Working with CakePHP’s Console Task System
In the world of web development, efficiency and automation are key factors for success. CakePHP, a popular PHP framework, understands this well and offers a robust console task system that allows developers to perform various command-line operations effortlessly. In this blog post, we’ll delve into the details of CakePHP’s Console Task System, exploring its structure, creating custom tasks, and uncovering real-world use cases.
Table of Contents
1. Understanding CakePHP’s Console Task System
1.1. What are Console Tasks?
Console tasks in CakePHP are command-line scripts that enable developers to perform various tasks beyond the scope of regular web requests. These tasks can range from database migrations and data imports to scheduled batch processing and generating reports. Console tasks provide a bridge between the web application and the command-line interface, allowing developers to execute complex operations efficiently.
1.2. Benefits of Using Console Tasks
The use of console tasks offers several advantages:
- Efficiency: Complex operations that might take a long time to execute through web requests can be performed more efficiently using console tasks.
- Automation: Console tasks allow developers to automate repetitive tasks, reducing manual intervention and the chances of errors.
- Parallel Processing: Tasks can be run in parallel, making use of available system resources and speeding up the execution of time-consuming processes.
- Background Execution: Console tasks can be run in the background, freeing up the web application to handle user requests without interruptions.
- Access to Shell Environment: Console tasks provide access to the full power of the shell environment, enabling interactions with system tools and resources.
2. Exploring Console Task Structure
2.1. Built-in Tasks
CakePHP comes with a set of built-in console tasks that cover common scenarios. Some examples include:
- Database Migrations: Automate the process of applying changes to the database schema.
- Fixture Loading: Populate the database with test data using fixtures.
- Cache Management: Clear, build, and manage the application’s cache.
- Baking Code: Quickly generate code for models, controllers, and views.
2.2. Task Command Format
To execute a console task, you use the following command format:
php bin/cake <task_name> <action> <options>
- <task_name>: The name of the task you want to execute.
- <action>: The specific action or method within the task.
- <options>: Additional options or arguments required by the task.
For instance, to run the built-in database migration task to create a new table, the command would look like:
bash bin/cake migrations migrate create_table_name
3. Creating Custom Console Tasks
3.1. Generating a Basic Task
While the built-in tasks cover many scenarios, you might encounter situations where you need a custom task tailored to your application’s specific needs. Creating a custom task is straightforward:
- Use the CakePHP Console’s bake command to generate the basic structure of the task:
bash bin/cake bake task Custom
2. This will create a CustomTask.php file under the src/Console/Command/Task directory.
3.2. Implementing Task Logic
Once the task structure is generated, you can start implementing the task logic in the generated file. The task class should extend Shell and implement the desired functionality in its methods.
php // src/Console/Command/Task/CustomTask.php namespace App\Console\Command\Task; use Cake\Console\Shell; class CustomTask extends Shell { public function main() { $this->out('This is a custom task.'); } }
In this example, the main method contains the logic for your custom task. You can replace the output with your desired functionality, such as interacting with the database or performing calculations.
4. Real-world Use Cases
4.1. Database Migrations
Console tasks are particularly useful for managing database migrations. Instead of manually applying changes to the database schema, you can create migration tasks that automate this process. This ensures consistency and makes it easier to collaborate with other developers.
4.2. Batch Processing
Batch processing, such as data imports or updates, can be efficiently handled using console tasks. You can create a task that reads data from a file, processes it, and updates the database accordingly. This is especially valuable when dealing with large datasets that might exceed the memory limits of a web request.
4.3. Automated Reporting
Generating automated reports at specific intervals is another scenario where console tasks shine. You can create a task that pulls data from the database, processes it, and generates reports in various formats (PDF, CSV, etc.). This eliminates the need for manual report generation and distribution.
5. Tips for Effective Console Task Management
5.1. Logging and Error Handling
When working with console tasks, it’s important to implement proper logging and error handling mechanisms. Since tasks might run in the background, you need a way to monitor their progress and handle any exceptions that might occur.
5.2. Task Scheduling
CakePHP provides integration with task scheduling tools like cron jobs on Unix-like systems or Task Scheduler on Windows. This allows you to automate the execution of tasks at specific intervals, ensuring that critical operations are performed regularly without manual intervention.
Conclusion
CakePHP’s Console Task System empowers developers with a versatile toolset for executing command-line operations efficiently. Whether you’re managing database migrations, processing data in batches, or automating report generation, console tasks streamline the process and enhance overall productivity. By understanding the structure, creating custom tasks, and implementing best practices, you can harness the full potential of this powerful feature and take your application’s performance to new heights.
Table of Contents
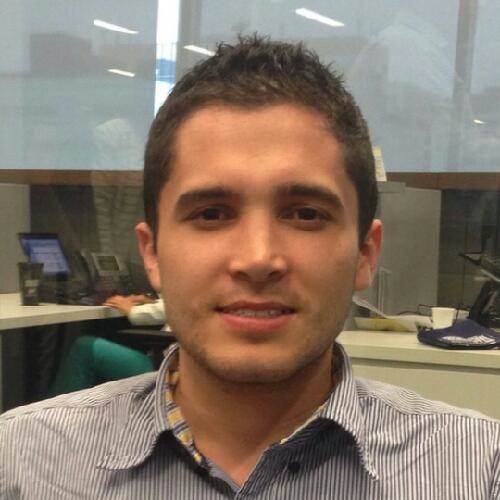
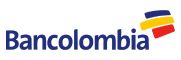