Yii Error Handling: Dealing with Exceptions and Logging
Handling errors and exceptions is a critical aspect of developing robust applications. Yii, a popular PHP framework, offers comprehensive tools and practices for managing errors and logging in your application. This article will guide you through the essentials of error handling and logging in Yii, providing practical examples to enhance your application’s reliability and maintainability.
Setting Up Yii Error Handling
Yii provides built-in mechanisms to handle exceptions and errors gracefully. You can customize how errors are managed by configuring error handling components and utilizing Yii’s logging capabilities.
Example: Configuring Error Handling in Yii
In Yii, error handling can be configured in the application configuration file (`config/web.php` or `config/main.php`). Here’s how you can set up custom error handling:
```php 'components' => [ 'errorHandler' => [ 'errorAction' => 'site/error', ], ], ```
This configuration directs Yii to use the `site/error` action for handling errors.
Handling Exceptions with Custom Error Actions
To provide a user-friendly experience, you can create a custom error action that displays a meaningful error page or message.
Example: Custom Error Action in Controller
```php public function actionError() { $exception = Yii::$app->errorHandler->exception; if ($exception !== null) { return $this->render('error', ['exception' => $exception]); } } ```
Logging Errors and Exceptions
Yii offers a powerful logging mechanism to record errors and exceptions. You can configure different logging targets and levels to suit your needs.
Example: Configuring Logging in Yii
In your application configuration, you can set up logging as follows:
```php 'components' => [ 'log' => [ 'targets' => [ [ 'class' => 'yii\log\FileTarget', 'levels' => ['error', 'warning'], 'logFile' => '@runtime/logs/app.log', ], ], ], ], ```
This configuration writes error and warning logs to a file named `app.log` in the runtime logs directory.
Creating Custom Loggers
You might need to create custom loggers for specific use cases, such as logging to an external service or database.
Example: Custom Logger in Yii
```php class CustomLogger extends \yii\log\Target { public function export() { foreach ($this->messages as $message) { // Custom logic to handle the log message $this->logToExternalService($message); } } private function logToExternalService($message) { // Logic to send log message to an external service } } // Add the custom logger to configuration 'components' => [ 'log' => [ 'targets' => [ [ 'class' => 'app\components\CustomLogger', 'levels' => ['error'], ], ], ], ], ```
Handling Specific Errors
You may need to handle specific errors differently based on their type or context. Yii allows you to manage this through custom exception handling.
Example: Handling Specific Exception Types
```php public function actionError() { $exception = Yii::$app->errorHandler->exception; if ($exception instanceof \yii\web\NotFoundHttpException) { return $this->render('404'); } elseif ($exception instanceof \yii\web\ForbiddenHttpException) { return $this->render('403'); } return $this->render('error', ['exception' => $exception]); } ```
Conclusion
Effective error handling and logging are crucial for building stable and maintainable Yii applications. By configuring error handling, utilizing Yii’s logging capabilities, and implementing custom loggers, you can enhance the reliability of your applications and gain valuable insights into their operation.
Further Reading:
Table of Contents
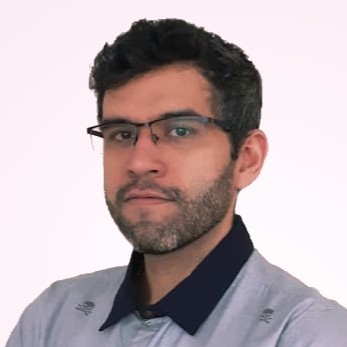
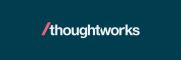