.
Refreshing Sessions and Managing Expiry
Yii sessions expire based on configuration settings. You can set session expiry and automatic renewal to ensure a smooth user experience.
Example: Configuring Session Timeout
```php 'session' => [ 'class' => 'yii\web\Session', 'timeout' => 3600, // 1 hour ], ```
Logging Out and Revoking Access
To log out users and invalidate sessions, use the `logout` method provided by Yii:
Example: User Logout
```php public function actionLogout() { Yii::$app->user->logout(); return $this->goHome(); } ```
Conclusion
Securing a Yii application involves robust authentication and authorization mechanisms. By implementing user identity management, access control, role-based permissions, and additional security features, you can build secure, scalable, and high-performance web applications.
Further Reading:
Table of Contents
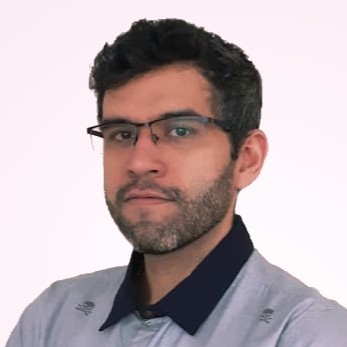
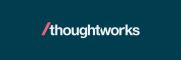