Exploring Angular Dependency Injection: Understanding Core Concepts
Angular is a popular and powerful front-end framework that simplifies the development of web applications. One of its key features is Dependency Injection (DI), a design pattern that plays a crucial role in building maintainable and scalable Angular applications. In this blog post, we will delve deep into the core concepts of Angular Dependency Injection, explore its benefits, and provide you with practical examples to help you grasp this fundamental concept.
Table of Contents
1. Introduction to Dependency Injection
1.1 What is Dependency Injection?
Dependency Injection (DI) is a software design pattern used to manage the dependencies between different components of an application. In the context of Angular, DI is a way to provide a class with the objects it depends on, rather than allowing it to create them itself. This pattern promotes the separation of concerns, making your code more modular, maintainable, and testable.
In Angular, components, services, and other classes can have dependencies on other classes. Dependency Injection helps resolve these dependencies by providing instances of the required dependencies at runtime. This eliminates the need for classes to create their dependencies, reducing tight coupling and making the code more flexible.
1.2 Why Use Dependency Injection in Angular?
Angular leverages Dependency Injection for several reasons:
- Modularity: DI encourages you to break your application into smaller, manageable pieces. Each piece can be developed, tested, and maintained independently, leading to a more modular architecture.
- Testability: With DI, it’s easier to replace real dependencies with mock objects during unit testing. This allows for thorough testing of individual components in isolation.
- Reusability: Components and services can be reused in different parts of your application or even in other projects because they are decoupled from their dependencies.
- Maintainability: By reducing tight coupling between components, DI makes your codebase more maintainable. You can easily swap out dependencies or update them without affecting other parts of the application.
Now that we understand the basics of Dependency Injection, let’s dive deeper into how it works in Angular.
2. How Dependency Injection Works in Angular
Angular’s Dependency Injection system consists of three main concepts: Providers, Injectors, and Tokens. Understanding these concepts is crucial for mastering DI in Angular.
2.1 Providers
Providers are a way of telling Angular how to create and deliver instances of a dependency. They can be services, values, or factories. Angular uses providers to know which dependencies a component or service requires and how to create them when needed.
Here’s an example of a simple provider in Angular:
typescript import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class MyService { // Service logic goes here }
In the above code, @Injectable() marks MyService as a provider. The providedIn property specifies that this service is available throughout the entire application.
2.2 Injectors
Injectors are responsible for creating and managing instances of dependencies. Angular’s DI system has a hierarchical injector tree, where each component has its own injector. When a component or service requests a dependency, Angular looks up the injector tree to find the nearest injector that can provide the requested dependency.
Here’s an example of injecting a service into a component:
typescript import { Component } from '@angular/core'; import { MyService } from './my-service'; @Component({ selector: 'app-my-component', template: '<p>{{ message }}</p>', }) export class MyComponent { message: string; constructor(private myService: MyService) { this.message = this.myService.getMessage(); } }
In this example, MyComponent injects MyService through its constructor. Angular’s injector system resolves this dependency by creating an instance of MyService and passing it to the component.
2.3 Tokens
Tokens are unique identifiers that Angular uses to map dependencies to providers. When you inject a dependency into a component or service, you use a token to specify which dependency you want.
Angular provides several types of tokens:
- Class tokens: These tokens use the class itself as the identifier. For example, when you inject a service into a component, Angular uses the service class as the token.
- Opaque tokens: These tokens use an opaque value (typically a string or symbol) as the identifier. Opaque tokens are useful when you need to inject multiple instances of the same class with different configurations.
Now that we’ve covered the basic concepts of Dependency Injection, let’s explore the benefits of using this pattern in your Angular applications.
3. The Benefits of Using Dependency Injection
3.1 Maintainability
Maintainability is a critical aspect of software development, and Dependency Injection greatly contributes to it. By injecting dependencies into components and services, you reduce the coupling between them. This means that if you need to change or update a dependency, you can do so without affecting the entire application. Each component remains isolated, making it easier to manage and maintain.
