Creating Custom CakePHP Exceptions and Error Handling
When developing web applications, error handling is an essential aspect of ensuring a smooth user experience and maintaining the robustness of your code. CakePHP, a popular PHP framework, offers powerful tools for managing exceptions and errors, allowing you to create custom exceptions that provide meaningful feedback to users and developers alike. In this article, we’ll delve into the world of custom CakePHP exceptions and explore how to handle errors effectively.
Table of Contents
1. Understanding the Importance of Custom Exceptions
Exception handling is the process of dealing with unexpected situations in your code, such as database connection failures, missing files, or incorrect user input. By default, PHP and frameworks like CakePHP provide a set of predefined exceptions that cover common scenarios. However, using custom exceptions tailored to your application’s specific context can significantly improve error messages and simplify debugging.
2. Benefits of Custom Exceptions
- Clear Feedback: Custom exceptions allow you to provide more specific and informative error messages to users, helping them understand what went wrong and how to address the issue.
- Maintenance: With custom exceptions, you can centralize error handling logic, making it easier to maintain and update error-handling mechanisms across your application.
- Debugging: Custom exceptions can include additional contextual information, such as the values of variables or the state of the application when the exception occurred. This information is invaluable for debugging and resolving issues quickly.
3. Creating Custom Exceptions in CakePHP
CakePHP provides a straightforward way to create custom exceptions that seamlessly integrate with its existing error handling infrastructure. Let’s walk through the process step by step.
Step 1: Extending the Base Exception Class
To create a custom exception in CakePHP, you need to extend the base Cake\Core\Exception\Exception class. This ensures that your custom exception inherits all the necessary error-handling features provided by the framework.
php // src/Exception/CustomException.php namespace App\Exception; use Cake\Core\Exception\Exception; class CustomException extends Exception { }
In this example, we’ve created a CustomException class that extends Exception.
Step 2: Adding Contextual Information
One of the advantages of custom exceptions is the ability to include additional information about the error. You can achieve this by adding constructor parameters to your custom exception class. For instance, let’s say you want to create an exception for when a user attempts to access a resource they don’t have permission for:
php // src/Exception/PermissionException.php namespace App\Exception; use Cake\Core\Exception\Exception; class PermissionException extends Exception { protected $permissionResource; public function __construct($message, $code = 403, $resource = null) { parent::__construct($message, $code); $this->permissionResource = $resource; } public function getPermissionResource() { return $this->permissionResource; } }
In this example, the PermissionException class takes an additional parameter $resource in its constructor to store the resource that the user was trying to access without permission. The getPermissionResource method allows retrieving this resource.
Step 3: Throwing Custom Exceptions
Once your custom exception class is defined, you can use it throughout your application to handle specific scenarios. In a controller or service, you can throw your custom exception when a permission check fails:
php // src/Controller/ArticlesController.php namespace App\Controller; use App\Exception\PermissionException; use Cake\Controller\Controller; class ArticlesController extends Controller { public function view($id) { // Check user's permission to view the article if (!$this->checkPermission($id)) { throw new PermissionException('You do not have permission to view this article.', 403, 'articles'); } // Rest of the view action logic } // Other methods }
In this example, if the permission check fails, a PermissionException is thrown with a meaningful error message and the resource (‘articles’) that the user was trying to access.
Step 4: Handling Custom Exceptions
To ensure your custom exceptions are properly handled, you need to configure the error handling in your CakePHP application. CakePHP provides an ErrorController that you can extend to define how different types of exceptions should be handled.
php // src/Controller/ErrorController.php namespace App\Controller; use App\Exception\PermissionException; use Cake\Controller\Controller; use Cake\Http\Exception\HttpException; class ErrorController extends Controller { public function initialize(): void { parent::initialize(); $this->loadComponent('RequestHandler'); } public function appException($exception) { if ($exception instanceof PermissionException) { $this->response = $this->response->withStatus(403); $this->set('message', $exception->getMessage()); $this->set('resource', $exception->getPermissionResource()); } else { // Handle other types of exceptions } } public function httpException($exception) { // Handle HTTP exceptions } // Other methods }
In this example, the ErrorController extends the base controller and overrides the appException method to handle PermissionException. It sets the response status, error message, and resource information.
Conclusion
Custom exceptions are a powerful tool in your error-handling toolkit, enabling you to provide better user feedback and streamline the debugging process. By following CakePHP’s structured approach to creating and handling custom exceptions, you can enhance the reliability and user experience of your web applications. Remember that effective error handling isn’t just about managing technical hiccups; it’s also about creating a user-friendly environment that gracefully handles unexpected situations.
Incorporating custom exceptions into your CakePHP application might require a bit of initial setup and configuration, but the long-term benefits in terms of maintainability and user satisfaction make it well worth the effort. So, go ahead and start implementing custom exceptions in your CakePHP projects, and take your error handling to the next level.
Table of Contents
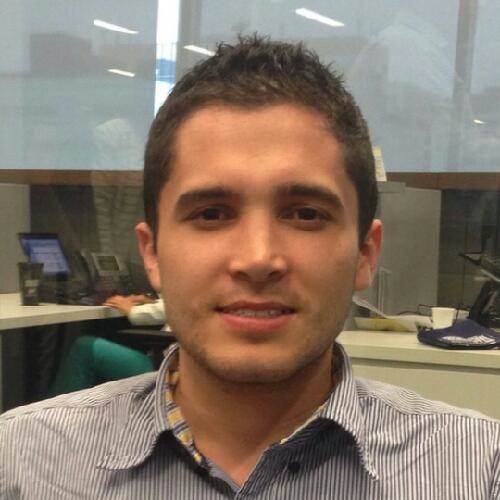
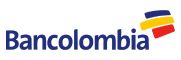