CakePHP Caching: Boost Performance and Scalability
In today’s fast-paced digital landscape, web application performance and scalability are crucial factors that can make or break the success of your project. Slow-loading pages and server overloads can lead to frustrated users and even impact your search engine rankings. To tackle these challenges and ensure a smooth user experience, implementing caching techniques is essential. CakePHP, being a powerful PHP framework, offers robust caching mechanisms that can significantly boost your application’s performance and scalability.
In this blog, we’ll explore the world of caching in CakePHP and demonstrate how to utilize it effectively to optimize your web application. We’ll delve into different caching strategies, provide code samples, and highlight the benefits of each approach.
1. Understanding Caching and its Benefits
1.1 What is Caching?
Caching is the process of storing frequently accessed data in a temporary storage area called a cache. When a user requests data from your application, the system first checks if the data is available in the cache. If found, it is retrieved from the cache instead of querying the database or executing expensive operations, significantly reducing response times.
1.2 Benefits of Caching
- Improved Performance: Caching ensures that frequently requested data is readily available, reducing the time needed to generate dynamic content, resulting in faster page loads and a smoother user experience.
- Reduced Database Load: By retrieving data from the cache, you minimize the number of database queries, easing the load on your database server and preventing potential bottlenecks.
- Enhanced Scalability: Caching allows your application to handle more concurrent users and traffic without compromising on performance.
- Cost-Efficiency: Caching reduces server load, leading to lower hosting costs, as you can manage more traffic with the same resources.
2. Types of Caching in CakePHP
2.1. View Caching
View caching involves caching the rendered view templates of your CakePHP application. This is particularly useful for pages that don’t change frequently, such as static content or pages with a relatively low update frequency.
2.2. Data Caching
Data caching focuses on caching the results of database queries or other expensive operations. By caching query results, you can avoid redundant database calls, making your application more responsive and efficient.
2.3. Query Caching
Query caching is a specific type of data caching that caches the results of CakePHP’s find queries. This can be very effective when your application executes similar find queries frequently.
3. Implementing View Caching
3.1. Enabling View Caching
In CakePHP, enabling view caching is straightforward. By using the built-in caching function Cache::write(), you can cache the rendered view output for a specified period.
php // In your controller action public function view_article($id) { // Your article retrieval logic $article = $this->Article->findById($id); // Enable view caching for this action $this->viewBuilder()->enableCache('my_view_cache', 'default', '+1 hour'); // Pass data to the view $this->set('article', $article); }
In this example, we use view caching for the “view_article” action. The rendered output for this action will be cached for one hour (‘+1 hour’).
3.2. Clearing View Cache
To clear the view cache when necessary (e.g., when an article is updated), you can use the Cache::delete() function.
php // In your controller action public function edit_article($id) { // Your article update logic // Clear the view cache for the "view_article" action Cache::delete('my_view_cache'); }
4. Implementing Data Caching
4.1. Enabling Data Caching
In CakePHP, data caching can be enabled by using the Cache::remember() method. This method retrieves data from the cache if available, and if not, it executes the provided callback function to fetch the data and store it in the cache.
php // In your controller action public function get_products() { $products = Cache::remember('product_list', function () { // Your database query to fetch products return $this->Product->find('all')->toArray(); }, 'default', '+1 hour'); $this->set('products', $products); }
In this example, the result of the database query will be cached as “product_list” for one hour.
4.2. Clearing Data Cache
Clearing data cache is similar to clearing view cache. You can use the Cache::delete() function to delete the cached data when needed.
php // In your controller action public function update_product($id) { // Your product update logic // Clear the data cache for "product_list" key Cache::delete('product_list'); }
5. Implementing Query Caching
5.1. Enabling Query Caching
Query caching in CakePHP is automatically enabled when you set the ‘cache’ option to ‘true’ while executing find queries.
php // In your controller action public function get_latest_products() { // Enable query caching for this find query $latestProducts = $this->Product->find('all', ['cache' => true]); $this->set('latestProducts', $latestProducts); }
In this example, the result of the find() query will be cached, and subsequent identical queries will be served from the cache.
5.2. Clearing Query Cache
To clear the query cache, you can use the Cache::clear() function with the appropriate parameters.
php // In your controller action public function add_product() { // Your product creation logic // Clear the query cache for the Product model Cache::clear(false, 'product'); }
Conclusion
CakePHP caching provides a powerful toolset to enhance your application’s performance and scalability. By implementing view caching, data caching, and query caching, you can significantly reduce response times, ease the load on your database, and ensure a smooth user experience even during peak traffic.
Remember that caching strategies should be applied wisely, as excessive caching can lead to stale data and potential consistency issues. By choosing the right caching approach for each scenario, you can strike the perfect balance between performance, scalability, and data freshness.
Optimize your CakePHP application today with caching, and witness the transformation in your website’s performance and user satisfaction. Happy coding!
Table of Contents
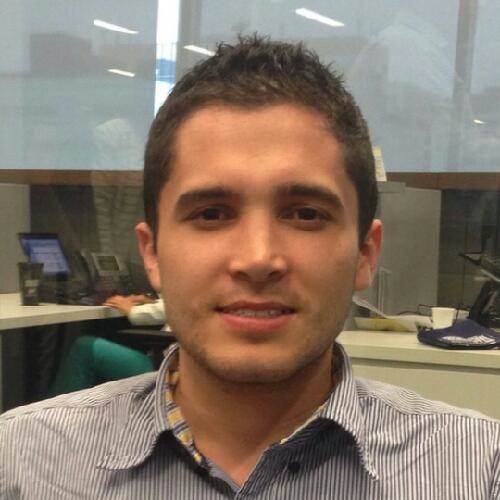
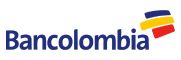