Yii Caching Strategies: Improving Application Speed and Efficiency
Caching is a crucial technique for improving the performance of web applications by storing frequently accessed data to reduce the load on your database and server. Yii, a powerful PHP framework, offers several caching strategies that can help you optimize your application’s speed and efficiency. This article explores various caching strategies available in Yii and how to implement them effectively.
Setting Up Yii Caching
To start using caching in Yii, you’ll need to configure caching components in your Yii application. Yii provides support for different caching methods, including file-based caching, database caching, and more.
Example: Configuring Yii Caching
```php // config/web.php return [ // other configurations... 'components' => [ 'cache' => [ 'class' => 'yii\caching\FileCache', ], // other components... ], ]; ```
Using File Cache
File caching is one of the simplest caching methods in Yii. It stores cached data in files on the server’s filesystem. This method is suitable for small to medium-sized applications.
Example: Implementing File Caching
```php use Yii; $data = Yii::$app->cache->get('myData'); if ($data === false) { // Data is not in cache, fetch it from the database $data = MyModel::find()->all(); // Store data in cache for future requests Yii::$app->cache->set('myData', $data, 3600); // Cache for 1 hour } // Use the data ```
Database Caching
Database caching is another effective caching method, particularly useful when you need to store cached data in a database table. This method can be beneficial for large-scale applications where file-based caching might not be efficient.
Example: Implementing Database Caching
```php use Yii; $data = Yii::$app->cache->get('myData'); if ($data === false) { // Data is not in cache, fetch it from the database $data = MyModel::find()->all(); // Store data in cache Yii::$app->cache->set('myData', $data, 3600); // Cache for 1 hour } // Use the data ```
Using APCu Cache
APCu (Alternative PHP Cache User) is an in-memory caching method that can significantly boost performance for applications with high read and write operations. It stores data in memory, reducing the need to read from disk.
Example: Configuring APCu Cache
```php // config/web.php return [ // other configurations... 'components' => [ 'cache' => [ 'class' => 'yii\caching\ApcCache', ], // other components... ], ]; ```
Caching Query Results
Caching query results can help reduce database load and improve application performance. Yii provides ways to cache database query results efficiently.
Example: Caching Query Results
```php use Yii; use app\models\MyModel; $dataProvider = new \yii\data\ActiveDataProvider([ 'query' => MyModel::find(), 'pagination' => [ 'pageSize' => 20, ], ]); // Cache the query result $dataProvider->query->cache(3600); // Cache for 1 hour ```
Implementing Cache Dependency
Cache dependencies allow you to control when cached data should be invalidated based on specific conditions. This helps ensure that your cache remains fresh and relevant.
Example: Using Cache Dependency
```php use yii\caching\DbDependency; $dependency = new DbDependency([ 'sql' => 'SELECT MAX(updated_at) FROM my_table', ]); $data = Yii::$app->cache->getOrSet('myData', function () { return MyModel::find()->all(); }, 3600, $dependency); // Cache for 1 hour ```
Conclusion
Implementing effective caching strategies in Yii can significantly improve the performance and efficiency of your web applications. By using file caching, database caching, APCu caching, caching query results, and cache dependencies, you can optimize your application’s response time and reduce server load.
Further Reading:
Table of Contents
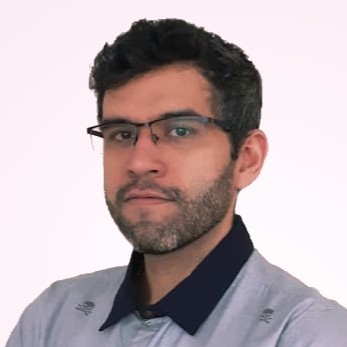
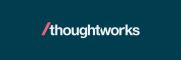