Understanding CakePHP Components: Reusable Building Blocks
In the world of web development, efficiency and reusability are key factors that contribute to successful and maintainable applications. CakePHP, a popular PHP framework, provides a wide range of features and tools to streamline the development process. One of its most powerful features is the concept of components. In this blog, we will delve into the world of CakePHP components, understanding what they are, how they work, and how they can become reusable building blocks for your web applications.
1. What are CakePHP Components?
In CakePHP, components are modular, reusable pieces of code that can be shared and used across multiple controllers. They encapsulate specific functionality, such as authentication, session management, pagination, or email handling. By using components, you can extract common code into reusable building blocks, promoting code reusability and reducing duplication.
2. Benefits of Using Components
2.1 Code Reusability: Components allow you to define functionality once and reuse it in multiple controllers. This not only reduces code duplication but also simplifies maintenance and updates.
2.2 Modularity: Components enable you to organize your code into logical units, making it easier to understand and manage. They encapsulate specific features or behaviors, promoting cleaner and more maintainable code.
2.3 Flexibility: With components, you can easily mix and match functionality based on your application’s needs. You can include and configure components in different controllers, tailoring them to specific requirements without affecting the overall architecture.
2.4 Extensibility: CakePHP components can be extended to add custom functionality or modify existing behavior. This allows you to leverage the power of the framework while tailoring it to your specific project requirements.
3. Creating and Implementing Components
3.1 Defining a Component:
To create a component in CakePHP, you need to define a class that extends the Component class provided by the framework. Let’s say we want to create a LoggingComponent that logs certain actions in our application. Here’s an example of how the component class would be defined:
php // app/Controller/Component/LoggingComponent.php class LoggingComponent extends Component { public function logAction($action) { // Perform logging logic here } }
3.2 Using Components in Controllers:
Once you’ve defined a component, you can include it in your controllers. In CakePHP, you can specify which components to load using the $components property. For our LoggingComponent, we can add it to a PostsController like this:
php // app/Controller/PostsController.php class PostsController extends AppController { public $components = ['Logging']; public function add() { // Perform action logic $this->Logging->logAction('Add post'); } }
3.3 Accessing Components in Views:
Components can also be accessed in your views to provide additional functionality. To access a component from a view, you can use the $this->ComponentName syntax. For example, if you wanted to display a log message in your view, you could do the following:
php // app/View/Posts/add.ctp <h1>Add Post</h1> <p><?php echo $this->Logging->logMessage; ?></p>
4. Sharing Data between Components and Controllers:
CakePHP provides several methods for sharing data between components and controllers. One common approach is to use component properties and controller variables. Components can set properties that controllers can access, allowing for seamless communication between the two.
5. Best Practices for Using Components:
To make the most out of CakePHP components, consider the following best practices:
5.1 Keep Components Focused: Components should have a clear and specific responsibility. Avoid creating components that handle too many unrelated functionalities.
5.2 Reusability and Extensibility: Design your components in a way that promotes reusability and extensibility. Keep them modular and flexible, allowing for easy integration into different projects.
5.3 Proper Documentation: Document your components thoroughly, including their purpose, usage, and configuration options. This will make it easier for other developers (including yourself) to understand and utilize the components.
Conclusion:
CakePHP components provide a powerful way to create reusable building blocks for your web applications. By encapsulating common functionality, components promote code reusability, modularity, and flexibility. They allow you to streamline your development process, making your code cleaner, more maintainable, and easier to manage. Understanding and utilizing CakePHP components will empower you to build efficient and robust web applications with ease.
Table of Contents
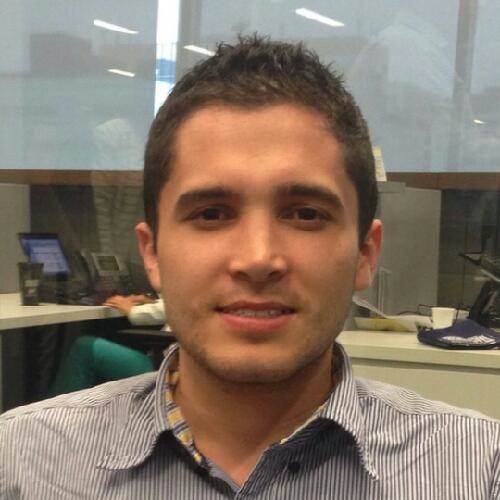
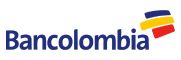