Yii and React: Integrating Frontend Components with Ease
Combining Yii, a robust PHP framework, with React, a popular JavaScript library, offers a powerful approach to building dynamic and interactive web applications. Yii handles the backend while React takes care of the frontend, enabling developers to leverage the strengths of both technologies. This article will guide you through the process of integrating Yii with React, providing a seamless experience for building modern web applications.
Setting Up Yii and React
To integrate Yii with React, you first need to set up a Yii application and then configure React within it. This typically involves setting up Yii for the backend and creating a React application that communicates with Yii’s APIs.
Example: Setting Up Yii
- Install Yii via Composer
```bash composer create-project --prefer-dist yiisoft/yii2-app-basic yii-react-app ```
- Set Up a Basic Yii Application
Edit `config/web.php` to configure your Yii application. For example:
```php return [ 'id' => 'app-backend', 'basePath' => dirname(__DIR__), 'components' => [ 'db' => [ 'class' => 'yii\db\Connection', 'dsn' => 'mysql:host=localhost;dbname=mydatabase', 'username' => 'root', 'password' => '', 'charset' => 'utf8', ], ], ]; ```
Example: Setting Up React
- Create a React Application
In the root of your Yii application directory, create a React app using Create React App:
```bash npx create-react-app frontend ```
- Configure React
Navigate to the `frontend` directory and start the React development server:
```bash cd frontend npm start ```
- Serve React from Yii
To serve React within Yii, build the React app and move the build files to the Yii `web` directory.
```bash npm run build cp -r build/* ../web/ ```
Creating and Using APIs in Yii
React components will interact with your Yii backend through APIs. Define these APIs in Yii to handle requests from your React frontend.
Example: Creating an API Endpoint in Yii
- Create a Controller
Create a new API controller in `controllers/ApiController.php`:
```php namespace app\controllers; use yii\rest\Controller; class ApiController extends Controller { public function actionIndex() { return ['message' => 'Hello from Yii']; } } ```
- Configure API Routes
Add the route to `config/web.php`:
```php 'urlManager' => [ 'enablePrettyUrl' => true, 'showScriptName' => false, 'rules' => [ 'GET api/index' => 'api/index', ], ], ```
Integrating React Components with Yii APIs
Once your APIs are set up, you can call them from React components to fetch or send data.
Example: Fetching Data in React
- Create a React Component
In `src/App.js`, create a component that fetches data from the Yii API:
```javascript import React, { useState, useEffect } from 'react'; function App() { const [message, setMessage] = useState(''); useEffect(() => { fetch('/api/index') .then(response => response.json()) .then(data => setMessage(data.message)); }, []); return ( <div> <h1>{message}</h1> </div> ); } export default App; ```
- Run Your Application
Ensure both Yii and React servers are running. React should now be able to make API requests to Yii and display the data.
Handling Authentication
For secure interactions between React and Yii, you should implement authentication mechanisms. Typically, this involves using tokens or session-based authentication.
Example: Implementing Token Authentication
- Create Authentication Endpoints in Yii
Add login and token generation to `ApiController`:
```php public function actionLogin() { $username = Yii::$app->request->post('username'); $password = Yii::$app->request->post('password'); // Perform authentication and generate token // Return the token as JSON } ```
- Store and Send Token in React
Store the token in local storage and include it in API requests:
```javascript const token = localStorage.getItem('token'); fetch('/api/index', { headers: { 'Authorization': `Bearer ${token}` } }) .then(response => response.json()) .then(data => setMessage(data.message)); ```
Conclusion
Integrating Yii with React combines the best of both worlds, offering a robust backend with a dynamic frontend. By setting up Yii for API management and React for interactive components, you can create modern web applications that are both powerful and user-friendly.
Further Reading:
– Yii Framework Documentation
– React Documentation
– Integrating React with Yii for Advanced Use Cases
Table of Contents
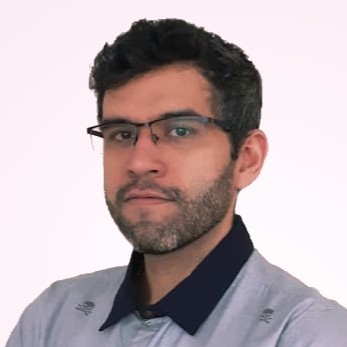
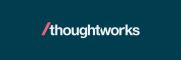