Yii Internationalization and Localization: Reaching Global Audiences
Internationalization (i18n) and localization (l10n) are essential for creating applications that can reach a global audience. Yii, a popular PHP framework, offers robust tools for handling these aspects, allowing you to build applications that adapt to different languages and regional preferences. This article will guide you through implementing i18n and l10n in Yii to enhance the global accessibility of your applications.
Setting Up Yii for Internationalization and Localization
To begin with internationalizing and localizing your Yii application, you’ll need to configure Yii to support multiple languages and regions. This involves setting up language files and configuring components to handle translations.
Example: Configuring Yii for i18n and l10n
```php // config/web.php return [ 'components' => [ 'i18n' => [ 'translations' => [ '*' => [ 'class' => 'yii\i18n\PhpMessageSource', 'basePath' => '@app/messages', 'allowAliases' => true, ], ], ], ], ]; ```
Creating Translation Files
To support multiple languages, you’ll need to create translation files that contain the text for different languages. Yii uses message source files to handle translations.
Example: Creating a Translation File for English
```php // messages/en/site.php return [ 'Welcome' => 'Welcome', 'Thank you for visiting our website' => 'Thank you for visiting our website', ]; ```
Example: Creating a Translation File for Spanish
```php // messages/es/site.php return [ 'Welcome' => 'Bienvenido', 'Thank you for visiting our website' => 'Gracias por visitar nuestro sitio web', ]; ```
Translating Text in Views
Once you have your translation files set up, you can use Yii’s translation functions in your views to display text in the appropriate language.
Example: Translating Text in a View
```php // views/site/index.php use Yii; echo Yii::t('site', 'Welcome'); echo Yii::t('site', 'Thank you for visiting our website'); ```
Handling Date and Time Localization
Localization isn’t limited to text translation; it also involves adapting date, time, and number formats to fit regional preferences. Yii provides tools for handling these aspects.
Example: Formatting Date and Time
```php // controllers/SiteController.php public function actionIndex() { $date = Yii::$app->formatter->asDate('2024-08-26', 'long'); return $this->render('index', ['date' => $date]); } // views/site/index.php echo "Today's date is: " . $date; ```
Switching Between Languages
To offer users the ability to switch between different languages, you’ll need to implement a mechanism for changing the application’s language dynamically.
Example: Switching Language in Yii
```php // controllers/SiteController.php public function actionSetLanguage($language) { Yii::$app->language = $language; return $this->redirect(Yii::$app->request->referrer); } // views/site/index.php echo \yii\helpers\Html::a('English', ['site/set-language', 'language' => 'en']); echo \yii\helpers\Html::a('Español', ['site/set-language', 'language' => 'es']); ```
Testing Internationalization and Localization
To ensure that your application is properly internationalized and localized, test it with different languages and regional settings. Yii’s i18n component makes it easy to switch languages and verify translations.
Example: Testing Translations
```php // Use Yii's built-in tools or third-party testing tools to verify that translations appear correctly ```
Conclusion
Implementing internationalization and localization in Yii allows you to build applications that are accessible to users from various linguistic and regional backgrounds. By configuring Yii for i18n and l10n, creating and managing translation files, handling date and time formatting, and providing language-switching options, you can enhance the global reach of your applications.
Further Reading:
Table of Contents
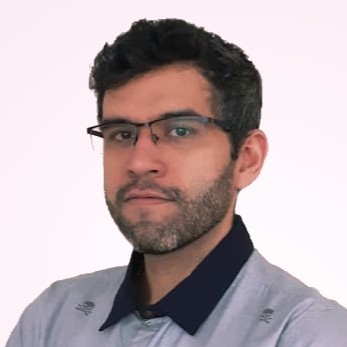
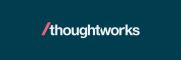