Yii Deployment Best Practices: Launching Your Application Successfully
Deploying a Yii application involves several crucial steps to ensure it runs smoothly and securely in a production environment. Proper deployment practices can prevent common issues, enhance performance, and ensure a successful launch. This article provides a comprehensive guide to Yii deployment best practices, from environment configuration to post-deployment checks.
Setting Up Your Yii Environment
Before deploying your Yii application, it’s essential to prepare your environment for production. This includes configuring your server, database, and application settings to optimize performance and security.
Example: Configuring Yii for Production
```php // In config/web.php or config/main.php return [ 'components' => [ 'db' => [ 'class' => 'yii\db\Connection', 'dsn' => 'mysql:host=localhost;dbname=your_database', 'username' => 'your_username', 'password' => 'your_password', 'charset' => 'utf8', ], 'urlManager' => [ 'enablePrettyUrl' => true, 'showScriptName' => false, 'rules' => [ // Define your URL rules here ], ], ], 'params' => [ // Your application parameters ], ]; ```
Setting Up a Web Server
Configuring your web server is a critical step in deploying a Yii application. Ensure that your server is properly set up to handle PHP and Yii’s requirements.
Example: Nginx Configuration for Yii
```nginx server { listen 80; server_name yourdomain.com; root /path/to/your/yii/application/web; index index.php; location / { try_files $uri $uri/ /index.php?$args; } location ~ \.php$ { include snippets/fastcgi-php.conf; fastcgi_pass unix:/var/run/php/php7.4-fpm.sock; fastcgi_index index.php; fastcgi_param SCRIPT_FILENAME $document_root$fastcgi_script_name; include fastcgi_params; } location ~ /\.ht { deny all; } } ```
Database Migration and Seeding
Ensure that your database schema is up to date and any required data is seeded before the application goes live.
Example: Running Yii Migrations
```bash php yii migrate ```
Securing Your Yii Application
Security is paramount in any deployment. Implement best practices to secure your Yii application from potential threats.
Example: Updating Security Settings
```php // In config/web.php return [ 'components' => [ 'request' => [ 'cookieValidationKey' => 'your_secure_key', 'csrfParam' => '_csrf-backend', ], 'security' => [ 'passwordHashStrategy' => \yii\base\Security::PASSWORD_HASH_DEFAULT, ], ], ]; ```
Performance Optimization
Optimizing your Yii application for performance can significantly improve user experience. Implement caching and optimize your code to handle high traffic efficiently.
Example: Configuring Yii Caching
```php // In config/web.php return [ 'components' => [ 'cache' => [ 'class' => 'yii\caching\FileCache', ], ], ]; ```
Post-Deployment Checks
After deploying your Yii application, perform a series of checks to ensure everything is functioning correctly. Verify that your application is accessible, the database is connected, and no errors are present in the logs.
Example: Checking Logs
```bash tail -f /path/to/your/yii/application/runtime/logs/app.log ```
Conclusion
Deploying a Yii application requires careful planning and execution to ensure a smooth and successful launch. By setting up your environment properly, configuring your web server, migrating and seeding your database, securing your application, optimizing performance, and performing post-deployment checks, you can achieve a reliable and efficient deployment.
Further Reading:
Table of Contents
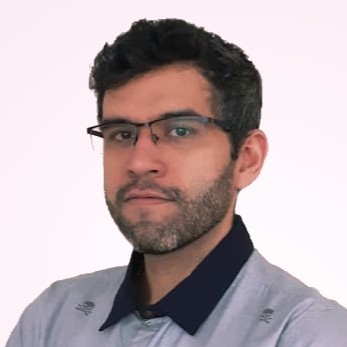
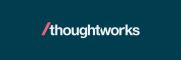