Building a Blogging Platform with CakePHP: A Step-by-Step Tutorial
Blogging has become an integral part of the online world, providing a platform for individuals and businesses to share information, ideas, and engage with their audience. If you’re considering building your blogging platform, CakePHP offers an excellent foundation to create a robust and feature-rich solution. CakePHP is a popular PHP framework known for its simplicity, ease of use, and scalability.
Table of Contents
In this step-by-step tutorial, we’ll guide you through the process of building a blogging platform using CakePHP. We’ll cover everything from setting up the environment to implementing essential features like user authentication, post creation, and commenting functionality. By the end of this tutorial, you’ll have a functional blogging platform that you can expand and customize to suit your specific needs.
Prerequisites
Before we dive into the development process, make sure you have the following prerequisites in place:
- Basic knowledge of PHP and MySQL.
- PHP installed on your development machine.
- A web server like Apache or Nginx.
- Composer, the PHP package manager, installed on your system.
Step 1: Setting Up the Project
The first step is to set up a new CakePHP project. Open your terminal and run the following command:
bash composer create-project --prefer-dist cakephp/app my_blog
This will create a new CakePHP project named ‘my_blog’ in the current directory.
Step 2: Database Configuration
Next, we need to configure the database for our blogging platform. Navigate to the ‘config’ folder in your project and open ‘app.php’. Locate the ‘Datasources’ section and update the ‘default’ connection with your database credentials:
php 'Datasources' => [ 'default' => [ 'host' => 'localhost', 'username' => 'your_database_username', 'password' => 'your_database_password', 'database' => 'your_database_name', // ...other settings ], ],
Replace ‘your_database_username’, ‘your_database_password’, and ‘your_database_name’ with your actual database credentials.
Step 3: Creating the Database Tables
Now, let’s create the necessary tables in the database for our blogging platform. CakePHP comes with a built-in command-line tool called ‘bake’ that helps us generate code quickly. Run the following command to create the tables:
bash bin/cake bake migration create_posts_table
This will generate a migration file for creating the ‘posts’ table. Open the generated file in the ‘config/Migrations’ folder and define the table structure:
php use Migrations\AbstractMigration; class CreatePostsTable extends AbstractMigration { public function change() { $table = $this->table('posts'); $table->addColumn('title', 'string', ['limit' => 255]); $table->addColumn('body', 'text'); $table->addColumn('created', 'datetime'); $table->addColumn('modified', 'datetime'); $table->create(); } }
Run the migration to create the ‘posts’ table:
bash bin/cake migrations migrate
Step 4: Creating the Model, View, and Controller for Posts
With the ‘posts’ table in place, we can now generate the Model, View, and Controller (MVC) files for handling posts. Run the following command:
bash bin/cake bake all posts
This will create the necessary files for the ‘posts’ resource, including the Model, Controller, and basic CRUD views.
Step 5: Implementing User Authentication
For our blogging platform, it’s essential to have user authentication to manage authors and prevent unauthorized access. Thankfully, CakePHP provides a convenient way to integrate user authentication using ‘bake’. Run the following command:
bash bin/cake bake controller Users
This will create a basic UsersController. Now, let’s generate the User model and user authentication:
bash bin/cake bake model Users bin/cake bake template Users bin/cake bake template Users login
Now, open the ‘UsersController.php’ file and modify it to include authentication actions:
php use Cake\Controller\Controller; class UsersController extends AppController { public function initialize() { parent::initialize(); $this->loadComponent('Auth'); } public function beforeFilter(Event $event) { parent::beforeFilter($event); $this->Auth->allow(['add', 'login']); } public function login() { if ($this->request->is('post')) { $user = $this->Auth->identify(); if ($user) { $this->Auth->setUser($user); return $this->redirect($this->Auth->redirectUrl()); } $this->Flash->error(__('Invalid username or password, try again')); } } public function logout() { return $this->redirect($this->Auth->logout()); } }
In the above code, we enabled the Auth component, allowed public access to ‘add’ and ‘login’ actions, and implemented the login and logout functionalities.
