Yii Dependency Injection: Managing Component Dependencies
Dependency Injection (DI) is a powerful design pattern that facilitates loose coupling in software architecture, making your application more modular, testable, and maintainable. Yii, a high-performance PHP framework, supports dependency injection to help developers manage component dependencies efficiently. This article dives into how to implement dependency injection in Yii to enhance your application’s flexibility and modularity.
Understanding Dependency Injection in Yii
Dependency Injection is a technique where a class receives its dependencies from an external source rather than creating them itself. In Yii, DI is implemented through the Yii service container, which is a dependency injection container that manages dependencies between different components.
Setting Up Dependency Injection in Yii
To get started with DI in Yii, you’ll first need to configure the Yii service container to manage your application’s dependencies. The service container allows you to define and retrieve components or objects that your application depends on.
Example: Configuring the Yii Service Container
```php use Yii; use app\components\MyComponent; // Register MyComponent with the container Yii::$container->set('app\components\MyComponent', [ 'class' => 'app\components\MyComponent', 'property1' => 'value1', 'property2' => 'value2', ]); // Now, when you call Yii::createObject(), the container will inject the dependencies $component = Yii::createObject('app\components\MyComponent'); ```
Creating and Using Dependencies
In Yii, you can define dependencies for components and services directly in the service container configuration. When these components are requested, the service container will automatically resolve and inject the necessary dependencies.
Example: Defining a Component with Dependencies
Let’s consider a scenario where a `UserService` depends on a `UserRepository`:
```php use app\services\UserService; use app\repositories\UserRepository; // Register UserRepository Yii::$container->set('app\repositories\UserRepository', [ 'class' => 'app\repositories\UserRepository', ]); // Register UserService and inject UserRepository Yii::$container->set('app\services\UserService', [ 'class' => 'app\services\UserService', 'userRepository' => Yii::$container->get('app\repositories\UserRepository'), ]); // Retrieve UserService with its dependencies resolved $userService = Yii::createObject('app\services\UserService'); ```
Constructor Injection
Yii supports constructor injection, where dependencies are passed through the constructor of the class. This approach provides a clear way to define required dependencies for a class.
Example: Using Constructor Injection
```php namespace app\services; class UserService { private $userRepository; public function __construct($userRepository) { $this->userRepository = $userRepository; } // Service methods here } // Registering the service with constructor injection Yii::$container->set('app\services\UserService', [ 'class' => 'app\services\UserService', 'userRepository' => Yii::$container->get('app\repositories\UserRepository'), ]); ```
Property Injection
Yii also supports property injection, where dependencies are set directly on the properties of a class. This can be useful when you have optional dependencies or want to inject dependencies that do not need to be set through the constructor.
Example: Using Property Injection
```php namespace app\services; class NotificationService { public $mailer; public function sendNotification($message) { $this->mailer->send($message); } } // Register NotificationService with property injection Yii::$container->set('app\services\NotificationService', [ 'class' => 'app\services\NotificationService', 'mailer' => Yii::$container->get('app\components\Mailer'), ]); ```
Injecting Dependencies into Actions
Yii allows injecting dependencies directly into controller actions, which provides a convenient way to manage dependencies within your controllers without manually instantiating components.
Example: Injecting Dependencies into Controller Actions
```php namespace app\controllers; use Yii; use yii\web\Controller; use app\services\UserService; class UserController extends Controller { public function actionIndex(UserService $userService) { // The UserService dependency is automatically injected $users = $userService->getAllUsers(); return $this->render('index', ['users' => $users]); } } ```
Benefits of Using Dependency Injection in Yii
– Decoupling: Components are not tightly coupled to their dependencies, making your code more modular and flexible.
– Testability: DI makes unit testing easier by allowing you to inject mock objects.
– Reusability: Components with injected dependencies are more reusable as they are not bound to specific implementations.
– Maintenance: Managing dependencies centrally improves maintainability and makes refactoring easier.
Conclusion
Implementing dependency injection in Yii applications enhances the flexibility, modularity, and testability of your code. By configuring dependencies through the Yii service container, using constructor and property injection, and leveraging action injection in controllers, you can build more robust and maintainable PHP applications.
Further Reading:
Table of Contents
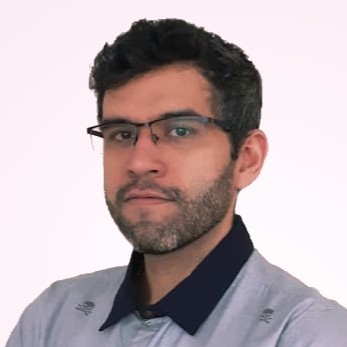
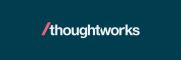