Creating Dynamic Forms with CakePHP FormHelper
Creating web forms is an essential part of building interactive and user-friendly websites. From simple contact forms to complex data entry interfaces, forms are the primary means through which users interact with websites. CakePHP, a popular PHP framework, offers a powerful tool called FormHelper that simplifies the process of creating and handling forms. In this tutorial, we’ll explore how to use CakePHP FormHelper to build dynamic forms efficiently. We’ll cover essential concepts, provide code samples, and guide you through each step of the process.
Table of Contents
Before diving into the details, let’s take a brief look at what CakePHP FormHelper is and why it’s a valuable tool for developers. FormHelper is a core utility in the CakePHP framework designed to streamline the process of creating and managing HTML forms. It offers a range of features that make form building more efficient and less error-prone. From generating form elements to handling validation and security, FormHelper provides a comprehensive solution for handling forms in CakePHP applications.
1. Getting Started: Setting Up CakePHP
Before we begin, make sure you have a CakePHP project set up. If you haven’t done this yet, you can follow the official CakePHP documentation to create a new project. Once you have your project ready, you can start using FormHelper to create dynamic forms.
2. Creating Form Elements
FormHelper simplifies the creation of form elements such as text inputs, checkboxes, radio buttons, and more. To create a form element using FormHelper, you’ll typically use the create() method followed by the specific form element methods. Here’s an example of how to create a simple text input field using FormHelper:
php // In your view file (e.g., src/Template/YourController/add.ctp) echo $this->Form->create(); echo $this->Form->text('username'); echo $this->Form->end('Submit');
In this example, Form->text(‘username’) generates an HTML text input element with the name attribute set to “username.”
3. Working with Form Options
FormHelper provides various options to customize the behavior and appearance of form elements. You can set options such as labels, default values, and CSS classes. Here’s how you can use options to create a text input with a label and default value:
php echo $this->Form->create(); echo $this->Form->text('email', ['label' => 'Email', 'default' => 'user@example.com']); echo $this->Form->end('Submit');
By providing options within the method call, you can control various aspects of the form element.
4. Handling Form Submissions
Handling form submissions is a crucial aspect of form development. FormHelper makes it easy to process form data and perform validation. After submitting a form, you can access the submitted data using the $this->request->getData() method. Here’s a basic example of handling form submission and validation:
php // In your controller public function add() { $entity = $this->YourModel->newEmptyEntity(); if ($this->request->is('post')) { $entity = $this->YourModel->patchEntity($entity, $this->request->getData()); if ($this->YourModel->save($entity)) { $this->Flash->success('Data saved successfully.'); return $this->redirect(['action' => 'index']); } $this->Flash->error('Data could not be saved.'); } $this->set(compact('entity')); }
In this example, we create a new entity, patch it with the form data, and attempt to save it. If the data validation and saving are successful, we show a success message; otherwise, we display an error message.
5. Implementing Dynamic Forms
One of the strengths of CakePHP FormHelper is its ability to generate dynamic form elements based on data. This is particularly useful when working with dynamic forms that require options to be populated from a database or another data source. Let’s say you want to create a dropdown select element with options from a related model. Here’s how you can achieve that:
php // In your controller public function add() { $entity = $this->YourModel->newEmptyEntity(); $relatedOptions = $this->YourRelatedModel->find('list')->toArray(); if ($this->request->is('post')) { // ... (same form submission handling as before) } $this->set(compact('entity', 'relatedOptions')); } php // In your view file (e.g., src/Template/YourController/add.ctp) echo $this->Form->create(); echo $this->Form->select('related_id', $relatedOptions, ['empty' => true]); echo $this->Form->end('Submit');
In this example, we retrieve a list of options from a related model (YourRelatedModel) and pass it to the select() method of FormHelper. The dynamic dropdown is generated with the retrieved options.
6. Validation and Security
FormHelper also integrates with CakePHP’s validation and security features. You can define validation rules in your model and use them to automatically validate form data. To display validation errors, CakePHP provides the error() method. Here’s an example of adding validation to a form element:
php // In your model public function validationDefault(Validator $validator) { $validator ->requirePresence('username') ->notEmptyString('username', 'Please enter a username.'); return $validator; } php // In your view file echo $this->Form->create(); echo $this->Form->text('username'); echo $this->Form->error('username'); echo $this->Form->end('Submit');
The requirePresence() and notEmptyString() methods define validation rules for the “username” field. The error() method displays any validation errors associated with the field.
Conclusion
In this tutorial, we’ve explored the CakePHP FormHelper and how it simplifies the process of creating dynamic forms in CakePHP applications. We’ve covered essential concepts such as creating form elements, using form options, handling form submissions, and implementing dynamic forms. With FormHelper’s powerful features, you can efficiently create interactive and user-friendly forms while taking advantage of CakePHP’s validation and security capabilities. By incorporating FormHelper into your development workflow, you can save time, reduce errors, and create seamless form experiences for your users.
Table of Contents
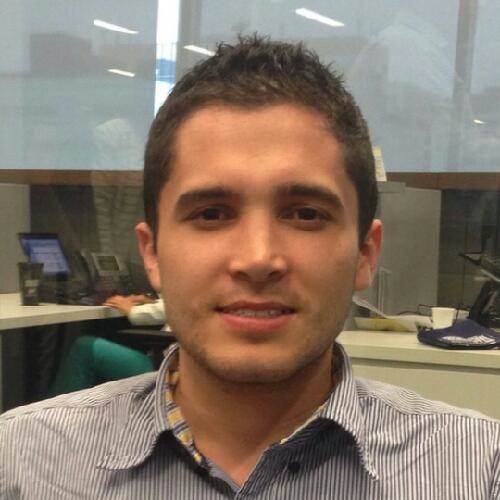
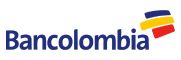