Revolutionize Your Android App: Techniques for Optimizing Performance & Efficiency
In the realm of mobile applications, providing an exceptional user experience is the pathway to success. This is where hiring expert Android developers becomes pivotal. They can create Android apps that are not only brimming with features but are also meticulously optimized for performance and efficiency. In this blog post, we’ll venture into various strategies and techniques that these skilled Android developers implement to elevate the performance and efficiency of your apps.
Table of Contents
1. Why is Optimization Important?
Before we jump into the specifics, it’s essential to understand why optimization is critical. Optimization can significantly enhance the user experience, leading to higher engagement rates, increased app usage, and better app store ratings. If an app is slow or consumes a lot of battery life, users may uninstall it and look for alternatives. Hence, developers must focus on improving the speed, responsiveness, and resource consumption of their applications.
Let’s explore the different areas where Android app optimization can be applied.
2. UI Rendering Performance
To ensure your app remains responsive and fluid, your UI should consistently render frames in under 16ms to achieve a smooth 60 frames per second. If your app spends more time rendering frames, it can cause noticeable stutters and lag, leading to a poor user experience.
Example – Overdraw Reduction
Overdraw occurs when the app draws the same pixel more than once within the same frame. Overdraw can be taxing on the device’s GPU and can lead to sluggish performance. To reduce overdraw:
* Use the “Debug GPU overdraw” tool in the Developer Options on your device. This tool color codes the screen to show you how many times each pixel is drawn. This can help you identify areas of excessive overdraw within your app.
* Use solid color backgrounds where possible. The more complex your view hierarchy, the more likely overdraw will occur.
* Use the `android:background` attribute sparingly. Every time you use this attribute, you add another layer that needs to be drawn.
* Clip views using `View.setClipToOutline()` when not in use.
3. Memory Management
Efficient memory management ensures your app maintains steady performance without becoming resource-intensive. If your app uses more memory than necessary, it can lead to garbage collection, which can cause your app to stutter and slow down.
Example – Object Pooling
Object pooling is a software creational design pattern that uses a set of initialized objects kept ready to use. Instead of creating and destroying objects repeatedly, the app reuses objects from the pool. This can greatly improve the app’s performance, especially when dealing with memory-intensive operations like graphics rendering.
Here is a basic implementation of object pooling:
```java public class ObjectPool { private List<MyObject> freeObjects; public ObjectPool() { this.freeObjects = new ArrayList<>(); } public MyObject getObject() { if (freeObjects.isEmpty()) { return new MyObject(); } else { return freeObjects.remove(freeObjects.size() - 1); } } public void returnObject(MyObject obj) { freeObjects.add(obj); } } ```
4. Battery Life Optimization
Battery life is one of the most critical resources on a mobile device. If your app drains the battery quickly, users may avoid using it. Thus, it’s essential to minimize your app’s battery usage.
Example – Efficient Background Services
Background services can be significant contributors to battery drain. They can be optimized by:
* Using JobScheduler for performing background tasks: The JobScheduler API allows Android to batch together tasks from various apps, reducing the overall amount of device wake-ups and therefore saving battery.
* Using WorkManager for deferrable and guaranteed tasks: WorkManager ensures tasks are run even if the app or device restarts and it’s optimized to respect system background restrictions and the device’s battery.
```java OneTimeWorkRequest myWorkRequest = new OneTimeWorkRequest.Builder(MyWorker.class) .setConstraints(myConstraints) .build(); WorkManager.getInstance(myContext).enqueue(myWorkRequest); ```
5. Network Usage Optimization
Excessive network usage can slow down your app, consume a lot of battery, and use up the user’s data. It’s essential to ensure your app uses the network wisely.
Example – Using OkHttp and Retrofit
OkHttp and Retrofit are powerful libraries for networking in Android. They offer features such as response caching, which saves previously retrieved responses and uses them when the network is not available or when the server revalidates them. This helps to reduce network calls and improve app performance.
```java OkHttpClient okHttpClient = new OkHttpClient.Builder() .cache(new Cache(new File(context.getCacheDir(), "http"), 10 * 1024 * 1024)) // 10 MB .build(); Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://myapi.com/") .client(okHttpClient) .addConverterFactory(GsonConverterFactory.create()) .build(); ```
Conclusion
Optimizing your Android application for performance and efficiency is a significant move that can greatly enhance the user experience. This is where the decision to hire Android developers becomes key, as this task requires constant monitoring, measuring, and improving. Android equips these professional developers with many potent tools like the Profiler in Android Studio and the Battery Historian. These tools aid developers in analyzing their apps’ performance and pinpointing areas ripe for optimization.
Remember, every millisecond saved, every byte of memory freed, and every percentage of battery conserved by your hired Android developers contributes to making your app smoother, faster, and more efficient. Here’s to successful optimization!
Table of Contents
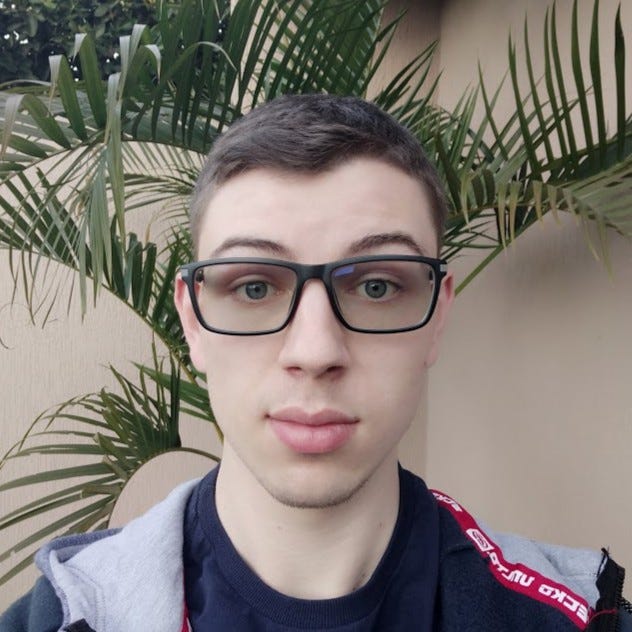
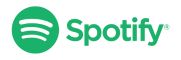