Building a RESTful JSON API with CakePHP and JWT Authentication
In the world of web development, creating APIs has become a crucial part of building modern applications. Representational State Transfer (REST) is a widely adopted architectural style for designing networked applications, and CakePHP is a popular PHP framework known for its flexibility and ease of use. In this tutorial, we’ll explore how to build a RESTful JSON API using CakePHP while implementing JSON Web Token (JWT) authentication for enhanced security.
Table of Contents
1. Prerequisites
Before diving into the tutorial, make sure you have the following prerequisites:
- Basic understanding of PHP and MVC architecture.
- Composer installed on your system.
- Familiarity with CakePHP framework.
- A code editor of your choice.
2. Setting Up the Project
To start building our RESTful API, we’ll first need to set up a new CakePHP project. If you haven’t already, install CakePHP using Composer with the following command:
bash composer create-project --prefer-dist cakephp/app my_api
This will create a new CakePHP project named “my_api” in a directory called “my_api.” Next, navigate to the project directory:
bash cd my_api
3. Creating the API Endpoint
Let’s begin by creating a simple API endpoint that retrieves a list of items. We’ll create a “Items” controller and corresponding routes for the API. Run the following command to generate the controller:
bash bin/cake bake controller Items
This command will create a new controller named “ItemsController” along with the necessary files.
4. Defining Routes
In the config/routes.php file, define the route for the API endpoint:
php use Cake\Routing\RouteBuilder; use Cake\Routing\Router; use Cake\Routing\Route\DashedRoute; Router::defaultRouteClass(DashedRoute::class); Router::scope('/', function (RouteBuilder $routes) { $routes->setExtensions(['json']); $routes->resources('Items'); });
This configuration will enable the API to respond to requests with the .json extension and map them to the ItemsController.
5. Creating the Model
Now, let’s create a model for our API. Run the following command to generate the model:
bash bin/cake bake model Items
This will create an “ItemsTable” class inside the src/Model/Table directory. Edit this file to define the database schema and relationships if necessary.
6. Building the Controller
Open the src/Controller/ItemsController.php file and update it with the following code:
php namespace App\Controller; use App\Controller\AppController; class ItemsController extends AppController { public function initialize(): void { parent::initialize(); $this->loadComponent('RequestHandler'); } public function index() { $items = $this->Items->find('all'); $this->set([ 'items' => $items, '_serialize' => ['items'] ]); } }
In this code, we’re using the RequestHandler component to automatically serialize our data to JSON format.
7. Testing the API
Start the built-in PHP server to test the API:
bash bin/cake server
Your API endpoint is now accessible at http://localhost:8765/items.json. Visiting this URL in your browser or using tools like cURL will return a JSON response with the list of items.
8. Implementing JWT Authentication
While our API is up and running, it’s important to secure it using authentication. JSON Web Tokens (JWT) provide a secure way to transmit information between parties. Let’s integrate JWT authentication into our CakePHP API.
8.1. Installing the Required Plugin
We’ll use the cakephp/authentication plugin for JWT authentication. Install the plugin using Composer:
bash composer require cakephp/authentication
8.2. Configuration
Next, enable the authentication component in your src/Controller/AppController.php file:
php namespace App\Controller; use Cake\Controller\Controller; use Cake\Event\EventInterface; class AppController extends Controller { public function initialize(): void { parent::initialize(); // ... $this->loadComponent('Authentication.Authentication', [ 'storage' => 'Memory', 'identifiers' => [ 'Authentication.JwtSubject', // You can add more identifiers here if needed ], 'unauthenticatedRedirect' => false, 'queryParam' => 'token', ]); // ... } }
In this configuration, we’re using the JwtSubject identifier, which expects the JWT token to be passed via the query parameter named “token.”
8.3. Protecting API Endpoints
To protect our API endpoints, we’ll add authentication middleware to the ItemsController. Open the src/Controller/ItemsController.php file and update the initialize method:
php namespace App\Controller; use App\Controller\AppController; class ItemsController extends AppController { public function initialize(): void { parent::initialize(); $this->loadComponent('RequestHandler'); $this->Authentication->allowUnauthenticated(['index']); } // ... }
In this code, we’re allowing unauthenticated access to the index action while protecting other actions by default.
8.4. Generating JWT Tokens
To generate JWT tokens, we’ll create a new controller action that handles user authentication and token generation. Create a new controller named “AuthController” using the following command:
bash bin/cake bake controller Auth
Open the src/Controller/AuthController.php file and add the following code:
php namespace App\Controller; use App\Controller\AppController; use Cake\Utility\Security; use Cake\I18n\Time; use Cake\Http\Exception\UnauthorizedException; use Lcobucci\JWT\Configuration; use Lcobucci\JWT\Signer\Hmac\Sha256; class AuthController extends AppController { public function token() { if (!$this->request->is('post')) { throw new MethodNotAllowedException(); } $username = $this->request->getData('username'); $password = $this->request->getData('password'); // Implement your authentication logic here // Example: Verify user credentials from the database if (!$authenticated) { throw new UnauthorizedException(__('Invalid credentials')); } $config = Configuration::forSymmetricSigner( new Sha256(), Security::getSalt() // Use a secure salt value ); $now = new Time(); $token = $config->builder() ->issuedBy('your_app') ->permittedFor('your_app_client') ->issuedAt($now) ->expiresAt($now->addHour(1)) ->withClaim('username', $username) ->getToken($config->signer(), $config->signingKey()); $this->set([ 'token' => $token->toString(), '_serialize' => ['token'] ]); } }
In this code, replace the authentication logic placeholder with your actual authentication process. The token action generates a JWT token and returns it in the response.
Conclusion
Congratulations! You’ve successfully built a RESTful JSON API using the CakePHP framework and implemented JWT authentication for secure communication. By following the steps in this tutorial, you’ve learned how to create API endpoints, protect them with JWT authentication, and generate JWT tokens for user authorization. This foundation can be extended to build more complex and feature-rich APIs to power your applications. Happy coding!
Table of Contents
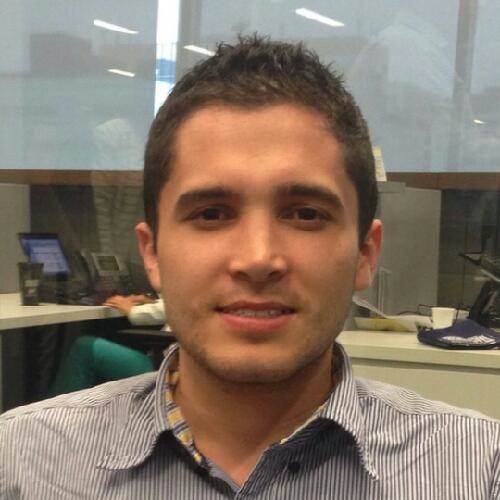
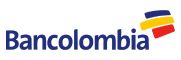