Building a Task Management System with CakePHP
In today’s fast-paced world, managing tasks efficiently is a crucial aspect of staying organized and productive. A well-designed Task Management System can significantly impact an individual’s or a team’s ability to achieve their goals and complete projects on time. In this tutorial, we’ll explore how to build a robust Task Management System using CakePHP – a powerful PHP framework known for its simplicity and rapid development capabilities. Whether you’re a developer looking to enhance your skills or a project manager aiming to streamline your team’s workflow, this guide will provide you with the necessary tools to create a tailored task management solution.
Table of Contents
1. Prerequisites
Before we dive into the development process, make sure you have the following prerequisites in place:
- Web Server: A web server (e.g., Apache) with PHP and a database (MySQL, PostgreSQL) installed.
- Composer: Composer is a dependency management tool for PHP. Install it on your system.
- CakePHP: Install CakePHP using Composer by running the following command:
bash composer create-project --prefer-dist cakephp/app task-management-system
This command will create a new CakePHP project named “task-management-system.”
2. Setting Up the Database
To start building our Task Management System, we need to set up the database structure to store tasks. CakePHP follows the convention-over-configuration principle, which makes it easy to work with databases.
- Database Configuration: Open config/app.php and configure the database settings according to your setup.
- Creating the Tasks Table: Run the following CakePHP console command to create a migration for the tasks table:
bash bin/cake bake migration CreateTasks
Edit the generated migration file (config/Migrations/YYYYMMDDHHMMSS_CreateTasks.php) to define the tasks table schema.
php // Inside the migration file public function change() { $table = $this->table('tasks'); $table->addColumn('title', 'string', ['limit' => 255]) ->addColumn('description', 'text') ->addColumn('due_date', 'datetime') ->addColumn('status', 'integer', ['default' => 0]) ->addTimestamps() ->create(); }
- Running Migrations: Execute the migration to create the tasks table in the database:
bash bin/cake migrations migrate
3. Creating the Task Model and Controller
With the database structure in place, let’s create the necessary CakePHP Model and Controller to manage tasks.
- Creating the Task Model: Run the following command to create a Task model:
bash bin/cake bake model Tasks
The model will be generated at src/Model/Table/TasksTable.php.
- Creating the Task Controller: Generate a controller for tasks using the following command:
bash bin/cake bake controller Tasks
This will create a TasksController at src/Controller/TasksController.php.
4. Building the User Interface
An intuitive user interface is essential for a successful Task Management System. We’ll create views using CakePHP’s template system and integrate user actions with the controller.
- Listing Tasks: In your src/Template/Tasks/index.ctp file, display a list of tasks fetched from the database:
php <h1>Task Management System</h1> <table> <tr> <th>Title</th> <th>Description</th> <th>Due Date</th> <th>Status</th> <th>Actions</th> </tr> <?php foreach ($tasks as $task): ?> <tr> <td><?= h($task->title) ?></td> <td><?= h($task->description) ?></td> <td><?= h($task->due_date) ?></td> <td><?= h($task->status) ?></td> <td> <?= $this->Html->link('Edit', ['action' => 'edit', $task->id]) ?> <?= $this->Form->postLink('Delete', ['action' => 'delete', $task->id], ['confirm' => 'Are you sure?']) ?> </td> </tr> <?php endforeach; ?> </table>
- Adding and Editing Tasks: Create forms in src/Template/Tasks/add.ctp and src/Template/Tasks/edit.ctp to allow users to add new tasks or edit existing ones.
php <!-- add.ctp --> <h1>Add Task</h1> <?= $this->Form->create($task) ?> <?= $this->Form->control('title') ?> <?= $this->Form->control('description', ['rows' => '3']) ?> <?= $this->Form->control('due_date', ['type' => 'date']) ?> <?= $this->Form->button('Save Task') ?> <?= $this->Form->end() ?> <!-- edit.ctp --> <h1>Edit Task</h1> <?= $this->Form->create($task) ?> <?= $this->Form->control('title') ?> <?= $this->Form->control('description', ['rows' => '3']) ?> <?= $this->Form->control('due_date', ['type' => 'date']) ?> <?= $this->Form->button('Save Task') ?> <?= $this->Form->end() ?>
5. Implementing Task Actions
Now that we have the user interface set up, let’s implement the actions in the controller to handle task operations.
- Listing Tasks: In the TasksController.php file, add the following code to the index method:
php public function index() { $tasks = $this->Tasks->find('all'); $this->set(compact('tasks')); }
- Adding Tasks: Implement the add method to allow users to add new tasks:
php public function add() { $task = $this->Tasks->newEmptyEntity(); if ($this->request->is('post')) { $task = $this->Tasks->patchEntity($task, $this->request->getData()); if ($this->Tasks->save($task)) { $this->Flash->success(__('The task has been saved.')); return $this->redirect(['action' => 'index']); } $this->Flash->error(__('Unable to add the task.')); } $this->set('task', $task); }
- Editing Tasks: Implement the edit method to allow users to edit existing tasks:
php public function edit($id = null) { $task = $this->Tasks->get($id); if ($this->request->is(['post', 'put'])) { $this->Tasks->patchEntity($task, $this->request->getData()); if ($this->Tasks->save($task)) { $this->Flash->success(__('The task has been updated.')); return $this->redirect(['action' => 'index']); } $this->Flash->error(__('Unable to update the task.')); } $this->set('task', $task); }
- Deleting Tasks: Implement the delete method to allow users to delete tasks:
php public function delete($id = null) { $this->request->allowMethod(['post', 'delete']); $task = $this->Tasks->get($id); if ($this->Tasks->delete($task)) { $this->Flash->success(__('The task has been deleted.')); } else { $this->Flash->error(__('Unable to delete the task.')); } return $this->redirect(['action' => 'index']); }
6. Styling Your Task Management System
A visually appealing interface enhances user experience. You can use CSS frameworks like Bootstrap or create custom styles to improve the look and feel of your Task Management System.
Conclusion
Congratulations! You’ve successfully built a Task Management System using CakePHP. By following this guide, you’ve learned how to set up a database, create models and controllers, design user interfaces, and implement essential task operations. This system forms a solid foundation that you can further enhance by adding features like user authentication, task priorities, and notifications. CakePHP’s flexibility and powerful features make it an excellent choice for rapidly developing robust web applications.
Remember that building software is an iterative process, and there’s always room for improvement. As you become more comfortable with CakePHP, consider exploring advanced topics like testing, caching, and performance optimization to create a truly exceptional Task Management System tailored to your needs. Happy coding!
Table of Contents
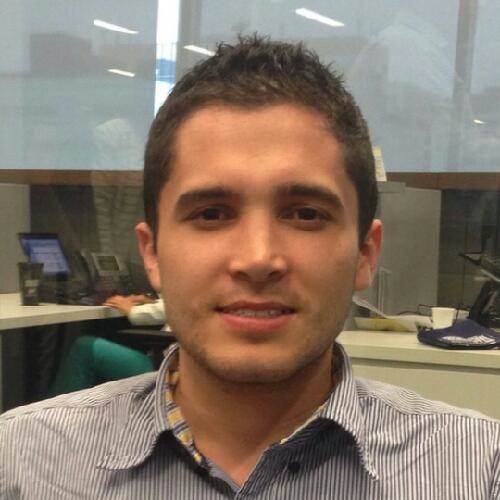
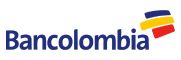