Implementing Caching in CakePHP with Redis
In today’s fast-paced digital landscape, delivering a seamless user experience is paramount for the success of web applications. One of the key aspects that directly influences user experience is performance. Slow-loading pages can drive users away, leading to decreased engagement and conversions. To mitigate this, implementing efficient caching mechanisms becomes crucial. In this guide, we’ll dive into how you can integrate caching into your CakePHP application using Redis, a powerful in-memory data store.
Table of Contents
1. Why Caching Matters
Caching is like a secret weapon that developers wield to optimize application performance. At its core, caching involves storing frequently accessed data in a temporary storage space. When a user requests that data again, the application can retrieve it quickly from the cache instead of re-fetching it from the database or performing complex computations.
For CakePHP applications, integrating caching can lead to significant improvements in response times, reduced database load, and ultimately a smoother user experience. Now, let’s explore how to implement caching using Redis with CakePHP.
2. Redis: A Brief Overview
Redis is an open-source, in-memory data store that is widely used for caching, real-time analytics, messaging, and more. Its lightning-fast read and write speeds make it an ideal choice for implementing caching in high-performance applications. Redis stores data in key-value pairs and keeps the entire dataset in memory, allowing for quick access and retrieval.
3. Setting Up Redis
Before we dive into integrating Redis with CakePHP, you need to ensure that Redis is properly set up and running on your server. Here’s a simplified guide to getting started:
- Installation: Install Redis on your server using the package manager specific to your operating system. For instance, on a Linux system, you can use the following command:
arduino sudo apt-get install redis-server
- Verification: After installation, verify that Redis is running by executing the following command:
redis-cli ping
If Redis is running, it will respond with “PONG.”
With Redis up and running, let’s move on to integrating it into your CakePHP application.
4. Integrating Redis Caching in CakePHP
CakePHP provides a flexible caching system that supports various caching engines, and Redis is one of them. Follow these steps to implement Redis caching in your CakePHP application:
Step 1: Configure Redis
Open the config/app.php file in your CakePHP application directory. Locate the ‘Cache’ configuration section and add a new configuration for Redis caching. Here’s an example configuration:
php 'Cache' => [ // ... other cache configurations 'redis' => [ 'className' => 'Cake\Cache\Engine\RedisEngine', // Redis server connection details 'server' => 'localhost', // Redis server hostname 'port' => 6379, // Redis server port // Optional Redis configuration options 'database' => 0, // Redis database index 'password' => null, // Redis authentication password ], ],
Step 2: Using Redis for Caching
With Redis configured, you can start using it for caching in your CakePHP application. The Cache class provides a convenient interface for working with various caching engines. To use Redis, simply specify ‘redis’ as the cache configuration name. Here’s how you can cache query results using Redis:
php // In your controller or model use Cake\Cache\Cache; // ... public function expensiveOperation() { $cacheKey = 'expensive_result'; // Try to get data from cache $result = Cache::read($cacheKey, 'redis'); if (!$result) { // Data not found in cache, perform the expensive operation $result = $this->performExpensiveOperation(); // Cache the result for future use Cache::write($cacheKey, $result, 'redis'); } return $result; }
In this example, the Cache::read method attempts to retrieve the data from the Redis cache. If the data is not found, the expensive operation is performed, and the result is stored in the cache using the Cache::write method.
Step 3: Cache Expiration and Invalidation
Caching data indefinitely is not always ideal, as data might become outdated or irrelevant over time. CakePHP allows you to set cache expiration times and provides mechanisms for cache invalidation. For instance, you can specify a cache duration when writing data to the cache:
php // Cache data for 1 hour Cache::write($cacheKey, $data, 'redis', '+1 hour');
You can also invalidate specific cache entries when relevant data changes:
php // Invalidation example when a new item is added public function addItem($item) { // ... add the item to the database // Invalidate the cache for the list of items Cache::delete('item_list', 'redis'); }
5. Best Practices for Redis Caching in CakePHP
While implementing Redis caching can greatly enhance your CakePHP application’s performance, it’s important to follow best practices to ensure effective caching:
- Selective Caching: Not all data needs to be cached. Focus on caching frequently accessed data that is resource-intensive to compute or fetch from the database.
- Cache Invalidation: Implement strategies to invalidate or update cache when relevant data changes. Stale data can lead to incorrect results and a poor user experience.
- Expiration: Set appropriate expiration times for cached data to ensure that users are always served fresh information when needed.
- Monitoring and Scaling: Monitor your Redis server’s performance and consider scaling options as your application grows to maintain optimal caching performance.
Conclusion
Caching is a powerful technique that can significantly improve the performance of your CakePHP application. By integrating Redis as a caching engine, you can take advantage of its lightning-fast in-memory data storage capabilities. This guide has walked you through the process of setting up Redis, configuring CakePHP to use Redis for caching, and implementing caching in your application. Following best practices ensures that your cached data remains relevant and contributes to an optimal user experience. With these tools in your arsenal, you’re well-equipped to create high-performing CakePHP applications that delight users with their responsiveness.
Incorporating caching with Redis in CakePHP might initially require some effort, but the dividends in terms of improved performance and a smoother user experience make it well worth it. So go ahead, supercharge your CakePHP application with Redis caching, and watch your application’s speed and efficiency soar!
Table of Contents
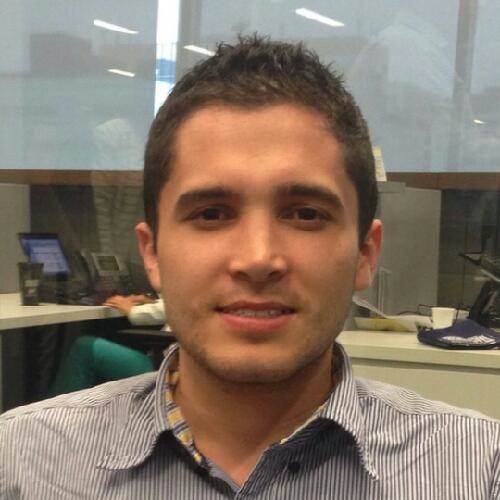
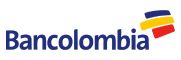