Building a CMS with CakePHP: Content Management Made Easy
In today’s digital age, managing and organizing content effectively is crucial for businesses and individuals alike. A content management system (CMS) is a powerful tool that simplifies the process of creating, editing, organizing, and publishing digital content. With the right framework, building a CMS can be a seamless process, and that’s where CakePHP comes into play. CakePHP, a popular PHP framework, provides developers with a robust foundation to create feature-rich web applications, including content management systems. In this tutorial, we will delve into the process of building a CMS with CakePHP, exploring the necessary steps, code samples, and best practices.
Table of Contents
1. Introduction to CakePHP and Content Management Systems
1.1. Understanding CakePHP Framework
CakePHP is a widely used open-source PHP web application framework that follows the Model-View-Controller (MVC) architectural pattern. It provides a structured and organized way to develop web applications, making it an excellent choice for building content management systems. With features like built-in validation, database access, and templating, CakePHP streamlines the development process.
1.2. Exploring the Need for a CMS
A Content Management System simplifies the process of managing digital content such as articles, images, videos, and more. It allows users to create, edit, organize, and publish content without requiring deep technical knowledge. Whether it’s a blog, an e-commerce site, or a news portal, a CMS makes content management accessible and efficient.
2. Setting Up the CakePHP Project
2.1. Installing CakePHP via Composer
To start building our CMS, we first need to set up a CakePHP project. The easiest way is by using Composer, a dependency management tool for PHP. Open your terminal and run the following command:
bash composer create-project --prefer-dist cakephp/app cms
This command creates a new CakePHP project in a directory named “cms.” Composer will download the necessary files and set up the basic project structure.
2.2. Database Configuration and Setup
CakePHP uses a configuration file named app.php located in the config directory to manage various settings, including database configuration. Open config/app.php and locate the ‘Datasources’ section. Update the ‘default’ datasource configuration with your database credentials:
php 'Datasources' => [ 'default' => [ 'host' => 'localhost', 'username' => 'your_username', 'password' => 'your_password', 'database' => 'cms_database', // ... ], // ... ],
Replace ‘your_username’, ‘your_password’, and ‘cms_database’ with your actual database credentials and desired database name.
3. Creating the Basic Structure
3.1. Generating Models, Views, and Controllers
CakePHP’s powerful command-line tools allow you to quickly generate the essential components of your application. Run the following commands to generate a basic structure for our CMS:
bash # Generate a model and its corresponding database table bin/cake bake model Articles # Generate a controller for managing articles bin/cake bake controller Articles # Generate views for the Articles controller's actions bin/cake bake template Articles
These commands create the necessary files for managing articles. The model represents the data structure, the controller handles business logic, and the views handle the presentation.
3.2. Building the User Authentication System
Security is paramount in any CMS, and CakePHP provides built-in tools for user authentication. Generate the user authentication code using CakePHP’s Bake tool:
bash bin/cake bake controller Users # Generate views for the Users controller's actions bin/cake bake template Users # Generate the User model for authentication bin/cake bake model User
With these commands, you’ve generated the user authentication components, allowing you to create and manage user accounts securely.
4. Designing the Database Schema
4.1. Defining the Content Types
Before diving into building the CMS interface, design your database schema. Determine the types of content your CMS will manage. For instance, in a blogging CMS, you might have articles, images, and categories. Create corresponding database tables for each content type and define their fields.
4.2. Establishing Relationships between Tables
Use CakePHP’s associations to define relationships between tables. For example, an article might belong to a category, and each article might have multiple images. Define these associations in your model files to enable easy retrieval and manipulation of related data.
5. Building the Admin Panel
5.1. Creating the Dashboard Interface
The admin panel is the heart of a CMS, enabling users to manage content efficiently. Design a user-friendly dashboard where administrators can view, create, edit, and delete content. Utilize CakePHP’s baked views as a starting point and customize them to fit your CMS’s design.
5.2. Implementing CRUD Functionality for Content
CRUD (Create, Read, Update, Delete) operations are fundamental to a CMS. Implement these operations for each content type using CakePHP’s conventions and helpers. Leverage tools like FormHelper to create user-friendly input forms, and PaginatorHelper to manage pagination for large datasets.
php // Example: Creating a new article public function add() { $article = $this->Articles->newEmptyEntity(); if ($this->request->is('post')) { $article = $this->Articles->patchEntity($article, $this->request->getData()); if ($this->Articles->save($article)) { $this->Flash->success(__('The article has been saved.')); return $this->redirect(['action' => 'index']); } $this->Flash->error(__('Unable to add the article.')); } $this->set('article', $article); }
6. Implementing WYSIWYG Editor
6.1. Integrating TinyMCE for Rich Text Editing
To empower users with seamless content creation, integrate a WYSIWYG (What You See Is What You Get) editor. TinyMCE is a popular choice. Include TinyMCE’s JavaScript library in your project and apply it to the relevant input fields in your CMS.
