CakePHP and Internet of Things (IoT): Connecting Devices
The Internet of Things (IoT) has rapidly transformed the way we interact with everyday objects and devices. From smart thermostats that adjust the temperature based on our preferences to wearable fitness trackers that monitor our health, IoT has woven a web of interconnected devices that enhance our lives. Behind the scenes, robust software frameworks play a crucial role in making these devices work seamlessly. One such framework is CakePHP, a popular PHP framework known for its simplicity and flexibility. In this blog post, we’ll delve into the exciting world of CakePHP and IoT, exploring how CakePHP can connect and control IoT devices.
Table of Contents
1. Understanding the Internet of Things (IoT)
1.1. What is IoT?
Before we dive into the technical aspects of CakePHP and IoT integration, let’s establish a solid understanding of what the Internet of Things is. IoT refers to the interconnectedness of everyday objects and devices through the internet. These objects, often embedded with sensors and communication hardware, can collect and exchange data, making them “smart” in the process.
IoT has a broad range of applications, from smart homes and cities to industrial automation and healthcare. Some common examples include smart thermostats that adjust temperature based on user preferences, connected vehicles that monitor engine health, and wearable devices that track fitness metrics.
1.2. The IoT Ecosystem
The IoT ecosystem comprises three essential components:
- Devices: These are the physical objects or sensors that collect data. Devices can range from simple temperature sensors to complex industrial machinery equipped with multiple sensors.
- Connectivity: Connectivity ensures that devices can communicate with each other and transmit data to the cloud or other endpoints. This is often achieved using Wi-Fi, cellular networks, Bluetooth, or even low-power, wide-area networks (LPWAN).
- Cloud and Applications: The cloud serves as the central hub where data from IoT devices is collected, stored, and processed. IoT applications, often web or mobile-based, enable users to interact with and control these devices remotely.
Now that we have a foundational understanding of IoT, let’s explore how CakePHP can be leveraged to connect and control these devices.
2. CakePHP: A Brief Overview
2.1. What is CakePHP?
CakePHP is an open-source, rapid development framework for PHP. It follows the Model-View-Controller (MVC) architectural pattern and provides a set of conventions for rapid application development. CakePHP is known for its:
- Ease of use: It simplifies complex tasks and reduces the amount of code you need to write.
- Security: It includes built-in security features to protect your web applications from common vulnerabilities.
- Database access: CakePHP offers a powerful ORM (Object-Relational Mapping) system that simplifies database interactions.
- Extensibility: You can extend CakePHP with plugins and components to add custom functionality.
- Community support: A large and active community provides resources, plugins, and documentation.
3. CakePHP and IoT: The Synergy
Now that we’re acquainted with CakePHP and IoT, let’s explore how these two technologies can work together harmoniously.
3.1. Device Communication
One of the key aspects of IoT is the ability of devices to communicate and share data. CakePHP can facilitate this communication by serving as an intermediary between IoT devices and the cloud. Here’s a simplified example of how this can be achieved using CakePHP and MQTT, a lightweight messaging protocol commonly used in IoT:
php // Include the MQTT library require_once('mqtt.php'); // Create an MQTT client instance $mqtt = new phpMQTT('example.com', 1883, 'ClientId'); // Connect to the MQTT broker if ($mqtt->connect()) { // Subscribe to a topic $topics = array('iot/data' => array('qos' => 0, 'function' => 'processMessage')); $mqtt->subscribe($topics, 0); // Keep the connection alive while ($mqtt->proc()) { } // Disconnect from the MQTT broker $mqtt->close(); } // Callback function to process incoming messages function processMessage($topic, $message) { // Process the message received from the IoT device // You can use CakePHP models and controllers here to handle the data }
In this example, CakePHP sets up an MQTT client to subscribe to a specific topic where IoT devices publish data. When a message is received, CakePHP’s callback function processes the data using CakePHP’s built-in features, such as models and controllers.
3.2. Data Storage and Analysis
IoT generates massive amounts of data, and CakePHP’s ORM system can efficiently handle data storage and retrieval. You can create models that represent IoT data and use CakePHP’s querying capabilities to analyze and present this data to users.
php // CakePHP model for IoT data class IoTData extends AppModel { public $name = 'IoTData'; // Define fields and validation rules here }
With CakePHP’s ORM, you can easily create, update, and retrieve IoT data in your application’s database. This data can then be used to generate reports, charts, and dashboards for monitoring and analysis.
3.3. Remote Device Control
One of the most compelling aspects of IoT is the ability to control devices remotely. CakePHP can facilitate this by providing a web-based interface through which users can interact with and control IoT devices. Here’s a simplified example of how to create a web page to control a smart light bulb:
php // CakePHP controller action for controlling a smart light bulb public function controlLightBulb($deviceId, $action) { // Logic to send commands to the IoT device // This can involve sending MQTT messages or making API requests // You can use CakePHP's HTTP client for API requests // Update the device status in the database // Return a response to the user }
In this example, CakePHP’s controller action receives user input to control a smart light bulb. It then communicates with the IoT device, either through MQTT or API requests, to execute the desired action.
