CakePHP and Vue.js: Creating Reactive Interfaces
In the ever-evolving landscape of web development, creating interactive and user-friendly interfaces has become paramount. The combination of backend frameworks like CakePHP with frontend libraries such as Vue.js can yield remarkable results. CakePHP offers a robust foundation for building server-side logic, while Vue.js empowers developers to create reactive and dynamic user interfaces. In this tutorial, we’ll delve into the integration of CakePHP and Vue.js, exploring how this duo can work seamlessly to create compelling web applications.
Table of Contents
1. Why CakePHP and Vue.js?
Before we dive into the technical details, let’s understand why CakePHP and Vue.js make an excellent combination for building reactive interfaces.
1.1. CakePHP: A Strong Backend Framework
CakePHP is a popular and powerful PHP framework that follows the convention-over-configuration principle. It provides a structured way to build web applications, making it easier to manage database interactions, routing, authentication, and more. Its robust architecture aids in creating scalable and maintainable backend code, enabling developers to focus on building features rather than dealing with repetitive tasks.
1.2. Vue.js: A Dynamic Frontend Library
Vue.js, on the other hand, is a progressive JavaScript library for building user interfaces. Its core library focuses on the view layer only, making it easy to integrate with other libraries or existing projects. Vue.js excels at creating reactive components, allowing developers to build dynamic interfaces that update in real-time as data changes. Its simplicity and flexibility make it an ideal choice for crafting modern frontend experiences.
2. Setting Up the Project
Before we can start integrating CakePHP and Vue.js, we need to set up our project environment. Make sure you have Composer and npm (Node Package Manager) installed on your system.
Step 1: CakePHP Installation and Configuration
Start by installing CakePHP using Composer. Open your terminal and navigate to the directory where you want to create your project. Run the following command:
bash composer create-project --prefer-dist cakephp/app my-vue-cake-app
Once the installation is complete, navigate to the config/app.php file and configure your database connection settings.
Step 2: Vue.js Installation
Navigate to your CakePHP project’s webroot directory and create a new directory for your Vue.js frontend. You can name it anything you prefer, such as vue-app. In your terminal, navigate to this new directory and initialize a new npm project:
bash mkdir vue-app cd vue-app npm init -y
This will create a package.json file in your vue-app directory.
3. Integrating Vue.js in CakePHP
With the project environment set up, it’s time to integrate Vue.js into our CakePHP application.
Step 1: Installing Vue.js
In your vue-app directory, install Vue.js and its dependencies using npm:
bash npm install vue
Step 2: Creating a Vue Component
Vue.js works by building components that encapsulate various parts of your user interface. Let’s create a simple Vue component. In the vue-app directory, create a new file named HelloVue.vue:
vue <template> <div> <h1>{{ greeting }}</h1> <p>{{ message }}</p> </div> </template> <script> export default { data() { return { greeting: 'Hello from Vue!', message: 'Welcome to our CakePHP-Vue.js app.' }; } }; </script>
Step 3: Building the Vue Instance
In the same directory, create a new file named main.js to initialize the Vue instance and mount it to an element in your CakePHP view:
javascript import Vue from 'vue'; import HelloVue from './HelloVue.vue'; new Vue({ render: h => h(HelloVue) }).$mount('#app');
Step 4: Displaying the Vue Component
Now, let’s display our Vue component in a CakePHP view. In your CakePHP project, navigate to src/Template/Pages/home.ctp and add the following code:
php <div id="app"></div> <script src="/vue-app/dist/build.js"></script>
4. Making Vue.js Reactive with CakePHP Data
A major advantage of using Vue.js is its reactivity – changes in data trigger immediate updates in the UI. To achieve this with CakePHP data, we’ll integrate Vue.js with CakePHP’s API endpoints.
Step 1: Creating an API Endpoint
In your CakePHP project, create an API endpoint to fetch data. For example, let’s create a simple endpoint to fetch a list of tasks. In src/Controller/TasksController.php:
php public function apiTasks() { $tasks = $this->Tasks->find('all'); $this->set([ 'tasks' => $tasks, '_serialize' => ['tasks'] ]); }
Step 2: Vue Component Modification
Modify the HelloVue.vue component to fetch data from the CakePHP API endpoint using the axios library:
vue <template> <div> <h1>{{ greeting }}</h1> <ul> <li v-for="task in tasks" :key="task.id">{{ task.title }}</li> </ul> </div> </template> <script> import axios from 'axios'; export default { data() { return { greeting: 'Hello from Vue!', tasks: [] }; }, mounted() { this.fetchTasks(); }, methods: { fetchTasks() { axios.get('/tasks/apiTasks') .then(response => { this.tasks = response.data.tasks; }) .catch(error => { console.error(error); }); } } }; </script>
Step 3: Displaying CakePHP Data
Update your home.ctp file to display the modified Vue component:
php <div id="app"></div> <script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script> <script src="/vue-app/dist/build.js"></script>
Conclusion
By combining the backend strength of CakePHP with the frontend interactivity of Vue.js, you can create powerful and reactive web interfaces. This dynamic duo enables you to build applications that not only handle complex server-side logic but also provide users with seamless and real-time interactive experiences. Whether you’re developing a content management system, an e-commerce platform, or any other web application, the CakePHP-Vue.js combination is a potent choice to consider.
In this tutorial, we’ve explored the process of setting up a project with CakePHP and Vue.js, integrating Vue.js components, and making them reactive with data from CakePHP API endpoints. This is just the beginning of the possibilities you can explore with these technologies. As you delve deeper, you’ll discover even more ways to enhance user experiences and streamline development workflows. Happy coding!
Table of Contents
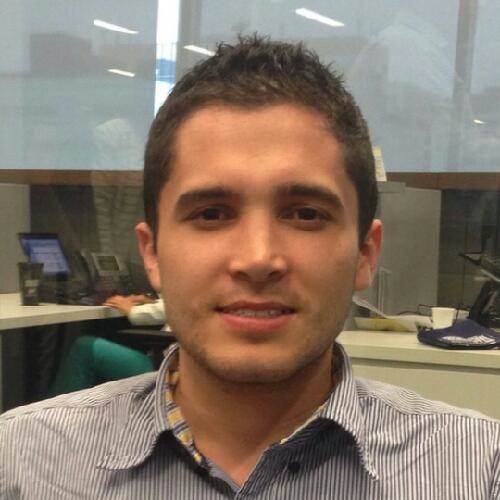
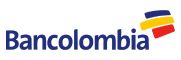