Yii Routing and URL Management: Creating SEO-Friendly URLs
Routing and URL management are critical aspects of web development that directly impact user experience and SEO performance. In Yii, a popular PHP framework, managing URLs effectively can help create user-friendly and SEO-optimized web applications. This article explores how to implement routing and URL management in Yii to create SEO-friendly URLs.
Setting Up Yii and Configuring URL Manager
To get started with URL management in Yii, you need to set up a basic Yii application and configure the URL manager to handle custom routes and create SEO-friendly URLs.
Example: Configuring URL Manager in Yii
First, you need to configure the `urlManager` component in the application configuration file (`config/web.php`).
```php 'components' => [ 'urlManager' => [ 'enablePrettyUrl' => true, 'showScriptName' => false, 'rules' => [ 'about' => 'site/about', 'contact' => 'site/contact', 'post/<id:\d+>' => 'post/view', 'posts' => 'post/index', ], ], ], ```
– `enablePrettyUrl`: Enables human-readable URLs.
– `showScriptName`: Hides the `index.php` in URLs.
– `rules`: Defines custom URL rules to map requests to controllers and actions.
Creating Custom Routes
Yii allows you to define custom routes to map specific URLs to controller actions, improving both SEO and usability. Custom routes can be easily created in the URL manager rules.
Example: Defining Custom Routes for SEO-Friendly URLs
```php 'rules' => [ 'about' => 'site/about', // Maps "/about" to "site/about" 'contact' => 'site/contact', // Maps "/contact" to "site/contact" 'post/<id:\d+>' => 'post/view', // Maps "/post/123" to "post/view?id=123" 'posts' => 'post/index', // Maps "/posts" to "post/index" 'category/<category:\w+>' => 'post/category', // Maps "/category/technology" to "post/category?category=technology" ], ```
This configuration allows you to create clean and descriptive URLs that are more appealing to both users and search engines.
Handling Dynamic URL Parameters
Yii provides a flexible way to handle dynamic parameters in URLs, such as user IDs or article slugs, using regular expressions. This approach is particularly useful for creating descriptive URLs that are easy to understand and index by search engines.
Example: Handling Dynamic Parameters in URLs
```php 'rules' => [ 'post/<id:\d+>' => 'post/view', // Matches numeric "id" parameter 'post/<slug:[\w-]+>' => 'post/view-by-slug', // Matches "slug" parameter containing letters, numbers, and dashes ], ```
Here, the `<id:\d+>` rule matches numeric IDs, while the `<slug:[\w-]+>` rule matches slugs that may contain letters, numbers, and hyphens.
Generating SEO-Friendly URLs in Yii
Yii provides a built-in helper, `Url`, to generate URLs dynamically within the application, ensuring consistency and flexibility in URL management.
Example: Generating URLs in Yii
```php use yii\helpers\Url; echo Url::to(['post/view', 'id' => 123]); // Generates "/post/123" echo Url::to(['post/category', 'category' => 'technology']); // Generates "/category/technology" ```
By using the `Url` helper, you can generate URLs dynamically based on the defined rules, ensuring they are SEO-friendly and consistent across your application.
Utilizing Slugs for SEO
Using slugs in URLs is a common SEO practice that involves creating human-readable strings instead of numeric IDs. Yii makes it easy to work with slugs in URLs to enhance SEO.
Example: Implementing Slugs in URLs
```php 'rules' => [ 'post/<slug:[\w-]+>' => 'post/view-by-slug', // Matches URL with slugs ], ```
When generating URLs or processing requests, you can use slugs to create more descriptive and readable URLs.
Redirecting Old URLs to New URLs
In some cases, you may need to redirect old URLs to new ones to maintain SEO value and provide a better user experience. Yii allows you to implement redirects easily using HTTP status codes.
Example: Redirecting Old URLs to New URLs
```php 'rules' => [ 'old-post/<id:\d+>' => 'post/redirect', // Redirect old URLs ], 'components' => [ 'request' => [ 'baseUrl' => '', ], ], ```
And in the `PostController`:
```php public function actionRedirect($id) { return $this->redirect(['post/view', 'id' => $id], 301); // 301 Permanent Redirect } ```
Handling 404 Errors and Custom Error Pages
To provide a better user experience, it’s important to handle 404 errors gracefully by displaying custom error pages. Yii allows you to define custom error actions in your application configuration.
Example: Custom Error Page Configuration
```php 'components' => [ 'errorHandler' => [ 'errorAction' => 'site/error', ], ], ```
In the `SiteController`, define the `actionError` method:
```php public function actionError() { $exception = Yii::$app->errorHandler->exception; if ($exception !== null) { return $this->render('error', ['exception' => $exception]); } } ```
Conclusion
Effective routing and URL management are essential for building SEO-friendly and user-friendly web applications. By leveraging Yii’s powerful routing capabilities, you can create clean, descriptive URLs that improve your website’s SEO and enhance user experience. With proper configuration and usage of custom routes, slugs, and error handling, you can optimize your Yii application for both users and search engines.
Further Reading:
Table of Contents
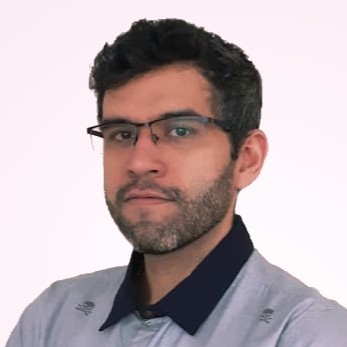
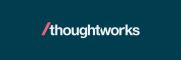