Yii Model-View-Controller (MVC) Explained: The Backbone of Yii
The Model-View-Controller (MVC) pattern is a crucial design principle in Yii, one of the most popular PHP frameworks for building web applications. Understanding MVC is essential for leveraging Yii’s full potential and creating organized, maintainable code. This article delves into the MVC architecture and its implementation in Yii.
Understanding MVC Architecture
MVC separates the application into three main components: Model, View, and Controller. Each component has distinct responsibilities, promoting a clean separation of concerns and facilitating easier maintenance and scalability.
- Model:
– Purpose: Represents the data and business logic of the application.
– Responsibilities: Interacts with the database, processes data, and defines business rules.
– Example in Yii: The `ActiveRecord` class, which provides an abstraction layer for database operations.
- View:
– Purpose: Displays data to the user and provides a user interface.
– Responsibilities: Renders the data provided by the model and handles user input.
– Example in Yii: Views are PHP files located in the `views` directory, responsible for generating HTML.
- Controller:
– Purpose: Acts as an intermediary between the Model and View.
– Responsibilities: Handles user input, manipulates data via the Model, and updates the View accordingly.
– Example in Yii: Controllers extend the `Controller` class and are responsible for defining actions and managing the flow of the application.
Setting Up Yii MVC
To illustrate the MVC pattern in Yii, let’s look at a simple example: creating a basic CRUD (Create, Read, Update, Delete) application.
- Defining the Model:
In Yii, models are often defined using the `ActiveRecord` class. Here’s how to create a simple model for a `Post` entity.
```php namespace app\models; use yii\db\ActiveRecord; class Post extends ActiveRecord { public static function tableName() { return 'post'; } public function rules() { return [ [['title', 'content'], 'required'], [['content'], 'string'], [['title'], 'string', 'max' => 255], ]; } } ```
- Creating the View:
Views in Yii are responsible for rendering the data. For the `Post` model, you might have views for creating, updating, and displaying posts.
Example: `views/post/index.php`
```php <?php use yii\helpers\Html; use yii\widgets\DetailView; /* @var $this yii\web\View */ /* @var $model app\models\Post */ ?> <h1><?= Html::encode($this->title) ?></h1> <p> <?= Html::a('Create Post', ['create'], ['class' => 'btn btn-success']) ?> </p> <?= DetailView::widget([ 'model' => $model, 'attributes' => [ 'id', 'title', 'content:ntext', ], ]) ?> ```
- Implementing the Controller:
Controllers handle user actions and invoke the appropriate model and view.
Example: `controllers/PostController.php`
```php namespace app\controllers; use Yii; use app\models\Post; use yii\web\Controller; use yii\web\NotFoundHttpException; use yii\filters\VerbFilter; class PostController extends Controller { public function actions() { return [ 'index' => 'app\controllers\actions\PostIndexAction', 'create' => 'app\controllers\actions\PostCreateAction', 'update' => 'app\controllers\actions\PostUpdateAction', 'delete' => 'app\controllers\actions\PostDeleteAction', ]; } } ```
Conclusion
The Model-View-Controller (MVC) architecture is fundamental to Yii, providing a robust structure for developing web applications. By separating the application into Models, Views, and Controllers, Yii promotes organized code and facilitates maintainable and scalable development.
Further Reading
Table of Contents
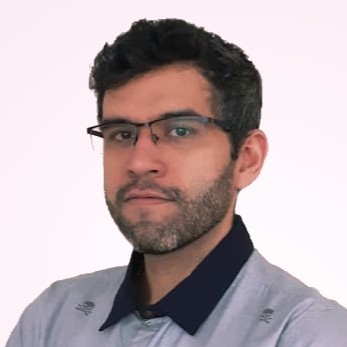
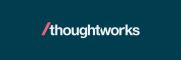