Creating Custom Exceptions in C#: Handling Errors Your Way
In any programming language, error handling is an essential part of building robust and reliable applications. C# provides a powerful exception handling mechanism that allows developers to catch and handle errors gracefully. While C# offers a range of built-in exceptions, sometimes you may encounter situations where none of the existing exceptions fit your specific needs. In such cases, creating custom exceptions can be a valuable technique to handle errors in a way that aligns with your application’s requirements. This blog post will guide you through the process of creating custom exceptions in C#, showcasing how you can take control of error handling in your applications.
Understanding Exceptions in C#
Exceptions in C# are objects that represent exceptional conditions or errors that occur during program execution. They allow you to handle errors in a structured and controlled manner, preventing abrupt termination of the application. C# includes a wide range of built-in exception classes, such as ArgumentException, NullReferenceException, and InvalidOperationException, to handle common error scenarios. However, there are cases where these built-in exceptions may not provide enough context or specificity for your application’s error handling needs.
The Need for Custom Exceptions
Creating custom exceptions allows you to tailor error handling to your application’s specific requirements. By defining your own exception classes, you can add custom properties, methods, and behaviors to enhance the error handling process. Custom exceptions also provide a way to encapsulate domain-specific information about the error, making it easier to communicate and diagnose issues within your application.
Creating Custom Exceptions in C#
1. Inheriting from the Exception Class:
To create a custom exception in C#, you need to define a new class that inherits from the base Exception class. This inheritance allows your custom exception to inherit all the standard exception behaviors and properties. For example, let’s create a custom exception called CustomException:
csharp public class CustomException : Exception { // Custom exception code }
2. Adding Custom Properties and Methods:
One of the advantages of creating custom exceptions is the ability to add custom properties and methods. These additional members can provide more information about the error or perform specific actions during exception handling. For instance, let’s add a custom property called ErrorCode to our CustomException class:
csharp public class CustomException : Exception { public string ErrorCode { get; } public CustomException(string message, string errorCode) : base(message) { ErrorCode = errorCode; } }
3. Overriding Existing Exception Methods:
In addition to adding custom properties and methods, you can also override existing methods from the base Exception class. By doing so, you can customize the behavior of your custom exception. For example, let’s override the ToString() method to include the ErrorCode property in the exception’s string representation:
csharp public class CustomException : Exception { public string ErrorCode { get; } public CustomException(string message, string errorCode) : base(message) { ErrorCode = errorCode; } public override string ToString() { return $"{base.ToString()} Error Code: {ErrorCode}"; } }
Throwing and Catching Custom Exceptions
Once you have defined your custom exception, you can throw it using the throw statement wherever necessary in your code. To catch and handle the custom exception, you can use try-catch blocks. For example:
try { // Code that may throw a custom exception } catch (CustomException ex) { // Handle the custom exception }
Practical Example: Creating a Custom FileProcessingException
Let’s explore a practical example of creating a custom exception for handling file processing errors.
1. Defining the Custom Exception:
csharp public class FileProcessingException : Exception { public string FileName { get; } public FileProcessingException(string fileName, string message) : base(message) { FileName = fileName; } public override string ToString() { return $"File Processing Exception: {FileName} - {Message}"; } }
2. Throwing and Catching the Custom Exception:
Suppose you have a file processing method that reads a file and performs some operations. If an error occurs during the file processing, you can throw the FileProcessingException:
csharp public void ProcessFile(string fileName) { try { // File processing logic } catch (Exception ex) { throw new FileProcessingException(fileName, "Error processing file.", ex); } }
Best Practices for Using Custom Exceptions
- Use custom exceptions judiciously and only when necessary to avoid unnecessary complexity.
- Ensure that your custom exceptions provide clear and meaningful information about the error.
- Consider creating a hierarchy of custom exceptions to handle different error scenarios in a more granular way.
- Document the usage of your custom exceptions and provide examples to facilitate their proper handling by other developers.
Conclusion
Creating custom exceptions in C# empowers you to handle errors in a way that aligns with your application’s needs. By defining your own exception classes, adding custom properties and methods, and overriding existing exception methods, you can tailor the error handling process to provide more context and specificity. Custom exceptions offer a powerful mechanism for capturing domain-specific error information, improving the diagnostic process within your application. Use custom exceptions judiciously and follow best practices to ensure your code remains maintainable and resilient when it comes to error handling.
Table of Contents
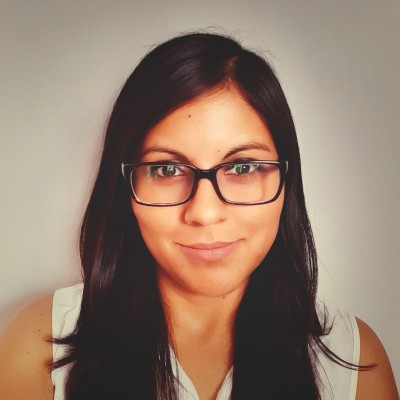
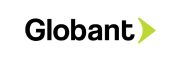