Building a Social Media Application with CakePHP
In today’s digital age, social media platforms have become an integral part of our lives, connecting people across the globe. If you’ve ever wondered how to create your own social media application, you’re in the right place. In this comprehensive guide, we’ll walk you through the process of building a social media application using CakePHP, a versatile and powerful PHP framework.
Table of Contents
1. Why CakePHP?
Before we dive into the nitty-gritty of building a social media application, let’s briefly discuss why CakePHP is an excellent choice for this project.
1.1. MVC Architecture
CakePHP follows the Model-View-Controller (MVC) architecture, which promotes a clean and organized code structure. This separation of concerns makes it easier to manage and scale your application as it grows.
1.2. Active Record Pattern
CakePHP uses the Active Record pattern, allowing you to interact with the database using an intuitive and object-oriented syntax. This simplifies database operations and reduces the need for complex SQL queries.
1.3. Built-in Security Features
Security is paramount in any social media application. CakePHP comes with built-in security features, including input validation, data sanitization, and protection against common web vulnerabilities like SQL injection and CSRF attacks.
1.4. Rapid Development
CakePHP offers a set of scaffolding tools and code generation features that accelerate the development process. This means you can prototype and launch your social media application faster.
Now that you understand why CakePHP is an excellent choice, let’s get started on the journey of creating your social media platform.
2. Prerequisites
Before we begin, make sure you have the following prerequisites in place:
- Web Server: You’ll need a web server (e.g., Apache, Nginx) to host your CakePHP application.
- PHP: CakePHP is built on PHP, so you should have PHP installed on your server. Ensure you have a version compatible with CakePHP (check CakePHP’s documentation for requirements).
- Composer: Composer is a PHP dependency manager. You’ll use it to install CakePHP and its dependencies. Download and install Composer from getcomposer.org.
- Database: Choose a database system (e.g., MySQL, PostgreSQL) for storing user data, posts, and other information. Make sure you have the necessary credentials and create a database for your application.
- Basic Understanding of PHP: Familiarity with PHP basics will be helpful, although CakePHP abstracts many complexities.
Now that you’ve covered the prerequisites, let’s move on to building your social media application step by step.
Step 1: Install CakePHP
The first step is to install CakePHP on your server. Open your terminal and navigate to your project directory. Run the following command to create a new CakePHP project:
bash composer create-project --prefer-dist cakephp/app my_social_media_app
This command will create a new CakePHP project named my_social_media_app in the current directory. Composer will automatically install CakePHP and its dependencies.
Step 2: Configure the Database
Next, you need to configure CakePHP to use your database. Navigate to the config directory in your project and copy the app.default.php file to app.php:
bash cd my_social_media_app/config cp app.default.php app.php
Now, open the app.php file and find the ‘Datasources’ section. Update the configuration with your database credentials:
php 'Datasources' => [ 'default' => [ 'className' => 'Cake\Database\Connection', 'driver' => 'Cake\Database\Driver\Mysql', // Change this to your database driver (e.g., Postgres, SQLite) 'persistent' => false, 'host' => 'localhost', // Your database host 'username' => 'your_username', // Your database username 'password' => 'your_password', // Your database password 'database' => 'your_database', // Your database name 'encoding' => 'utf8mb4', 'timezone' => 'UTC', 'cacheMetadata' => true, ], ],
Save the file with your changes.
Step 3: Create Models, Views, and Controllers
In CakePHP, Models represent database tables, Views are responsible for rendering content, and Controllers handle user requests and interact with Models. Let’s create these components for our social media application.
Creating a User Model
Run the following command to generate a User Model:
bash bin/cake bake model Users
CakePHP will create a User Model in the src/Model/Table directory. You can define user fields such as id, username, email, password, etc., in the generated file.
Creating a Posts Model
Similarly, create a Posts Model to handle user posts:
bash bin/cake bake model Posts
This command will generate a Posts Model in the src/Model/Table directory.
Creating Controllers and Views
Now, generate Controllers and Views for Users and Posts:
bash bin/cake bake controller Users bin/cake bake controller Posts
CakePHP will create the necessary Controllers and Views for managing users and posts.
Step 4: Routing and Views
CakePHP’s routing system allows you to define how URLs should be handled. Configure your routes in config/routes.php to map URLs to controllers and actions.
php use Cake\Routing\Route\DashedRoute; Router::defaultRouteClass(DashedRoute::class); Router::scope('/', function ($routes) { // Add routes for users and posts controllers here $routes->connect('/users', ['controller' => 'Users', 'action' => 'index']); $routes->connect('/posts', ['controller' => 'Posts', 'action' => 'index']); // ... });
You can add more routes as needed for user profiles, posting, and other features.
Creating Views
Create the necessary views for displaying user profiles, posts, and other pages. These views should be stored in the src/Template directory.
Step 5: User Registration and Authentication
User registration and authentication are crucial for any social media application. CakePHP simplifies this process with built-in tools and plugins.
Using CakePHP’s Authentication Plugin
CakePHP provides an authentication plugin that you can use to implement user registration and login. Install the plugin using Composer:
bash composer require cakephp/authentication
Enable the plugin by adding the following line to config/bootstrap.php:
php Plugin::load('Authentication');
Now, you can use the Authentication component in your UsersController to handle user registration and login.
php public function initialize(): void { parent::initialize(); $this->loadComponent('Authentication.Authentication'); }
The Authentication component simplifies user management, including password hashing, login, and session handling.
Step 6: Implement Social Media Features
With the basic structure in place, it’s time to add social media features to your application. Here are some essential features to consider:
1. User Profiles
Allow users to create and customize their profiles with profile pictures, bios, and other personal information.
2. Posting
Implement a posting system that allows users to create, edit, and delete posts. Posts should include text, images, and other media.
3. Friend Requests and Connections
Enable users to send and accept friend requests. Implement a system for managing connections and friendships.
4. Notifications
Implement a notification system to alert users about new friend requests, likes, comments, and other interactions.
5. News Feed
Create a personalized news feed for each user, displaying posts from their friends and connections.
Step 7: Testing and Debugging
Testing is crucial to ensure your social media application works as expected. CakePHP provides testing tools and a testing database configuration. Write test cases for your models, controllers, and views to identify and fix any issues.
Step 8: Deployment
Once you’ve thoroughly tested your social media application, it’s time to deploy it to a production server. Choose a reliable hosting provider and configure your server environment to meet the application’s requirements.
Conclusion
Building a social media application with CakePHP is an exciting journey that requires careful planning and development. With the right tools and knowledge, you can create a powerful and secure platform for connecting people online. Remember to keep security, scalability, and user experience in mind throughout the development process. Good luck with your social media project, and happy coding!
Table of Contents
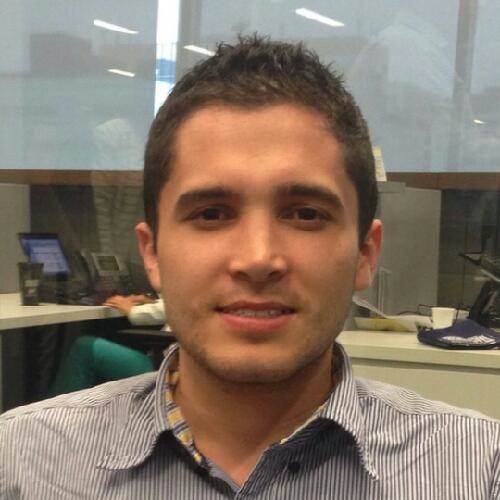
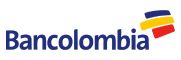