Building RESTful APIs with CakePHP
In today’s web development landscape, building robust and efficient RESTful APIs is a crucial skill for any developer. RESTful APIs allow applications to communicate and exchange data seamlessly, making them a fundamental component of modern web applications. If you’re looking to build RESTful APIs using a PHP framework, CakePHP is an excellent choice. In this blog post, we will explore how to leverage the power of CakePHP to create high-quality APIs that follow the REST architecture.
What is CakePHP?
CakePHP is an open-source PHP framework that provides a robust set of features and conventions for building web applications. It follows the MVC (Model-View-Controller) architectural pattern, promoting separation of concerns and code organization. CakePHP is known for its simplicity, rapid development capabilities, and support for modern web development practices.
Why Choose CakePHP for Building RESTful APIs?
CakePHP’s design and features make it an ideal choice for developing RESTful APIs. Here are a few reasons why CakePHP stands out:
- Convention over Configuration: CakePHP follows the principle of “convention over configuration,” providing sensible defaults and reducing the need for manual configuration. This convention-based approach simplifies API development and speeds up the development process.
- Data Modeling with ORM: CakePHP’s Object-Relational Mapping (ORM) system makes working with databases and data models a breeze. It allows you to define and manipulate database tables as objects, abstracting away the complexities of SQL queries and providing a seamless way to interact with your data.
- Built-in Routing: CakePHP’s powerful routing system enables you to define clean and expressive URL patterns for your API endpoints. It supports RESTful routes out of the box, making it easy to define routes for different HTTP methods and map them to corresponding controller actions.
- Request and Response Handling: CakePHP provides a robust Request and Response object abstraction, making it straightforward to handle incoming API requests and generate well-structured responses. It simplifies parameter handling, authentication, and input validation, ensuring your API remains secure and reliable.
- Authentication and Authorization: Securing APIs is a critical aspect of development. CakePHP offers built-in authentication and authorization components, making it easy to implement authentication schemes such as token-based authentication or OAuth. These components provide a solid foundation for securing your API endpoints.
Setting Up a CakePHP Project
Before we dive into building RESTful APIs with CakePHP, let’s quickly go through the setup process. Make sure you have PHP and Composer installed on your machine. Follow these steps to create a new CakePHP project:
Open your terminal and navigate to the desired directory for your project.
Run the following command to install CakePHP via Composer:
bash composer create-project --prefer-dist cakephp/app my_api_project
Once the installation is complete, navigate to the project directory:
bash cd my_api_project
Finally, start the built-in PHP server to run your CakePHP application:
bash bin/cake server
With these steps, you should have a working CakePHP project up and running locally.
Defining Routes for Your API
One of the first steps in building a RESTful API is defining the routes that map to different API endpoints. In CakePHP, you can define routes in the config/routes.php file. Let’s look at an example:
php use Cake\Routing\RouteBuilder; use Cake\Routing\Router; Router::scope('/api', function (RouteBuilder $routes) { // Define a GET route for retrieving all users $routes->get('/users', ['controller' => 'Users', 'action' => 'index']); // Define a POST route for creating a new user $routes->post('/users', ['controller' => 'Users', 'action' => 'add']); // Define a GET route for retrieving a specific user $routes->get('/users/:id', ['controller' => 'Users', 'action' => 'view']) ->setPass(['id']) ->setPatterns(['id' => '\d+']); // ... define more routes for your API endpoints });
In this example, we define routes for retrieving all users, creating a new user, and retrieving a specific user. We specify the corresponding controller and action for each route, allowing CakePHP to map the incoming requests to the appropriate controller methods.
Creating Controllers and Actions
Controllers in CakePHP handle the logic for processing API requests and generating responses. Let’s create a UsersController with some basic actions:
bash bin/cake bake controller Users
This command will generate a UsersController with CRUD actions (index, add, view, edit, delete) in the src/Controller directory.
Next, let’s implement the index action to retrieve all users:
php // src/Controller/UsersController.php namespace App\Controller; use Cake\ORM\TableRegistry; class UsersController extends AppController { public function index() { $usersTable = TableRegistry::getTableLocator()->get('Users'); $users = $usersTable->find()->all(); $this->set([ 'users' => $users, '_serialize' => 'users', ]); } }
In this example, we use the TableRegistry to fetch the Users table and retrieve all user records. We then set the retrieved users as the response data and specify the _serialize option to ensure the response is serialized correctly.
Serializing and Deserializing Data
When working with RESTful APIs, you often need to serialize and deserialize data to and from different formats like JSON or XML. CakePHP simplifies this process with the JsonView and XmlView classes. To enable JSON serialization for our API responses, follow these steps:
- Open src/Application.php.
- Uncomment the following line in the bootstrap() method:
php $this->addPlugin('Cake/JsonApi');
With this setup, CakePHP will automatically serialize the response data to JSON based on the _serialize option we defined in the controller.
Input Validation and Data Transformation
Validating incoming API requests and transforming data to meet specific requirements is crucial for building reliable APIs. CakePHP provides a robust validation system and data transformation capabilities. Here’s an example of how you can validate and transform request data in the add action of the UsersController:
php // src/Controller/UsersController.php public function add() { $user = $this->Users->newEmptyEntity(); $data = $this->getRequest()->getData(); $user = $this->Users->patchEntity($user, $data); if ($this->Users->save($user)) { $this->set([ 'user' => $user, '_serialize' => 'user', ]); } else { $errors = $user->getErrors(); $this->set([ 'errors' => $errors, '_serialize' => 'errors', ]); } }
In this example, we use the patchEntity() method to apply the incoming data to a new entity and validate it against the entity’s defined validation rules. If the data is valid, we save the user and return it in the response. Otherwise, we retrieve the validation errors and return them in the response.
Conclusion
CakePHP provides a solid foundation for building RESTful APIs. Its convention-based approach, powerful routing system, and integrated features like ORM, data validation, and serialization make it an excellent choice for API development. By following the best practices and leveraging the features provided by CakePHP, you can create robust and efficient APIs that meet the demands of modern web applications.
Remember, this blog post only scratches the surface of CakePHP’s capabilities for building RESTful APIs. I encourage you to explore the official CakePHP documentation and dive deeper into topics like authentication, versioning, and pagination to enhance your API development skills with CakePHP. Happy coding!
Table of Contents
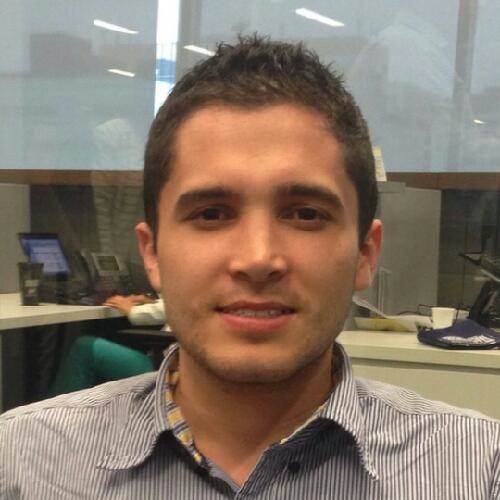
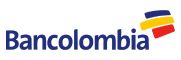