Step 6: Creating Views for User Authentication
Next, we need to create views for user authentication. Open the ‘login.ctp’ file under ‘src/Template/Users’ and add the following code:
html <h1>Login</h1> <?= $this->Form->create() ?> <?= $this->Form->control('username') ?> <?= $this->Form->control('password') ?> <?= $this->Form->button(__('Login')) ?> <?= $this->Form->end() ?>
This creates a simple login form with username and password fields.
Step 7: Implementing Blog Post Creation
Now that we have user authentication in place, let’s implement the functionality to create blog posts. Open the ‘PostsController.php’ file and add the following code:
php class PostsController extends AppController { public function beforeFilter(Event $event) { parent::beforeFilter($event); $this->Auth->allow(['index', 'view']); } public function add() { $post = $this->Posts->newEmptyEntity(); if ($this->request->is('post')) { $post = $this->Posts->patchEntity($post, $this->request->getData()); $post->user_id = $this->Auth->user('id'); if ($this->Posts->save($post)) { $this->Flash->success(__('The post has been saved.')); return $this->redirect(['action' => 'index']); } $this->Flash->error(__('Unable to add the post.')); } $this->set('post', $post); } }
In the above code, we’ve created an ‘add’ action that handles the blog post creation. We associate the currently logged-in user with the post, so they become the author. The ‘beforeFilter’ method allows public access to ‘index’ and ‘view’ actions while requiring authentication for ‘add’.
Step 8: Creating a Blog Post Form
To create a form for adding blog posts, open the ‘add.ctp’ file under ‘src/Template/Posts’ and add the following code:
html <h1>Add Post</h1> <?= $this->Form->create($post) ?> <?= $this->Form->control('title') ?> <?= $this->Form->control('body', ['rows' => '5']) ?> <?= $this->Form->button(__('Submit')) ?> <?= $this->Form->end() ?>
This will create a form with ‘title’ and ‘body’ fields for adding blog posts.
Step 9: Displaying Blog Posts
Now, let’s create a view to display all the blog posts. Open the ‘index.ctp’ file under ‘src/Template/Posts’ and add the following code:
html <h1>Blog Posts</h1> <table> <tr> <th>Title</th> <th>Created</th> <th>Actions</th> </tr> <?php foreach ($posts as $post): ?> <tr> <td><?= h($post->title) ?></td> <td><?= h($post->created->format('Y-m-d H:i:s')) ?></td> <td><?= $this->Html->link('View', ['action' => 'view', $post->id]) ?></td> </tr> <?php endforeach; ?> </table>
The above code will display a table with all the blog posts’ titles and creation dates.
Conclusion
Congratulations! You’ve successfully built a functional blogging platform with CakePHP. In this tutorial, we covered setting up the project, implementing user authentication, creating blog posts, and displaying them on the platform. You can further enhance the platform by adding features like categories, tags, and commenting functionality.
CakePHP’s flexibility and simplicity make it an excellent choice for building web applications, and with the foundation you’ve created, you have a solid starting point to customize and expand your blogging platform to meet your specific requirements. Happy blogging!
Table of Contents
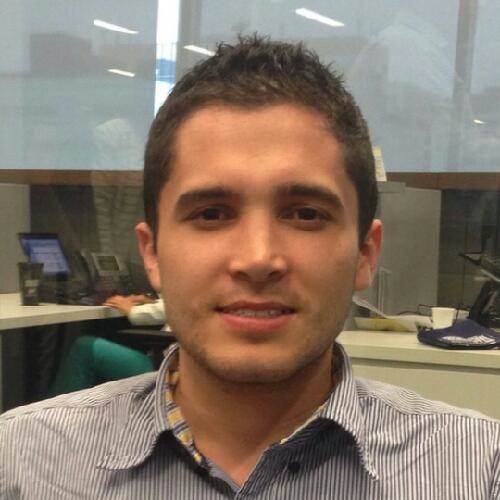
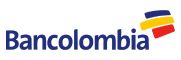