Yii Error Handling: Effective Strategies for Bug-Free Applications
Error handling is a critical aspect of developing robust web applications. In Yii, a powerful PHP framework, effective error handling ensures that your application can gracefully manage unexpected issues and provide meaningful feedback to users. This article delves into strategies for error handling in Yii to help you build bug-free applications.
Setting Up Yii Error Handling
Yii provides built-in support for error handling, which you can customize according to your application’s needs. To get started, ensure you have a Yii application set up and understand the basic error handling configuration.
Example: Basic Yii Error Handling Configuration
In Yii, you configure error handling in the `config/web.php` file. Here, you can define how exceptions and errors are managed.
```php 'components' => [ 'errorHandler' => [ 'errorAction' => 'site/error', ], // Other components... ], ```
Handling Exceptions
Exceptions are a common way to handle errors in Yii. You can create custom exception handlers to manage specific error conditions.
Example: Creating a Custom Exception Handler
```php class CustomException extends \Exception { public function report() { // Custom logic for reporting exceptions } } try { // Code that may throw an exception } catch (CustomException $e) { $e->report(); // Handle the exception } ```
Logging Errors
Logging is crucial for tracking errors and diagnosing issues. Yii integrates with various logging systems, allowing you to log errors to files, databases, or external services.
Example: Configuring Error Logging in Yii
In the `config/web.php` file, configure the `log` component to handle error logging.
```php 'components' => [ 'log' => [ 'traceLevel' => YII_DEBUG ? 3 : 0, 'targets' => [ [ 'class' => 'yii\log\FileTarget', 'levels' => ['error', 'warning'], 'logFile' => '@runtime/logs/app.log', ], ], ], // Other components... ], ```
Handling HTTP Errors
Yii provides a way to handle HTTP errors like 404 (Not Found) and 500 (Internal Server Error). Customize the error pages to improve user experience and provide clear feedback.
Example: Customizing Error Pages
Create custom views for error pages in the `views/site` directory:
– `error.php` (default error page)
– `404.php` (page not found)
– `500.php` (internal server error)
Configure error handling to use these views:
```php public function actionError() { $exception = Yii::$app->errorHandler->exception; if ($exception !== null) { if ($exception instanceof \yii\web\NotFoundHttpException) { return $this->render('404'); } elseif ($exception instanceof \yii\web\HttpException) { return $this->render('error', ['exception' => $exception]); } } return $this->render('error'); } ```
Debugging and Development
During development, Yii provides detailed error messages and stack traces to help you identify issues quickly. Ensure debugging is enabled in your development environment.
Example: Enabling Debugging
Set the `YII_DEBUG` constant to `true` in your `index.php` file:
```php defined('YII_DEBUG') or define('YII_DEBUG', true); ```
Conclusion
Effective error handling in Yii involves setting up proper configurations, creating custom exception handlers, logging errors, customizing error pages, and utilizing debugging tools. By implementing these strategies, you can ensure that your Yii-based applications are robust, reliable, and user-friendly.
Further Reading:
Table of Contents
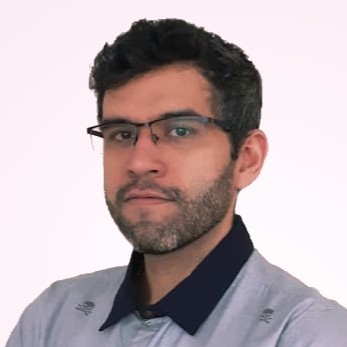
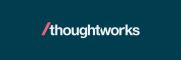