Building a Multi-Tenant Application with CakePHP
In today’s dynamic digital landscape, software applications are often required to cater to multiple clients or user groups, each with distinct requirements and data isolation needs. This demand has led to the rise of multi-tenant applications – applications that serve multiple tenants (clients or user groups) on a single codebase while keeping their data separate and secure. CakePHP, a popular PHP framework, provides an excellent foundation for building such applications. In this blog, we’ll explore the key concepts and steps involved in creating a multi-tenant application with CakePHP, along with code samples and best practices.
Table of Contents
1. Understanding Multi-Tenancy
Multi-tenancy is an architectural pattern where a single application instance serves multiple tenants. Each tenant is isolated from the others, maintaining its data, configurations, and customization while sharing the same application codebase. This approach offers various benefits, including cost efficiency, streamlined maintenance, and easier scalability.
2. Getting Started with CakePHP
Before diving into multi-tenancy, let’s briefly cover the basics of CakePHP. CakePHP is a powerful PHP framework that follows the Model-View-Controller (MVC) design pattern, providing developers with tools and conventions to build robust applications rapidly. It comes with features like ORM (Object-Relational Mapping), routing, authentication, and more, making it an ideal choice for complex projects like multi-tenant applications.
3. Setting Up Your CakePHP Project
- Installation: Begin by installing CakePHP using Composer, a dependency management tool for PHP. Run the following command to create a new CakePHP project:
bash composer create-project --prefer-dist cakephp/app my-multi-tenant-app
2. Database Configuration: Configure your database settings in config/app.php. This step is crucial as you’ll need separate databases or schema for each tenant.
3. Routes and Controllers: Define your application’s routes and create controllers for tenant-specific functionality. CakePHP’s routing system will play a pivotal role in directing requests to the appropriate tenant.
4. Implementing Multi-Tenancy in CakePHP
4.1. Database Separation
Each tenant requires a separate database or schema to maintain data isolation. CakePHP allows you to dynamically switch database connections based on the tenant’s context. Here’s how you can achieve this:
php // In config/app.php, define database configurations for each tenant 'databases' => [ 'tenant1' => [ 'datasource' => 'Database/Mysql', // ... ], 'tenant2' => [ 'datasource' => 'Database/Mysql', // ... ], // ... ] php // In your controller, dynamically switch the database connection $tenantConnection = 'tenant1'; // Fetch this based on the user's context $this->loadModel('Articles', $tenantConnection); $articles = $this->Articles->find('all')->toArray();
4.2. Tenant Identification
To identify the active tenant, you can use subdomains, URL parameters, or any other context-specific method. Subdomains are a common choice for multi-tenant applications. Here’s how to handle subdomains:
php // In config/routes.php, define a route that captures subdomains Router::scope('/', function (RouteBuilder $routes) { $routes->connect('/:tenant', ['controller' => 'Tenants', 'action' => 'view']) ->setPatterns(['tenant' => '[a-z0-9-]+']) ->setPass(['tenant']); }); php // In your controller, extract and set the active tenant $activeTenant = $this->request->getParam('tenant');
4.3. Shared vs. Tenant-Specific Code
Certain parts of your codebase will be shared among all tenants, while others will be customized for each tenant. Use CakePHP’s plugins, themes, and configuration overrides to manage this effectively.
For example, you can create a TenantTheme plugin to house tenant-specific views, styles, and templates. To load these views dynamically, utilize the plugin option in your controller:
php $this->viewBuilder()->setTemplatePath('TenantTheme');
4.4. Security and Data Isolation
Data isolation is crucial in multi-tenant applications to prevent cross-tenant data leakage. CakePHP’s ORM and query building capabilities make it easier to enforce data separation. Always use query conditions to restrict data retrieval based on the active tenant:
php $articles = $this->Articles->find('all') ->where(['tenant_id' => $activeTenant]) ->toArray();
5. Best Practices
- Testing: Implement unit and integration tests to ensure the stability of your multi-tenant application. Test scenarios involving different tenants, data isolation, and customization.
- Scalability: As your application grows, consider using techniques like database sharding and caching strategies to maintain optimal performance for all tenants.
- Security: Implement proper authentication and authorization mechanisms to ensure that tenants can only access their own data.
- Documentation: Clearly document your multi-tenancy implementation, including tenant onboarding, customization options, and database structure.
Conclusion
Building a multi-tenant application with CakePHP opens the doors to creating versatile, efficient, and scalable solutions for various clients or user groups. By understanding the key concepts of multi-tenancy, configuring databases, identifying tenants, and managing shared and tenant-specific code, you can develop a robust architecture that caters to diverse requirements while maintaining data isolation. Keep in mind the best practices and considerations to ensure a successful multi-tenant application that offers a seamless experience to each tenant while sharing the same codebase.
Incorporating multi-tenancy into your CakePHP projects can be complex, but the benefits in terms of efficiency, maintenance, and scalability make it a worthwhile endeavor. With the right approach and careful planning, you can create powerful applications that cater to multiple tenants without sacrificing data integrity or performance.
Table of Contents
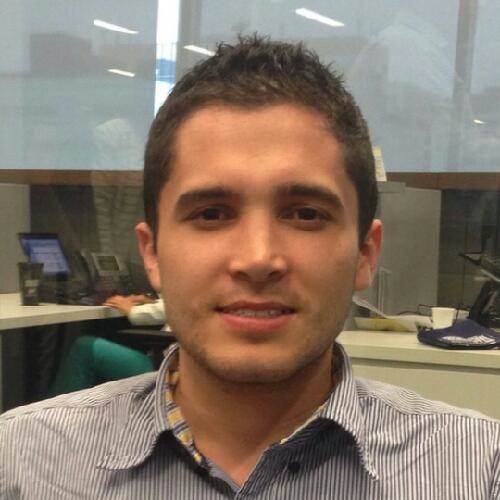
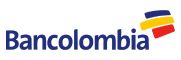