Creating Beautiful and Responsive Layouts with CakePHP
In today’s web development landscape, creating visually appealing and responsive layouts is essential to attract and retain users. CakePHP, a popular PHP framework, offers a powerful and flexible environment to design beautiful and mobile-friendly interfaces. In this blog, we will dive into the process of creating stunning and responsive layouts with CakePHP, exploring key techniques, best practices, and code samples along the way.
Table of Contents
1. Understanding the Importance of Responsive Layouts
Before we delve into the specifics of using CakePHP, let’s discuss the significance of responsive layouts. With the increasing use of mobile devices, having a responsive website is crucial to provide an optimal user experience across various screen sizes and resolutions. Responsive layouts adapt seamlessly to different devices, ensuring that users can access content and interact with the website efficiently, whether they are using a desktop computer, tablet, or smartphone.
A responsive layout not only improves user experience but also positively impacts search engine rankings. Search engines prioritize mobile-friendly websites in their results, making responsive design an essential factor in SEO.
2. Setting Up CakePHP
To get started with CakePHP and follow along with the code examples in this blog, make sure you have CakePHP installed on your local development environment. You can download the latest version from the official CakePHP website (https://cakephp.org/). Once downloaded, follow the installation instructions to set up the framework successfully.
2.1. Building a Basic Layout
In CakePHP, layouts are used to define the structure that wraps around your views. They act as the common template for your web application, ensuring consistent elements like headers, footers, and navigation menus across all pages. The default layout file is usually located at src/Template/Layout/default.ctp.
Let’s start by creating a basic layout using CakePHP’s default layout file. Open default.ctp and add the following code:
php <!DOCTYPE html> <html> <head> <?= $this->Html->charset() ?> <title><?= $this->fetch('title') ?></title> <?= $this->Html->meta('viewport', 'width=device-width, initial-scale=1') ?> <?= $this->Html->css('styles.css') ?> </head> <body> <header> <!-- Your header content here --> </header> <main> <?= $this->Flash->render() ?> <?= $this->fetch('content') ?> </main> <footer> <!-- Your footer content here --> </footer> </body> </html>
In the above code, we have a basic HTML structure with a header, main content area, and a footer. We’ve also included the necessary CakePHP functions to render the title, meta viewport tag, and load a CSS file called styles.css.
2.2. Implementing Responsive CSS
To make our layout responsive, we need to add responsive CSS styles. CSS frameworks like Bootstrap and Foundation are popular choices for building responsive websites, but you can also create custom styles based on your specific design requirements.
In this example, let’s use Bootstrap to make the layout responsive. First, download Bootstrap or include it using a CDN in your CakePHP project. Next, update the styles.css file and add the following code:
css /* styles.css */ /* Add some basic styles for the header and footer */ header, footer { background-color: #333; color: #fff; padding: 10px; text-align: center; } /* Style the main content area */ main { padding: 20px; } /* Make the layout responsive using Bootstrap classes */ @media (max-width: 768px) { header, footer { padding: 5px; } main { padding: 10px; } }
In this example, we have added basic styles for the header and footer elements. The @media query targets screen widths up to 768px, applying specific styles to make the layout more compact and user-friendly on smaller devices.
2.3. Creating Dynamic Content with Views
With our responsive layout in place, let’s focus on creating dynamic content using views. Views in CakePHP are responsible for presenting data to the users. They contain HTML and display data received from the controller.
To create a view, navigate to the src/Template directory and create a new folder for the corresponding controller. For example, if you have a controller called PagesController, create a new folder named Pages. Inside this folder, create a file named home.ctp with the following code:
php <!-- src/Template/Pages/home.ctp --> <section> <h1>Welcome to CakePHP Layouts</h1> <p> CakePHP is a powerful framework that allows you to build web applications quickly and efficiently. </p> <p> With the knowledge you've gained from this blog, you can now create beautiful and responsive layouts to enhance the user experience of your CakePHP applications. </p> </section>
In this example, we’ve created a simple view for the homepage. The view contains an introductory message about CakePHP and the advantages of using it for building web applications.
2.4. Dynamic Layouts with Elements
CakePHP also allows you to create reusable view components called elements. Elements are particularly useful for elements that are common across multiple views, such as navigation menus or call-to-action buttons.
Let’s create a dynamic navigation menu element. In the src/Template/Element directory, create a file named navigation.ctp with the following code:
php <!-- src/Template/Element/navigation.ctp --> <nav> <ul> <li><?= $this->Html->link('Home', ['controller' => 'Pages', 'action' => 'display', 'home']) ?></li> <li><?= $this->Html->link('About', ['controller' => 'Pages', 'action' => 'display', 'about']) ?></li> <li><?= $this->Html->link('Contact', ['controller' => 'Pages', 'action' => 'display', 'contact']) ?></li> </ul> </nav>
In this example, we’ve created a navigation menu with links to the home, about, and contact pages. We are using the Html->link() function to generate the links dynamically, based on the controller and action specified.
To include this navigation menu in our layout, open default.ctp, and add the following code:
php <!DOCTYPE html> <html> <head> <!-- Head content --> </head> <body> <header> <?= $this->element('navigation') ?> </header> <main> <?= $this->Flash->render() ?> <?= $this->fetch('content') ?> </main> <footer> <!-- Footer content --> </footer> </body> </html>
In the header section of the layout, we are including the navigation element using $this->element(‘navigation’). This will dynamically render the navigation menu across all pages that use this layout.
Conclusion
In this blog, we explored the process of creating beautiful and responsive layouts with CakePHP. We learned the importance of responsive design in modern web development and how CakePHP can help achieve this goal. Starting with a basic layout, we integrated Bootstrap to create a responsive design and also created dynamic content using views and elements.
With the knowledge gained from this blog, you are well-equipped to build stunning and user-friendly interfaces for your CakePHP web applications. Remember to keep experimenting and refining your layouts to provide the best possible experience for your users, regardless of the devices they use to access your site. Happy coding!
Table of Contents
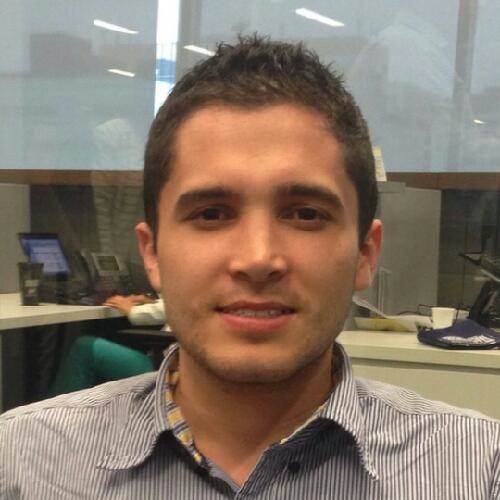
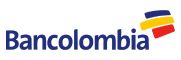