Working with CakePHP’s Cookie Component: Handling User Sessions
In web development, managing user sessions is a crucial aspect of ensuring a seamless and personalized user experience. CakePHP, a popular PHP framework, offers a robust Cookie Component that simplifies the process of handling user sessions. In this blog post, we’ll delve into the world of CakePHP’s Cookie Component and discover how it can be effectively utilized to manage user sessions within your web applications.
Table of Contents
1. Understanding User Sessions
1.1. What are User Sessions?
A user session is a temporary interaction between a user and a website or application. It allows the application to remember user-specific data and activities across multiple requests. Sessions enable the creation of personalized experiences, such as retaining items in a shopping cart or maintaining user login status.
1.2. The Role of Cookies in User Sessions
Cookies play a fundamental role in managing user sessions. When a user interacts with a website, a unique session ID is often stored in a cookie on the user’s device. This session ID is then sent along with each subsequent request, allowing the server to identify and associate the user with their specific session data.
2. Introduction to CakePHP’s Cookie Component
2.1. What is the Cookie Component?
CakePHP’s Cookie Component provides a convenient way to manage cookies and handle user sessions seamlessly. It abstracts the complexity of working with cookies directly, making it easier to read, write, and manipulate session data.
2.2. Advantages of Using the Cookie Component
- Simplicity: The Cookie Component simplifies the process of working with cookies and session data.
- Abstraction: Developers can focus on session logic rather than dealing with the intricacies of cookie management.
- Security: The component offers built-in security measures to mitigate common session-related vulnerabilities.
3. Integrating the Cookie Component
3.1. Enabling the Cookie Component
Integrating the Cookie Component into your CakePHP application is straightforward. You can enable it in your controller’s initialize method using the following code:
php // src/Controller/AppController.php public function initialize(): void { parent::initialize(); $this->loadComponent('Cookie'); }
3.2. Configuration Options
The Cookie Component comes with various configuration options that allow you to customize its behavior. Some common options include:
- expires: Sets the expiration time for the cookie.
- path: Specifies the path for which the cookie is available.
- secure: Determines whether the cookie should only be sent over HTTPS.
- httpOnly: Prevents client-side JavaScript from accessing the cookie.
Here’s an example of configuring the Cookie Component with custom options:
php // src/Controller/AppController.php public function initialize(): void { parent::initialize(); $this->loadComponent('Cookie', [ 'expires' => '+1 day', 'path' => '/', 'secure' => true, 'httpOnly' => true, ]); }
4. Working with User Sessions
4.1. Writing Data to the Session
To write data to the user session using the Cookie Component, you can use the write method. This method takes two arguments: the name of the session variable and the data you want to store. For example:
php // In a controller action $this->Cookie->write('username', 'john_doe');
4.2. Reading Data from the Session
Reading session data is equally straightforward. The read method allows you to retrieve the value of a session variable:
php // In another controller action $username = $this->Cookie->read('username');
4.3. Deleting Session Data
When you need to remove a session variable, you can use the delete method:
php // In a controller action $this->Cookie->delete('username');
5. Security Considerations
5.1. Preventing Session Tampering
Security is a vital aspect of user sessions. The Cookie Component helps prevent session tampering by using a combination of encryption and hashing techniques. This makes it challenging for attackers to modify the session data stored in cookies.
5.2. Regenerating Session IDs
To mitigate session fixation attacks, it’s recommended to regenerate session IDs periodically. The Cookie Component simplifies this process:
php // In a controller action (e.g., during user login) $this->Cookie->renew();
6. Best Practices
6.1. Keeping Session Data Minimal
While it’s convenient to store data in user sessions, it’s important to keep the data minimal and relevant. Storing excessive data in sessions can lead to performance issues and potential security risks.
6.2. Handling Expiry and Deletion
Set appropriate expiration times for your session cookies. Sessions that are no longer needed should be properly deleted to free up resources.
Conclusion
In conclusion, CakePHP’s Cookie Component offers a powerful and user-friendly way to manage user sessions in web applications. By enabling developers to handle session data efficiently and securely, the Cookie Component contributes to a better user experience. Understanding its features and following best practices empowers developers to build robust and secure web applications that make the most of user sessions.
Whether you’re building an e-commerce platform, a social networking site, or any other web application, mastering the art of user session management using CakePHP’s Cookie Component is a skill that can elevate your development projects to the next level.
Table of Contents
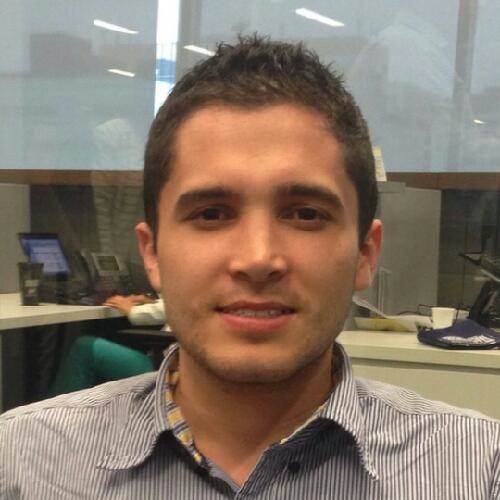
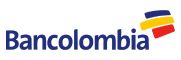