Geolocation in Android: Your Guide to Building GPS-Enabled Apps
The advent of smartphones has significantly transformed how we interact with technology, enabling functionalities that transcend mere communication. One critical feature is the capability to locate devices through GPS, which has inspired an array of location-based services (LBS) in Android apps. From ride-hailing apps like Uber and Lyft to social media apps like Instagram and Snapchat, LBS is central to their functionality. This heightened importance of LBS has led to a rise in demand to hire Android developers who specialize in building GPS-enabled apps. Let’s take a deep dive into how these skilled developers create GPS-enabled apps in Android, providing practical examples to guide us.
Table of Contents
1. The Basics of Location Services
LBS in Android leverages GPS, Network, and other location providers to determine a user’s location. The Android framework provides classes through the `android.location` package, such as `LocationManager` and `LocationListener`, enabling developers to access the device’s location services.
2. Permissions
Before you can access a user’s location, you must first request the necessary permissions. There are two types of location permissions:
- `ACCESS_FINE_LOCATION`: Allows access to precise location using GPS.
- `ACCESS_COARSE_LOCATION`: Allows access to an approximate location using sources like Wi-Fi and mobile networks.
In your AndroidManifest.xml, add:
```xml <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> ```
For apps targeting Android 6.0 (API level 23) and above, you must request location permissions at runtime. Here’s an example:
```java if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) { ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, PERMISSION_REQUEST_CODE); } ```
3. Accessing Location
To access location, you must create an instance of `LocationManager` and register a `LocationListener`. Here’s how to do it:
```java LocationManager locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE); LocationListener locationListener = new LocationListener() { public void onLocationChanged(Location location) { // Called when a new location is found by the network location provider. double lat = location.getLatitude(); double lng = location.getLongitude(); } public void onStatusChanged(String provider, int status, Bundle extras) {} public void onProviderEnabled(String provider) {} public void onProviderDisabled(String provider) {} }; // Register the listener with the Location Manager to receive location updates locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, locationListener); ```
4. Location Accuracy and Frequency
The frequency of location updates and their accuracy depend on the application requirements. For example, a weather app might not require high accuracy, while a navigation app requires frequent and highly accurate updates. The `requestLocationUpdates()` method has parameters to adjust the update frequency and distance interval.
5. Using Google Play Services for Location
The `android.location` package provides the basic tools for location services, but for more robust, accurate, and efficient location services, using Google Play Services’ `com.google.android.gms.location` package is recommended.
Here’s how to add it to your build.gradle:
```groovy dependencies { implementation 'com.google.android.gms:play-services-location:18.0.0' } ```
With Google Play Services, you can request the last known location of the user. It’s often enough for apps that need just a one-time location. Here’s how:
```java FusedLocationProviderClient fusedLocationClient = LocationServices.getFusedLocationProviderClient(this); fusedLocationClient.getLastLocation() .addOnSuccessListener(this, new OnSuccessListener<Location>() { @Override public void onSuccess(Location location) { if (location != null) { double lat = location.getLatitude(); double lng = location.getLongitude(); } } }); ```
6. Requesting Location Updates
For applications that need continuous location updates, you can request updates using `requestLocationUpdates()`. However, ensure the app does this efficiently to avoid draining the device battery.
```java LocationRequest locationRequest = LocationRequest.create(); locationRequest.setInterval(10000); locationRequest.setFastestInterval(5000); locationRequest.setPriority(LocationRequest.PRIORITY_HIGH_ACCURACY); LocationCallback locationCallback = new LocationCallback() { @Override public void onLocationResult(LocationResult locationResult) { if (locationResult == null) { return; } for (Location location : locationResult.getLocations()) { // Update UI with location data double lat = location.getLatitude(); double lng = location.getLongitude(); } } }; fusedLocationClient.requestLocationUpdates(locationRequest, locationCallback, null); ```
7. Geofencing
Geofencing allows your app to respond when a user enters or leaves a geographical region. It’s widely used in apps for triggering notifications, tracking, location-based marketing, and more.
```java Geofence geofence = new Geofence.Builder() .setRequestId("myGeofenceId") .setCircularRegion( lat, lng, 100 ) .setExpirationDuration(Geofence.NEVER_EXPIRE) .setTransitionTypes(Geofence.GEOFENCE_TRANSITION_ENTER | Geofence.GEOFENCE_TRANSITION_EXIT) .build(); GeofencingRequest geofencingRequest = new GeofencingRequest.Builder() .setInitialTrigger(GeofencingRequest.INITIAL_TRIGGER_ENTER) .addGeofence(geofence) .build(); PendingIntent geofencePendingIntent = ... // Create a PendingIntent geofencingClient.addGeofences(geofencingRequest, geofencePendingIntent) .addOnSuccessListener(this, new OnSuccessListener<Void>() { @Override public void onSuccess(Void aVoid) { // Geofences added } }); ```
Conclusion
Location-Based Services (LBS) are powerful tools that can greatly enhance your Android apps. To fully leverage this technology, you might consider seeking to hire Android developers proficient in LBS integration. However, remember to respect user privacy and only request location information when necessary, and always with user permission. Moreover, be sure to optimize for battery life when requesting location updates, a task that experienced Android developers are adept at. Happy coding!
Table of Contents
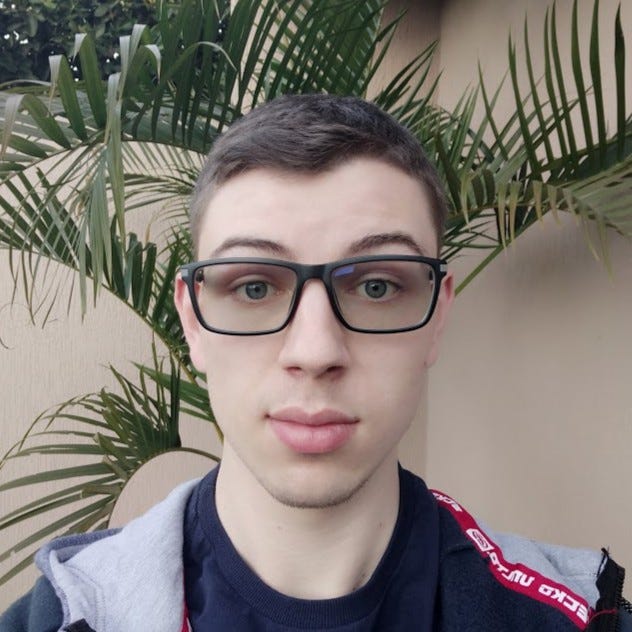
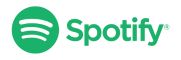