3.2 Testability
Testing is an essential part of software development, and Angular’s Dependency Injection system makes it easier to write unit tests. Since components and services depend on abstractions (interfaces or classes) rather than concrete implementations, you can easily replace real dependencies with mock objects for testing. This allows you to write isolated unit tests that verify the behavior of individual components.
3.3 Reusability
Dependency Injection promotes the creation of reusable components and services. When you design your classes to depend on abstractions, they become more versatile and can be used in different parts of your application or even in other projects. This reusability saves development time and promotes a consistent user experience across your applications.
4. Practical Examples
Now that we’ve explored the theory behind Dependency Injection, let’s dive into some practical examples to illustrate how it works in Angular.
4.1 Creating a Simple Service
In Angular, services are often used as dependencies for components. Let’s create a simple service and inject it into a component.
First, create a service using the Angular CLI:
bash ng generate service my-service
This command generates a service file (my-service.service.ts) with the following content:
typescript import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class MyService { constructor() { } getMessage(): string { return 'Hello from MyService!'; } }
The @Injectable decorator with providedIn: ‘root’ ensures that this service is available as a singleton throughout the application.
Now, let’s inject this service into a component:
typescript import { Component } from '@angular/core'; import { MyService } from './my-service.service'; @Component({ selector: 'app-my-component', template: '<p>{{ message }}</p>', }) export class MyComponent { message: string; constructor(private myService: MyService) { this.message = this.myService.getMessage(); } }
In this example, MyComponent injects MyService through its constructor, allowing it to access the service’s getMessage() method.
4.2 Injecting Services into Components
Angular’s Dependency Injection system makes it easy to inject services into components, as demonstrated in the previous example. This approach decouples your components from their dependencies, making your code more maintainable and testable.
4.3 Hierarchical Injectors
Angular’s injector system is hierarchical. Each component has its own injector, and when you request a dependency, Angular looks up the injector tree to find the nearest provider for that dependency. This hierarchical structure allows for fine-grained control over where dependencies are provided and accessed.
For example, you can provide a service at the component level:
typescript @Component({ selector: 'app-my-component', template: '<p>{{ message }}</p>', providers: [MyService], }) export class MyComponent { message: string; constructor(private myService: MyService) { this.message = this.myService.getMessage(); } }
In this case, MyComponent has its own instance of MyService, separate from other components in the application.
5. Best Practices for Dependency Injection in Angular
While Angular’s Dependency Injection system is powerful, it’s essential to follow best practices to ensure your application remains maintainable and efficient.
5.1 Use providedIn
Angular introduced the providedIn property in Angular 6. It’s recommended to use this property to specify the scope of service providers. Using providedIn: ‘root’ makes the service a singleton available throughout the application, which is often the desired behavior. However, you can also specify providers at the component level when needed, as shown earlier.
5.2 Avoid Using the ReflectiveInjector
Angular’s ReflectiveInjector should be used sparingly and only when you have specific use cases that require manual control over the injection process. In most cases, Angular’s built-in DI system and the providedIn property are sufficient.
5.3 Lazy Loading Modules
When using lazy-loaded modules, be aware that each module has its own injector. Ensure that you provide services at the module level to avoid multiple instances of the same service in different parts of your application.
Conclusion
In this blog post, we’ve explored the core concepts of Angular Dependency Injection, delving into providers, injectors, tokens, and the benefits of using this powerful design pattern in your Angular applications. By embracing Dependency Injection, you can create modular, maintainable, and testable code that promotes reusability and enhances the overall quality of your Angular projects. Remember to follow best practices to make the most of Angular’s Dependency Injection system and build robust web applications.
Table of Contents
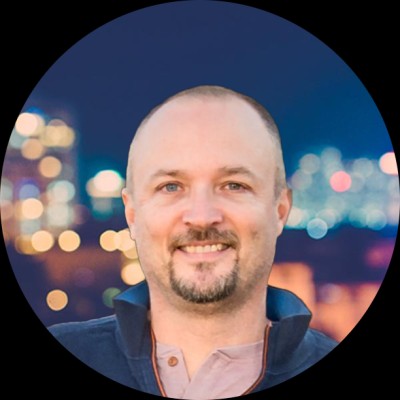
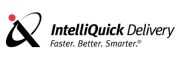