AI Development and Computer Vision: An In-depth Look
In today’s rapidly evolving technological landscape, the fields of AI development and computer vision have emerged as transformative forces with profound implications across industries. From automating routine tasks to enabling breakthroughs in medical diagnoses and autonomous vehicles, these technologies are redefining what’s possible. In this comprehensive exploration, we’ll delve into the core concepts, applications, and code samples that underpin AI development and computer vision.
1. Understanding AI Development
1.1. The Essence of Artificial Intelligence
Artificial Intelligence, or AI, refers to the creation of systems and machines that can simulate human intelligence processes. These processes include learning from experience, adapting to new situations, and performing tasks that typically require human intelligence. At the heart of AI development are algorithms and models that enable computers to recognize patterns, make predictions, and solve complex problems.
1.2. Machine Learning: Fueling AI Advancements
Machine learning is a subset of AI that involves training algorithms to learn from data and improve over time. Supervised learning, unsupervised learning, and reinforcement learning are common approaches. In supervised learning, algorithms learn from labeled examples, making predictions or decisions based on past data. Unsupervised learning involves finding patterns in unlabeled data, while reinforcement learning focuses on training models to make a sequence of decisions based on rewards and punishments.
1.3. Deep Learning: Unleashing the Power
Deep Learning, a subfield of machine learning, has garnered significant attention due to its ability to handle complex tasks with exceptional accuracy. Neural networks, inspired by the human brain’s structure, form the foundation of deep learning. Convolutional Neural Networks (CNNs) are particularly vital in computer vision, as they excel at image recognition tasks. Here’s an example of a simple CNN implementation in Python using TensorFlow:
python import tensorflow as tf from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense # Create a sequential model model = tf.keras.Sequential() # Add convolutional layers model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(256, 256, 3))) model.add(MaxPooling2D((2, 2))) model.add(Conv2D(64, (3, 3), activation='relu')) model.add(MaxPooling2D((2, 2))) model.add(Conv2D(128, (3, 3), activation='relu')) model.add(MaxPooling2D((2, 2))) # Flatten and add dense layers model.add(Flatten()) model.add(Dense(128, activation='relu')) model.add(Dense(10, activation='softmax')) # Compile the model model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
1.4. Natural Language Processing (NLP) in AI
Natural Language Processing (NLP) is a branch of AI that focuses on enabling computers to understand, interpret, and generate human language. NLP has found applications in sentiment analysis, language translation, chatbots, and more. Transformers, a breakthrough in NLP, have brought unprecedented improvements in language understanding and generation. The GPT-3 model is a prime example, capable of producing coherent and contextually relevant text.
2. Exploring Computer Vision
2.1. Unveiling Computer Vision
Computer vision empowers machines to interpret and understand visual information from the world. It involves processing and analyzing images and videos to extract meaningful insights. The applications range from facial recognition and object detection to medical image analysis and autonomous vehicles.
2.2. Key Techniques in Computer Vision
2.2.1. Image Classification:
Image classification involves assigning labels to images based on their content. This is a fundamental task in computer vision. Convolutional Neural Networks (CNNs) excel at image classification, as demonstrated earlier. These networks automatically learn features from images, enabling accurate classification.
2.2.2. Object Detection:
Object detection goes beyond classification by identifying and locating multiple objects within an image. It involves drawing bounding boxes around objects of interest. Faster R-CNN, YOLO (You Only Look Once), and SSD (Single Shot MultiBox Detector) are popular object detection algorithms.
2.2.3. Image Segmentation:
Image segmentation divides an image into segments, where each segment corresponds to a different object or region. This technique is crucial for tasks like autonomous driving, where understanding the precise boundaries of objects is essential.
2.3. Applying Computer Vision: Real-World Examples
2.3.1. Autonomous Vehicles:
Computer vision plays a pivotal role in enabling self-driving cars to navigate and make real-time decisions. By processing data from cameras, LiDAR, and other sensors, autonomous vehicles can detect lane boundaries, traffic signs, pedestrians, and other vehicles.
