Building a Blogging API with CakePHP and JSON Web Tokens (JWT)
In today’s interconnected world, APIs (Application Programming Interfaces) have become a crucial part of web development. They allow different applications to communicate and exchange data seamlessly. One common use case is building a Blogging API that enables content management from various platforms. In this tutorial, we will explore how to create a robust Blogging API using CakePHP, a popular PHP framework, and secure it with JSON Web Tokens (JWT).
Table of Contents
1. Introduction to CakePHP and JWT
CakePHP is a rapid development framework for PHP that provides an elegant syntax and set of tools to build web applications. It follows the Model-View-Controller (MVC) architectural pattern and includes features like routing, ORM (Object-Relational Mapping), and scaffolding for quick prototyping.
JSON Web Tokens (JWT), on the other hand, are a compact and self-contained way to securely transmit information between parties as a JSON object. JWTs are commonly used for authentication and authorization in web applications. They consist of three parts: a header, a payload, and a signature, which are encoded and combined into a single string.
2. Setting Up the Project
2.1. Installing CakePHP
To get started, make sure you have Composer installed, a dependency manager for PHP. Create a new CakePHP project using the following command:
bash composer create-project --prefer-dist cakephp/app blog-api
2.2. Database Configuration
Configure your database settings in the config/app.php file. Modify the Datasources section to match your database credentials:
php 'Datasources' => [ 'default' => [ 'host' => 'localhost', 'username' => 'your_username', 'password' => 'your_password', 'database' => 'blog_api', // ... ], ],
3. Designing the API’s Database Structure
A simple Blogging API might consist of two main tables: posts and users. The posts table will store information about blog posts, including id, title, content, and created.
The users table will be used for authentication and will store fields like id, username, and password.
Set up these tables using CakePHP’s migration tool:
bash bin/cake bake migration CreatePosts title:string content:text created:datetime bin/cake bake migration CreateUsers username:string password:string bin/cake migrations migrate
4. Creating API Endpoints
Now it’s time to create the API endpoints for managing blog posts.
4.1. Retrieving Blog Posts
Create a controller for handling blog posts:
bash bin/cake bake controller Posts
In the src/Controller/PostsController.php file, implement the index method to retrieve and return a list of blog posts:
php public function index() { $posts = $this->Posts->find('all'); $this->set([ 'posts' => $posts, '_serialize' => ['posts'], ]); }
Configure routes for the API in config/routes.php:
php Router::scope('/api', ['prefix' => 'api'], function (RouteBuilder $routes) { $routes->connect('/posts', ['controller' => 'Posts', 'action' => 'index']); });
You can now access the list of posts using the URL: /api/posts
4.2. Creating a New Post
Implement the add method in PostsController.php to create a new post:
php public function add() { $post = $this->Posts->newEmptyEntity(); if ($this->request->is('post')) { $post = $this->Posts->patchEntity($post, $this->request->getData()); if ($this->Posts->save($post)) { $this->set([ 'post' => $post, '_serialize' => ['post'], ]); } } }
Add a new route for creating posts:
php $routes->connect('/posts/add', ['controller' => 'Posts', 'action' => 'add']);
4.3. Updating an Existing Post
For updating posts, implement the edit method:
php public function edit($id) { $post = $this->Posts->get($id); if ($this->request->is(['post', 'put'])) { $post = $this->Posts->patchEntity($post, $this->request->getData()); if ($this->Posts->save($post)) { $this->set([ 'post' => $post, '_serialize' => ['post'], ]); } } }
Add a new route for updating posts:
php $routes->connect('/posts/edit/:id', ['controller' => 'Posts', 'action' => 'edit']) ->setPass(['id']);
4.4. Deleting a Post
Lastly, implement the delete method to remove a post:
php public function delete($id) { $this->request->allowMethod(['delete']); $post = $this->Posts->get($id); if ($this->Posts->delete($post)) { $this->set([ 'message' => 'Deleted', '_serialize' => ['message'], ]); } }
Configure the delete route:
php $routes->connect('/posts/delete/:id', ['controller' => 'Posts', 'action' => 'delete']) ->setPass(['id']);
5. Implementing JSON Web Tokens (JWT) for Authentication
To secure your API, you’ll need to implement JWT-based authentication.
5.1. Installing the firebase/php-jwt Library
Use Composer to install the firebase/php-jwt library:
bash composer require firebase/php-jwt
5.2. Generating JWTs
Create an authentication endpoint in UsersController.php:
php use Firebase\JWT\JWT; // ... public function login() { if ($this->request->is('post')) { $user = $this->Auth->identify(); if (!$user) { throw new UnauthorizedException('Invalid credentials'); } $token = JWT::encode(['sub' => $user['id']], 'your_secret_key'); $this->set([ 'token' => $token, '_serialize' => ['token'], ]); } }
Add a new route for the login endpoint:
php $routes->connect('/users/login', ['controller' => 'Users', 'action' => 'login']);
5.3. Authenticating API Requests
In your API methods, you can now use JWT for authentication:
php use Firebase\JWT\JWT; use Cake\Http\Exception\UnauthorizedException; // ... public function edit($id) { $token = $this->request->getHeaderLine('Authorization'); try { $decoded = JWT::decode($token, 'your_secret_key', ['HS256']); // Proceed with updating the post } catch (Exception $e) { throw new UnauthorizedException('Invalid token'); } }
6. Securing Routes and Enhancing Authorization
You can enhance authorization by using middleware to restrict access to certain routes based on user roles and permissions.
Conclusion
In this tutorial, we’ve covered the process of building a Blogging API using CakePHP and securing it with JSON Web Tokens (JWT). We explored creating API endpoints for managing blog posts, generating and using JWTs for authentication, and enhancing authorization. This API can serve as a foundation for your own content management system, providing a secure and efficient way to manage blog posts from different platforms.
Table of Contents
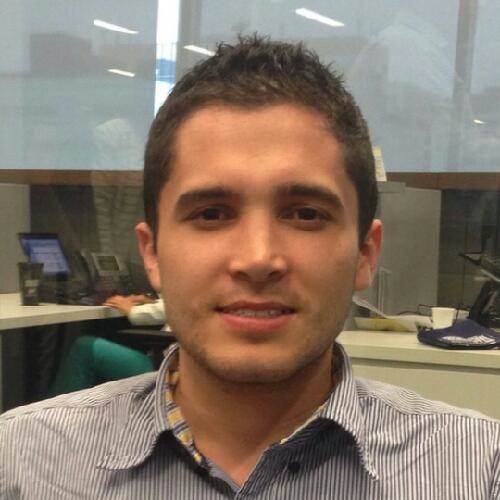
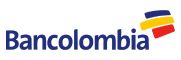