html <!-- Include TinyMCE --> <script src="https://cdn.tiny.cloud/1/apiKey/tinymce/5/tinymce.min.js"></script> <script> // Apply TinyMCE to a textarea tinymce.init({ selector: 'textarea#content' }); </script>
6.2. Storing HTML-Formatted Content
When storing content from the WYSIWYG editor, ensure you properly sanitize and validate the HTML. CakePHP’s validation and security features can help prevent cross-site scripting (XSS) attacks and maintain data integrity.
7. Managing User Roles and Permissions
7.1. Setting Up Different User Roles
In a CMS, different users have varying levels of access and permissions. Implement role-based access control to restrict users’ actions based on their roles. Define roles such as “admin,” “editor,” and “author,” and associate these roles with appropriate permissions.
7.2. Restricting Access Based on User Role
Use CakePHP’s authorization and authentication features to control access to different parts of your CMS. Create authorization rules that dictate which users can perform certain actions, such as editing articles or managing categories.
8. Implementing Frontend Display
8.1. Designing Templates for Content Display
The frontend of your CMS should showcase content in an organized and appealing manner. Create templates using CakePHP’s layout and view system. Leverage dynamic data insertion to populate templates with content from your database.
8.2. Retrieving and Displaying Dynamic Content
Use CakePHP’s querying capabilities to retrieve dynamic content from the database and display it on the frontend. Utilize elements and partials to reuse common components across multiple views.
9. Adding Search Functionality
9.1. Integrating Search Functionality
Efficiently finding content is crucial in a CMS, especially as the content database grows. Implement a search functionality that allows users to search for specific keywords across different content types.
9.2. Implementing Full-Text Search
Leverage database-specific features, such as MySQL’s full-text search, to enhance the search functionality. Implement filters and sorting options to further refine search results.
10. Enhancing Security and Performance
10.1. Sanitizing User Inputs
Security vulnerabilities often arise from improper handling of user inputs. Use CakePHP’s security features, such as validation rules and form tampering protection, to prevent common security risks.
10.2. Caching Strategies for Better Performance
Optimize the performance of your CMS by implementing caching strategies. Cache frequently accessed data and views to reduce database queries and improve page load times.
11. Testing the CMS
11.1. Writing Unit and Functional Tests
Ensure the reliability and functionality of your CMS by writing tests. CakePHP provides testing tools for unit tests, integration tests, and functional tests. Test user authentication, CRUD operations, and other critical functionalities.
12. Deployment and Beyond
12.1. Preparing the CMS for Deployment
Before deploying your CMS, make necessary configurations for the production environment. Set up secure database connections, configure caching mechanisms, and fine-tune server settings for optimal performance.
12.2. Ongoing Maintenance and Updates
Once your CMS is live, regular maintenance and updates are essential. Keep the CakePHP framework, plugins, and libraries up to date to ensure security and compatibility. Monitor performance and user feedback to identify areas for improvement.
Conclusion
In conclusion, building a content management system with CakePHP empowers you to create a robust platform for managing digital content. With its MVC architecture, powerful command-line tools, and security features, CakePHP provides an excellent foundation for developing a feature-rich CMS. By following best practices, implementing user-friendly interfaces, and prioritizing security and performance, you can create a content management system that meets the needs of both administrators and end-users.
Remember, the key to success lies in continuous learning and improvement. As technologies evolve and user expectations change, stay up-to-date with the latest trends and keep enhancing your CMS to deliver a seamless content management experience.
Table of Contents
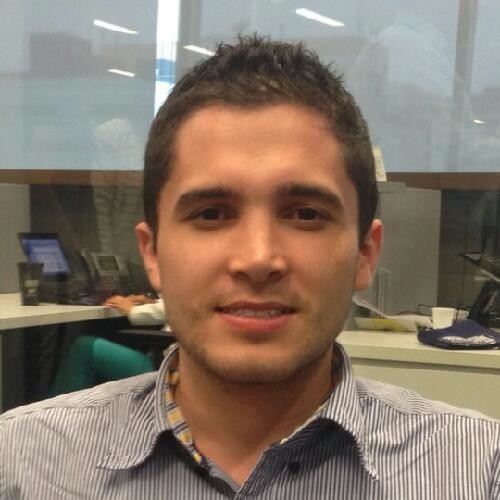
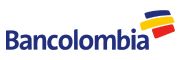