C# Design Patterns: Enhancing Code Reusability
In the world of software development, code reusability is a vital aspect that can significantly impact the efficiency and maintainability of projects. One of the most effective ways to achieve code reusability is through the implementation of design patterns. Design patterns provide proven techniques and best practices for solving common programming problems, making code more modular, flexible, and easier to understand. In this blog, we will explore various C# design patterns and demonstrate how they can enhance code reusability, leading to more efficient and maintainable software development.
The Importance of Code Reusability
Code reusability plays a crucial role in software development. When we write reusable code, we can avoid duplication, save development time, and improve overall code quality. By employing design patterns, developers can create solutions that are easily adaptable, maintainable, and extensible. This not only enhances code reusability but also leads to more efficient and scalable software systems.
Introduction to Design Patterns
Design patterns are reusable solutions to commonly occurring problems in software design. They provide a blueprint for constructing well-structured and modular code. There are three main categories of design patterns: creational, structural, and behavioral.
Creational Design Patterns
Creational design patterns focus on object creation mechanisms. They provide ways to instantiate objects while hiding the creation logic, making code more flexible and decoupled. Two popular creational design patterns in C# are the Singleton and Factory patterns.
1. Singleton
The Singleton pattern ensures that only one instance of a class is created throughout the application. This is useful when we need to restrict object creation and maintain a single point of access to the instance. Here’s an example of implementing the Singleton pattern in C#:
csharp public class Singleton { private static Singleton instance; private Singleton() { } public static Singleton Instance { get { if (instance == null) instance = new Singleton(); return instance; } } }
2. Factory
The Factory pattern provides an interface for creating objects, but allows subclasses to decide which class to instantiate. It promotes loose coupling by abstracting the object creation process. Here’s an example of the Factory pattern in C#:
csharp public abstract class Animal { public abstract void MakeSound(); } public class Dog : Animal { public override void MakeSound() { Console.WriteLine("Woof!"); } } public class Cat : Animal { public override void MakeSound() { Console.WriteLine("Meow!"); } } public class AnimalFactory { public Animal CreateAnimal(string type) { switch (type) { case "Dog": return new Dog(); case "Cat": return new Cat(); default: throw new ArgumentException("Invalid animal type."); } } }
Structural Design Patterns
Structural design patterns deal with the composition of classes and objects, enabling them to form larger structures. They focus on simplifying the relationships between objects and providing flexibility in the way they communicate. Two commonly used structural design patterns in C# are the Adapter and Composite patterns.
1. Adapter
The Adapter pattern allows incompatible interfaces to work together by acting as a bridge between them. It converts the interface of a class into another interface expected by clients. This enables objects with different interfaces to collaborate without modifying their original code. Here’s an example of the Adapter pattern in C#:
csharp public interface ITarget { void Request(); } public class Adaptee { public void SpecificRequest() { Console.WriteLine("Adaptee's specific request"); } } public class Adapter : ITarget { private readonly Adaptee adaptee; public Adapter(Adaptee adaptee) { this.adaptee = adaptee; } public void Request() { adaptee.SpecificRequest(); } }
2. Composite
The Composite pattern allows you to treat individual objects and groups of objects uniformly. It composes objects into tree structures, making it easier to work with hierarchical representations. Here’s an example of the Composite pattern in C#:
csharp public interface IComponent { void Display(); } public class Leaf : IComponent { private readonly string name; public Leaf(string name) { this.name = name; } public void Display() { Console.WriteLine($"Leaf: {name}"); } } public class Composite : IComponent { private readonly List<IComponent> children = new List<IComponent>(); public void Add(IComponent component) { children.Add(component); } public void Display() { foreach (var component in children) component.Display(); } }
Behavioral Design Patterns
Behavioral design patterns focus on communication between objects and the distribution of responsibilities. They define how objects interact with each other and help to provide flexibility in communication. Two widely used behavioral design patterns in C# are the Observer and Strategy patterns.
1. Observer
The Observer pattern establishes a one-to-many dependency between objects. When one object changes its state, all dependent objects are notified and updated automatically. This promotes loose coupling between objects and enables them to interact without knowing each other’s concrete implementations. Here’s an example of the Observer pattern in C#:
csharp public interface IObserver { void Update(); } public class ConcreteObserver : IObserver { public void Update() { Console.WriteLine("ConcreteObserver has been updated."); } } public class Subject { private readonly List<IObserver> observers = new List<IObserver>(); public void Attach(IObserver observer) { observers.Add(observer); } public void Notify() { foreach (var observer in observers) observer.Update(); } }
2. Strategy
The Strategy pattern allows you to define a family of algorithms and make them interchangeable at runtime. It encapsulates different algorithms, making them independent of the client code that uses them. This provides flexibility and enables dynamic selection of algorithms. Here’s an example of the Strategy pattern in C#:
csharp public interface IStrategy { void Execute(); } public class ConcreteStrategyA : IStrategy { public void Execute() { Console.WriteLine("ConcreteStrategyA executed."); } } public class ConcreteStrategyB : IStrategy { public void Execute() { Console.WriteLine("ConcreteStrategyB executed."); } } public class Context { private readonly IStrategy strategy; public Context(IStrategy strategy) { this.strategy = strategy; } public void ExecuteStrategy() { strategy.Execute(); } }
Conclusion
C# design patterns provide powerful tools for enhancing code reusability. By leveraging proven techniques and best practices, developers can create more efficient and maintainable software systems. Creational, structural, and behavioral design patterns enable modular and flexible code, making it easier to adapt, maintain, and extend. By incorporating these design patterns into your development process, you can significantly enhance code reusability, leading to more efficient and scalable software solutions.
In this blog, we explored several popular design patterns in C#, including the Singleton, Factory, Adapter, Composite, Observer, and Strategy patterns. Each pattern addresses specific aspects of software design and helps achieve code reusability. By understanding and applying these design patterns, you can elevate your programming skills and build more robust and maintainable software systems.
Table of Contents
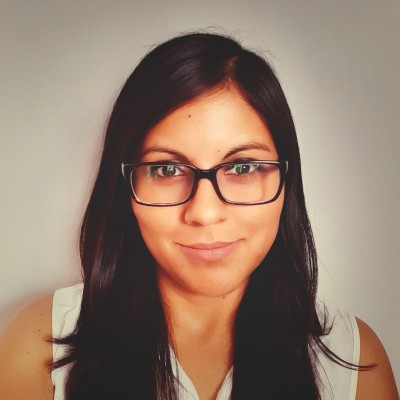
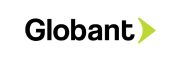