Implementing CakePHP Behaviors: Adding Functionality to Models
CakePHP is a popular PHP framework known for its simplicity and ease of use. One of its key features is the ability to extend and enhance models using behaviors. Behaviors allow you to encapsulate reusable functionality and attach it to your models, reducing code duplication and making your development process more efficient. In this blog post, we will explore how to implement CakePHP behaviors and leverage their power to add functionality to your models.
1. Understanding CakePHP Behaviors
1.1 What are Behaviors?
In CakePHP, behaviors are classes that can be attached to models, allowing you to enhance the functionality of those models. Behaviors encapsulate reusable logic, such as data validation, data manipulation, or even custom business rules. By attaching a behavior to a model, you can easily extend the model’s capabilities without modifying the core code.
1.2 Benefits of Using Behaviors
Using behaviors in your CakePHP application offers several advantages. First, it promotes code reusability and maintainability. By encapsulating common functionality in behaviors, you avoid code duplication and ensure consistent behavior across different models. Second, behaviors allow you to keep your models clean and focused on their core responsibilities, improving code organization. Finally, behaviors make it easier to test your models and write unit tests for specific functionality without the need for complex setups.
1.3 When to Use Behaviors
Behaviors are particularly useful in scenarios where you need to add common functionality across multiple models. For example, if you want to add timestamp fields to your models to track creation and modification dates, you can create a behavior that automatically handles these fields. This way, you can attach the behavior to any model that requires such functionality without repeating code.
2. Creating a Custom Behavior
2.1 Defining a Behavior Class
To create a custom behavior in CakePHP, you need to define a behavior class that extends the Cake\ORM\Behavior class. This base class provides the necessary methods and hooks to integrate your behavior with the model’s lifecycle. Here’s an example of a behavior class that adds soft deletion functionality to a model:
php namespace App\Model\Behavior; use Cake\ORM\Behavior; use Cake\Event\Event; use Cake\ORM\Query; use Cake\ORM\Table; class SoftDeleteBehavior extends Behavior { public function beforeFind(Event $event, Query $query, \ArrayObject $options, bool $primary) { $query->andWhere([$this->_table->getAlias() . '.deleted' => false]); } public function delete(Table $table, $entity) { $entity->deleted = true; return $table->save($entity); } }
2.2 Implementing Behavior Methods
In the example above, the beforeFind method is implemented to modify the query conditions and exclude soft-deleted records. The delete method overrides the default delete behavior, setting the deleted flag to true and saving the entity.
2.3 Attaching a Behavior to a Model
To attach a behavior to a model, you need to add it to the model’s initialize method. For example, to attach the SoftDeleteBehavior to a UsersTable model, you would do the following:
php namespace App\Model\Table; use Cake\ORM\Table; use App\Model\Behavior\SoftDeleteBehavior; class UsersTable extends Table { public function initialize(array $config) { $this->addBehavior('SoftDelete'); } }
3. Built-in CakePHP Behaviors
CakePHP comes with several built-in behaviors that provide common functionality out of the box. Let’s explore a few examples:
3.1 Timestamp Behavior
The Timestamp behavior automatically sets and updates timestamp fields, such as created and modified. To use this behavior, simply attach it to your model:
php $this->addBehavior('Timestamp');
3.2 SoftDelete Behavior
The SoftDelete behavior allows you to perform soft deletions by setting a flag instead of physically deleting records. Attaching this behavior to a model enables the soft deletion functionality:
php $this->addBehavior('SoftDelete');
3.3 Sluggable Behavior
The Sluggable behavior automatically generates slugs for specified fields in your model, making them URL-friendly. Attaching this behavior to a model allows you to generate slugs effortlessly:
php $this->addBehavior('Sluggable', ['field' => 'title']);
4. Working with Behaviors
4.1 Configuring Behavior Options
Behaviors often provide configurable options that allow you to customize their behavior. When attaching a behavior, you can pass an array of options as the second argument:
php $this->addBehavior('Sluggable', ['field' => 'title', 'slugField' => 'slug']);
4.2 Modifying Behavior Methods
You can override or extend behavior methods by re-implementing them in your model class. For example, if you want to modify the behavior’s beforeFind method, you can do the following:
php public function beforeFind(Event $event, Query $query, \ArrayObject $options, bool $primary) { // Custom logic here // Call parent method to preserve the original behavior parent::beforeFind($event, $query, $options, $primary); }
4.3 Attaching Multiple Behaviors
You can attach multiple behaviors to a single model by calling the addBehavior method multiple times:
php $this->addBehavior('Timestamp'); $this->addBehavior('Sluggable', ['field' => 'title']);
Conclusion
CakePHP behaviors offer a powerful way to extend the functionality of your models and make your development process more efficient. By encapsulating reusable logic in behaviors, you can achieve code reusability, maintainability, and cleaner models. Additionally, the built-in behaviors provided by CakePHP save you time and effort by offering common functionality out of the box. Start leveraging the power of behaviors in your CakePHP applications to enhance your models and streamline your development workflow.
Table of Contents
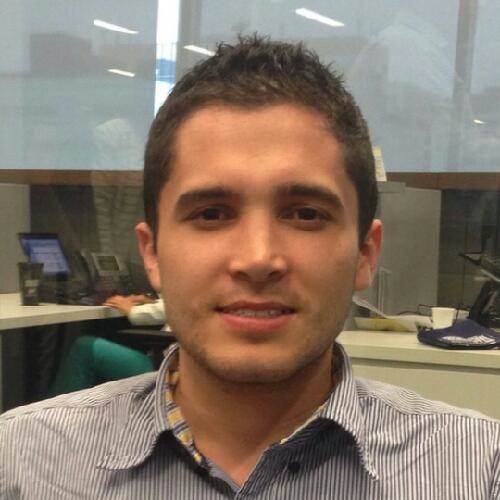
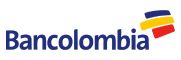