3.4. Security Considerations
Security is paramount when dealing with IoT devices, as they can be vulnerable to various attacks. CakePHP’s built-in security features can help mitigate these risks. Here are some security considerations when integrating CakePHP with IoT:
- Authentication: Implement user authentication to ensure that only authorized users can control IoT devices.
- Authorization: Define roles and permissions to restrict access to specific devices or actions.
- Data Encryption: Use encryption protocols to secure data transmission between CakePHP and IoT devices.
- API Security: If you expose APIs for IoT device communication, implement API keys or OAuth for authentication.
- Firmware Updates: Ensure that IoT devices receive regular firmware updates to patch vulnerabilities.
4. Case Study: Smart Home Automation with CakePHP and IoT
To illustrate the real-world application of CakePHP and IoT integration, let’s explore a case study: Smart Home Automation.
4.1. Scenario
Imagine you want to build a smart home automation system that allows you to control lights, thermostats, and security cameras in your home remotely. Here’s how CakePHP can help you achieve this:
- Device Integration: You connect smart bulbs, thermostats, and cameras to your home Wi-Fi network. These devices can communicate with CakePHP through MQTT.
- Data Management: CakePHP stores data from these devices, such as temperature readings, camera feeds, and light status, in a database using its ORM.
- User Interface: You create a web-based dashboard using CakePHP’s views and controllers. This dashboard allows you to view the camera feeds, adjust the thermostat, and control the lights.
- Security: You implement user authentication to ensure that only authorized users can access and control your smart home devices.
- Remote Control: Using your smartphone or computer, you can log in to the CakePHP-powered dashboard from anywhere and control your smart home devices.
- Automation: You can set up automation rules in CakePHP to, for example, turn on the lights when motion is detected or adjust the thermostat based on your schedule.
This case study showcases how CakePHP can be a powerful tool for building IoT applications, offering seamless device integration, data management, and user interaction.
5. Getting Started with CakePHP and IoT
Now that we’ve explored the potential synergy between CakePHP and IoT, let’s get started with a basic setup.
5.1. Prerequisites
Before you begin, make sure you have the following:
- A web server with PHP and CakePHP installed.
- IoT devices or simulators that can communicate using MQTT or other protocols.
- Access to a database for storing IoT data (you can use CakePHP’s built-in support for databases).
5.2. Steps
- Install CakePHP: If you haven’t already, install CakePHP on your web server. You can follow the official installation guide for detailed instructions.
- Create a CakePHP Project: Use CakePHP’s command-line tools to create a new project. Navigate to your web server’s directory and run:
bash composer create-project --prefer-dist cakephp/app my-iot-project
Replace my-iot-project with your desired project name.
- Set Up a Database: Configure CakePHP to connect to your database. Open config/app.php and update the Datasources section with your database details.
- Create Models: Create CakePHP models to represent your IoT data. For example:
php // In src/Model/Table/IoTData.php namespace App\Model\Table; use Cake\ORM\Table; class IoTDataTable extends Table { public function initialize(array $config): void { $this->addBehavior('Timestamp'); } }
- Device Communication: Implement code to communicate with your IoT devices. Use CakePHP’s controllers and MQTT or other protocols to send and receive data.
- User Interface: Create views and controllers to build a user interface for interacting with IoT devices.
- Security: Implement security measures, including user authentication and data encryption, to protect your IoT application.
- Testing: Thoroughly test your CakePHP and IoT integration to ensure it works as expected.
- Deployment: Deploy your CakePHP-powered IoT application to your web server.
Conclusion
CakePHP and IoT can be a powerful combination for building IoT applications that connect and control devices seamlessly. CakePHP’s simplicity, security features, and database support make it a versatile framework for handling IoT data and device communication.
As you embark on your journey to connect devices using CakePHP, remember to prioritize security, scalability, and user experience. With the right approach, you can create innovative IoT applications that improve efficiency, convenience, and quality of life.
So, whether you’re building a smart home automation system or an industrial IoT solution, CakePHP can be your trusted ally in connecting the dots of the Internet of Things.
Explore the endless possibilities of CakePHP and IoT, and turn your ideas into reality! Happy coding!
Table of Contents
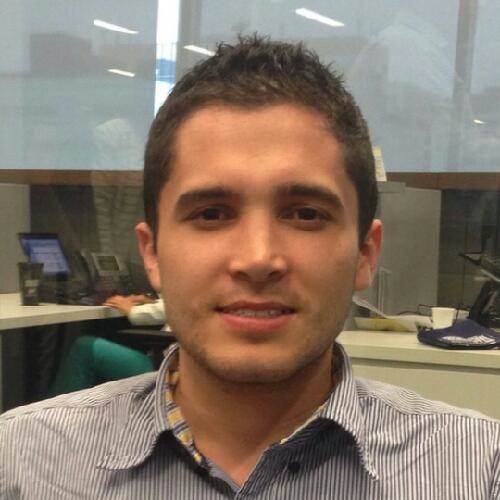
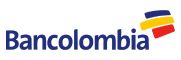