Yii Logging and Debugging Tools: Simplifying Development
Logging and debugging are essential for maintaining and improving web applications. Yii, a powerful PHP framework, provides a range of tools to streamline these processes. This article delves into Yii’s logging and debugging tools, helping you manage errors, optimize performance, and enhance development efficiency.
Setting Up Yii for Logging and Debugging
To leverage Yii’s logging and debugging capabilities, ensure you have a basic Yii application set up. Yii’s logging and debugging features are integrated into the framework, making them easy to configure and use.
Example: Basic Yii Setup
```php <?php // web/index.php require(__DIR__ . '/../vendor/autoload.php'); require(__DIR__ . '/../vendor/yiisoft/yii2/Yii.php'); require(__DIR__ . '/../config/bootstrap.php'); $config = require(__DIR__ . '/../config/web.php'); (new yii\web\Application($config))->run(); ```
Configuring Yii Logging
Yii offers a flexible logging system that can be tailored to meet your application’s needs. You can configure multiple log targets to handle different types of log messages.
Example: Configuring Logging in Yii
```php <?php // config/web.php return [ // Other configurations... 'components' => [ 'log' => [ 'traceLevel' => YII_DEBUG ? 3 : 0, 'targets' => [ [ 'class' => 'yii\log\FileTarget', 'levels' => ['error', 'warning'], 'categories' => ['yii\db\*'], 'logFile' => '@runtime/logs/db.log', 'maxFileSize' => 1024 * 2, 'maxLogFiles' => 5, ], [ 'class' => 'yii\log\FileTarget', 'levels' => ['info'], 'categories' => ['application'], 'logFile' => '@runtime/logs/application.log', ], ], ], ], ]; ```
Using Yii Debug Toolbar
The Yii Debug Toolbar provides a powerful and user-friendly interface for debugging. It offers insights into your application’s performance, database queries, and more.
Example: Enabling the Debug Toolbar
```php <?php // config/web.php return [ // Other configurations... 'components' => [ 'db' => [ // Database configuration... ], 'debug' => [ 'class' => 'yii\debug\Module', 'allowedIPs' => ['127.0.0.1', '::1'], ], ], ]; ```
Monitoring Performance
Monitoring performance is crucial for identifying bottlenecks and optimizing your application. Yii’s built-in profiling tools allow you to measure execution times and resource usage.
Example: Using Yii’s Profiler
```php <?php // Add profiling in your application code Yii::beginProfile('myProfile'); // Your code here... Yii::endProfile('myProfile'); ```
Handling Exceptions and Errors
Effective error handling ensures that your application can gracefully handle unexpected issues. Yii provides mechanisms to manage exceptions and errors, ensuring a better user experience.
Example: Configuring Error Handling
```php <?php // config/web.php return [ // Other configurations... 'components' => [ 'errorHandler' => [ 'errorAction' => 'site/error', ], ], ]; ```
Debugging with Yii Console
The Yii console application can be used to execute commands, manage migrations, and perform other tasks, aiding in debugging and development.
Example: Using Yii Console
```bash php yii migrate php yii cache/flush-all ```
Conclusion
Utilizing Yii’s logging and debugging tools enhances your development workflow by providing comprehensive insights into your application’s performance and behavior. By configuring logging, leveraging the debug toolbar, monitoring performance, handling errors effectively, and utilizing console commands, you can streamline your development process and build more robust applications.
Further Reading:
Table of Contents
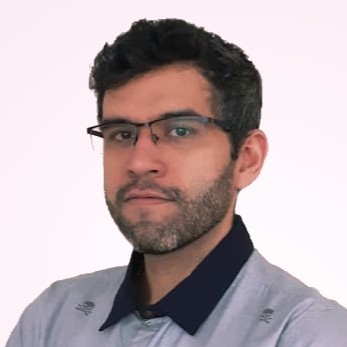
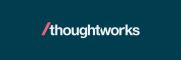