Securing CakePHP Applications with Authentication Middleware
In today’s digital landscape, web application security is of paramount importance. With cyber threats becoming increasingly sophisticated, developers must adopt robust security measures to protect user data and sensitive information. One fundamental aspect of web application security is user authentication. CakePHP, a popular PHP framework known for its simplicity and elegance, offers a powerful middleware system that can be leveraged to implement effective authentication mechanisms. In this blog post, we will delve into the world of securing CakePHP applications by utilizing authentication middleware. We will explore the significance of authentication, the role of middleware, implementation steps, best practices, and provide you with code samples to get you started.
Table of Contents
1. Understanding the Significance of Authentication
Authentication is the process of verifying the identity of a user, ensuring that they are who they claim to be before granting access to specific resources or functionalities within an application. Without proper authentication, unauthorized users could potentially gain access to sensitive information or manipulate the application’s behavior.
In the context of CakePHP applications, implementing robust authentication is crucial to safeguard user accounts, personal data, and maintain the integrity of the application. Authentication prevents unauthorized access, protects against identity theft, and helps in meeting regulatory compliance requirements.
2. The Role of Middleware in CakePHP
Middleware, in the context of web development, acts as a bridge between the server and application. It intercepts incoming requests, performs certain actions, and then passes the request along to the next middleware or the application itself. This architecture allows developers to modularize functionalities like authentication, logging, and more.
In CakePHP, middleware provides a flexible and elegant way to implement authentication checks across your application’s routes. By integrating authentication logic into middleware, you can enforce security measures consistently without cluttering your controllers with authentication-related code.
3. Implementing Authentication Middleware in CakePHP
Let’s dive into the steps of implementing authentication middleware in your CakePHP application. For the sake of this example, we will demonstrate how to set up a basic authentication middleware using the built-in tools provided by CakePHP.
Step 1: Create the Middleware
Start by creating a new middleware class. You can do this using CakePHP’s command-line tool:
bash bin/cake bake middleware Authentication
This command generates a new middleware file named AuthenticationMiddleware.php in the src/Middleware directory of your CakePHP application.
Step 2: Configure the Middleware
Open the generated AuthenticationMiddleware.php file and implement the authentication logic. Here’s a simplified example that uses CakePHP’s authentication component:
php // src/Middleware/AuthenticationMiddleware.php namespace App\Middleware; use Cake\Http\Middleware\Middleware; use Cake\Http\Response; use Cake\Http\ServerRequest; use Cake\Routing\Router; class AuthenticationMiddleware extends Middleware { public function process(ServerRequest $request, Response $response, $next) { $user = $this->request->getAttribute('identity'); if (!$user) { return $this->unauthenticatedResponse(); } return $next($request, $response); } protected function unauthenticatedResponse() { $response = new Response(); $response = $response->withLocation(Router::url(['controller' => 'Users', 'action' => 'login'])); return $response->withStatus(302); } }
In this example, the middleware checks if the user is authenticated (i.e., has an identity attribute). If not, it redirects them to the login page.
Step 3: Apply the Middleware
To apply the authentication middleware, you need to configure your application’s middleware pipeline. Open the src/Application.php file and add the following to the middleware method:
php // src/Application.php public function middleware(MiddlewareQueue $middlewareQueue): MiddlewareQueue { $middlewareQueue // Other middleware ->add(new AuthenticationMiddleware()); return $middlewareQueue; }
Ensure that the use statement for MiddlewareQueue is included at the top of the file.
4. Best Practices for CakePHP Authentication Middleware
While implementing authentication middleware, it’s essential to follow best practices to ensure the security and effectiveness of your application’s authentication system. Here are some key guidelines to keep in mind:
4.1. Use Strong Authentication Methods
Utilize secure authentication methods, such as password hashing, multi-factor authentication, and OAuth, to enhance the protection of user accounts.
4.2. Implement Role-Based Access Control
Incorporate role-based access control (RBAC) to restrict users’ actions based on their roles and permissions. CakePHP provides tools to manage roles and permissions effectively.
4.3. Secure Session Management
Pay attention to session management and implement measures like session expiration, regeneration, and secure cookie settings to mitigate session-related vulnerabilities.
4.4. Regularly Update Dependencies
Keep your CakePHP framework and other dependencies up to date. This ensures that you benefit from the latest security patches and improvements.
4.5. Protect Against Cross-Site Request Forgery (CSRF)
Implement CSRF protection to prevent attackers from executing unauthorized actions on behalf of authenticated users.
Conclusion
In the world of web application security, user authentication stands as a cornerstone of protection against unauthorized access and data breaches. CakePHP’s middleware system empowers developers to seamlessly integrate robust authentication mechanisms into their applications. By following the implementation steps outlined in this article and adhering to best practices, you can bolster the security of your CakePHP applications and instill confidence in your users. Remember, security is an ongoing process, so stay vigilant, keep learning, and adapt to emerging security challenges for a safer digital experience.
Table of Contents
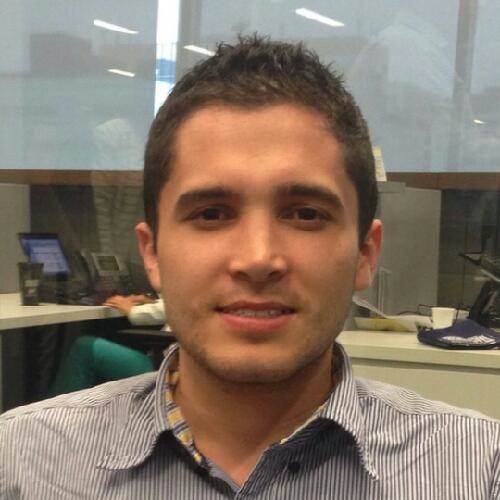
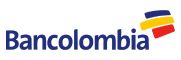