Working with CakePHP’s Command-Line Tools: Shells and Tasks
CakePHP, a popular PHP framework, empowers developers with a robust set of tools to streamline development processes. Among its many features, CakePHP’s command-line tools stand out for their ability to expedite various tasks, making development more efficient and organized. In this blog, we will dive into CakePHP’s command-line tools, specifically focusing on Shells and Tasks. These tools allow developers to automate tasks, manage the application’s CLI interface, and perform various operations swiftly.
Table of Contents
1. Understanding CakePHP Shells
In CakePHP, Shells are command-line scripts that provide a convenient interface for interacting with your application. Shells can be used to execute a variety of tasks, ranging from database migrations and data seeding to custom command execution. The framework ships with several built-in shells, and developers can create custom shells tailored to their application’s needs.
2. Built-in Shells
CakePHP comes with a set of built-in shells that cover common tasks. Let’s explore some of these:
2.1. Bake Shell
The Bake shell is a developer’s best friend when it comes to generating code quickly. It automates the process of creating controllers, models, views, and more, based on your application’s database schema. For instance, to generate a new controller for managing articles, you can run:
bash bin/cake bake controller Articles
2.2. Console Shell
The Console shell allows developers to interact with the framework’s ConsoleKit library. This provides a foundation for building custom command-line tools for your application. You can run custom commands using the Console shell, making it a versatile tool for automating various tasks.
2.3. Migration Shell
Managing database schema changes is crucial for any application’s evolution. The Migration shell simplifies this process by generating and executing migration files. These files define how the database schema should change over time, ensuring smooth transitions between different versions of your application.
3. Creating Custom Shells
While the built-in shells are powerful, you can create custom shells to address specific needs within your application. To create a custom shell, follow these steps:
- Navigate to the src/Shell directory of your CakePHP application.
- Create a new PHP file for your custom shell, e.g., MyCustomShell.php.
- Define your shell class by extending the Shell class provided by CakePHP.
- Implement the necessary command methods within your custom shell class.
Here’s a simple example of a custom shell that greets the user:
php // src/Shell/MyCustomShell.php namespace App\Shell; use Cake\Console\Shell; class MyCustomShell extends Shell { public function main() { $this->out('Hello, CakePHP Shell!'); } }
You can then run your custom shell using:
bash bin/cake my_custom
4. Leveraging CakePHP Tasks
In addition to shells, CakePHP’s command-line tools also include Tasks. While shells encapsulate larger commands, tasks represent smaller units of work that can be combined to create more complex operations.
4.1. Built-in Tasks
CakePHP offers several built-in tasks that can be used within your custom shells or invoked separately:
4.1.1. ExampleTask
As the name suggests, this is an example task provided by CakePHP. It showcases how to create and use tasks effectively.
4.1.2. FixtureTask
The Fixture task facilitates the management of test data. It allows developers to populate the database with predefined data for testing purposes. This is immensely useful when writing and executing unit tests.
4.1.3. ModelTask
The Model task provides command-line utilities for working with models. You can generate model classes, associations, validation rules, and more using this task. It complements the Bake shell’s capabilities by offering more granular control over model generation.
4.2. Creating Custom Tasks
Just like custom shells, you can create custom tasks tailored to your application’s requirements. Here’s how:
- Navigate to the src/Shell/Task directory of your CakePHP application.
- Create a new PHP file for your custom task, e.g., MyCustomTask.php.
- Define your task class by extending the Task class provided by CakePHP.
- Implement the necessary methods within your custom task class.
Let’s consider a simple example of a custom task that generates Lorem Ipsum text:
php // src/Shell/Task/MyCustomTask.php namespace App\Shell\Task; use Cake\Console\Shell; class MyCustomTask extends Shell { public function generateLoremIpsum() { $this->out('Lorem ipsum dolor sit amet...'); } }
You can then use your custom task within a shell or execute it directly from the command line.
5. Putting Shells and Tasks into Action
Now that you understand the concepts behind CakePHP’s Shells and Tasks, let’s see them in action with a real-world scenario.
Imagine you’re developing a blog application. You can create a custom shell named BlogShell that interacts with various tasks:
- ArticleImportTask: Imports articles from an external source.
- ImageResizeTask: Resizes and optimizes images for the blog.
- NotificationTask: Sends notifications to subscribers about new articles.
By combining these tasks within the BlogShell, you can automate the process of importing articles, optimizing images, and notifying subscribers whenever new content is added.
Conclusion
CakePHP’s command-line tools, Shells and Tasks, provide a powerful way to streamline development processes, automate tasks, and manage various operations efficiently. Whether you’re generating code, managing database migrations, or creating custom command-line tools, CakePHP’s command-line tools offer the flexibility and efficiency every developer desires. By mastering these tools, you can boost your productivity and create well-structured, feature-rich applications with ease.
Table of Contents
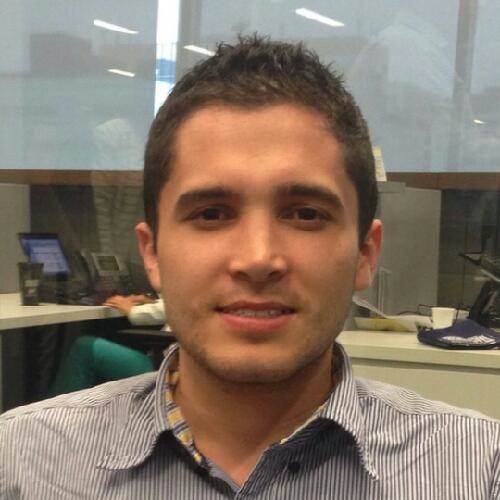
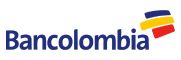