2.3.2. Healthcare:
In the medical field, computer vision aids in diagnosing diseases from medical images like X-rays, MRIs, and CT scans. It assists doctors by highlighting anomalies and potential areas of concern.
2.3.3. Augmented Reality (AR):
AR applications use computer vision to overlay digital information onto the physical world. From Snapchat filters to interactive museum exhibits, AR enhances user experiences by blending reality and digital elements.
3. Bridging AI and Computer Vision
The convergence of AI and computer vision has led to remarkable advancements that are reshaping industries. From enhancing the accuracy of medical diagnoses to revolutionizing how we interact with technology, this synergy has immense potential.
3.1. Building an AI-Powered Object Recognizer
Let’s explore a practical example that combines AI and computer vision: building an object recognizer using Python and OpenCV.
3.1.1. Install OpenCV:
Begin by installing the OpenCV library using the following command:
bash pip install opencv-python
3.1.2. Capture and Recognize Objects:
Use the following code to capture video from your webcam and apply object recognition using a pre-trained MobileNet SSD model.
python import cv2 # Load pre-trained MobileNet SSD model net = cv2.dnn.readNetFromCaffe('deploy.prototxt', 'mobilenet.caffemodel') # Open a connection to the webcam cap = cv2.VideoCapture(0) while True: ret, frame = cap.read() if not ret: break # Prepare the input image for object detection blob = cv2.dnn.blobFromImage(frame, 0.007843, (300, 300), 127.5) net.setInput(blob) # Perform object detection detections = net.forward() # Loop over the detections and draw bounding boxes for i in range(detections.shape[2]): confidence = detections[0, 0, i, 2] if confidence > 0.5: # Confidence threshold class_id = int(detections[0, 0, i, 1]) class_name = classNames[class_id] # Draw bounding box and label box = detections[0, 0, i, 3:7] * np.array([w, h, w, h]) x, y, x_end, y_end = box.astype('int') cv2.rectangle(frame, (x, y), (x_end, y_end), (0, 255, 0), 2) cv2.putText(frame, class_name, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2) # Display the output cv2.imshow('Object Recognizer', frame) if cv2.waitKey(1) & 0xFF == ord('q'): break cap.release() cv2.destroyAllWindows()
This code utilizes OpenCV’s Deep Neural Networks (dnn) module and the MobileNet SSD model for object detection. It captures video from your webcam, processes frames, and draws bounding boxes around recognized objects.
4. The Future of AI Development and Computer Vision
The journey of AI development and computer vision is only beginning. As technology advances, we can anticipate even more sophisticated algorithms, larger datasets, and enhanced hardware accelerating progress. From enabling robots to perceive and interact with the world around them to revolutionizing healthcare diagnostics, the future holds immense possibilities.
Conclusion
In conclusion, AI development and computer vision are driving innovations that transcend traditional boundaries. From understanding human language to making sense of the visual world, these technologies are reshaping industries and enhancing our daily lives. By understanding their core concepts, exploring their applications, and experimenting with code samples, we can all become part of this transformative journey into the future.
Table of Contents
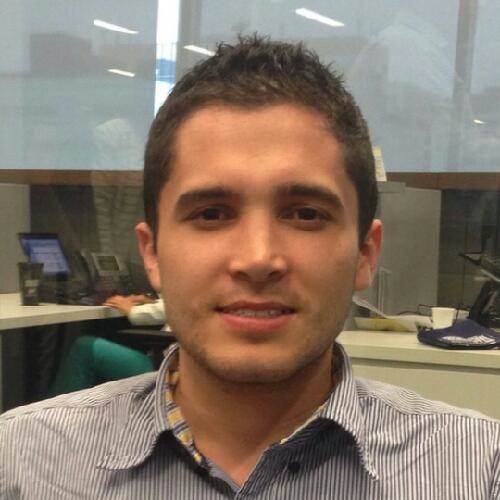
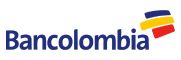