Yii Testing and Debugging: Ensuring Quality in Your Code
Testing and debugging are crucial steps in the development process to ensure code quality and maintainability. Yii, a high-performance PHP framework, offers a comprehensive set of tools and techniques to facilitate testing and debugging in your applications. This article explores how to implement effective testing and debugging strategies in Yii, ensuring your web applications are robust and reliable.
Setting Up Yii for Testing
To get started with testing in Yii, you need to set up your development environment and install the necessary testing tools. Yii supports PHPUnit for unit testing and Codeception for both functional and acceptance testing.
Example: Setting Up Yii Application for Testing
First, ensure you have PHPUnit and Codeception installed:
```bash composer require --dev phpunit/phpunit composer require --dev codeception/codeception ```
Next, initialize your Yii application with the testing environment:
```bash php yii init --env=Development ```
Unit Testing in Yii
Unit testing involves testing individual components or methods to ensure they work as expected. Yii integrates with PHPUnit, making it easy to write and run unit tests.
Example: Creating a Simple Unit Test
Create a test case file under the `tests/unit` directory. Here’s a basic example:
```php namespace tests\unit\models; use app\models\User; use PHPUnit\Framework\TestCase; class UserTest extends TestCase { public function testUserCreation() { $user = new User(); $user->username = 'testuser'; $this->assertTrue($user->validate(), 'User should be valid with correct attributes'); } } ```
Run the unit test with PHPUnit:
```bash vendor/bin/phpunit tests/unit/models/UserTest.php ```
Functional Testing in Yii
Functional testing ensures that the application behaves as expected by simulating user interactions. Yii leverages Codeception for functional tests.
Example: Writing a Functional Test
Create a functional test file under the `tests/functional` directory:
```php namespace tests\functional; use app\models\LoginForm; use tests\FunctionalTester; class LoginCest { public function ensureThatLoginWorks(FunctionalTester $I) { $I->amOnPage('/site/login'); $I->fillField('input[name="LoginForm[username]"]', 'admin'); $I->fillField('input[name="LoginForm[password]"]', 'admin'); $I->click('login-button'); $I->see('Logout'); } } ```
Run the functional test with Codeception:
```bash vendor/bin/codecept run functional LoginCest ```
Debugging Yii Applications
Yii provides powerful debugging tools through the Yii Debug Toolbar and Logger to identify and resolve issues in your application.
Enabling Yii Debug Toolbar
To enable the Yii Debug Toolbar, ensure the `debug` module is included in your configuration file:
```php 'bootstrap' => ['log', 'debug'], 'modules' => [ 'debug' => [ 'class' => 'yii\debug\Module', ], ], ```
The Debug Toolbar appears at the bottom of your application pages, providing insights into performance, SQL queries, and more.
Using Yii Logger for Debugging
Yii’s logging mechanism helps track down issues by recording messages at various levels (error, warning, info, trace). To configure logging, modify your `config/web.php` file:
```php 'components' => [ 'log' => [ 'traceLevel' => YII_DEBUG ? 3 : 0, 'targets' => [ [ 'class' => 'yii\log\FileTarget', 'levels' => ['error', 'warning'], ], ], ], ], ```
You can log custom messages using the `Yii::error()` or `Yii::info()` functions:
```php Yii::error('Something went wrong while processing user data.', __METHOD__); ```
Debugging with Breakpoints
For deeper inspection, you can use breakpoints with PHP’s built-in debugger (Xdebug). Configure Xdebug in your PHP environment and set breakpoints in your IDE to step through the code.
Conclusion
Testing and debugging are vital to maintaining code quality and reliability in Yii applications. By implementing unit and functional tests and utilizing Yii’s powerful debugging tools, you can ensure your applications are robust, scalable, and free from critical issues.
Further Reading:
Table of Contents
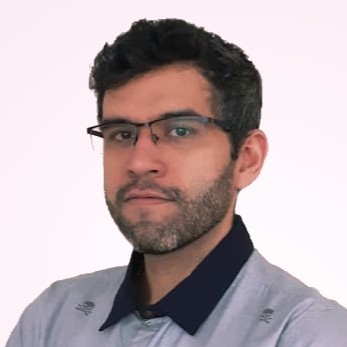
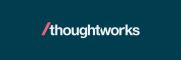