Creating Custom CakePHP Helpers for View Rendering
CakePHP is a powerful PHP framework that allows developers to build web applications rapidly and efficiently. One of its core features is the separation of concerns, which encourages a clean division between the presentation layer (views) and the business logic (controllers). To further enhance the reusability and maintainability of your code, CakePHP provides helpers—a set of utility classes that assist in generating HTML and other output. In this blog, we will dive into the world of CakePHP helpers and explore how to create custom helpers to handle complex view rendering tasks with ease.
1. Understanding CakePHP Helpers:
1.1 What are Helpers?
Helpers are utility classes in CakePHP that allow developers to package and reuse common view-related logic, such as generating HTML tags, form elements, pagination links, and more. They are intended to keep the view templates clean and free from complex PHP code, thereby improving readability and maintainability.
1.2 Predefined Helpers in CakePHP:
CakePHP ships with several built-in helpers, including:
- HtmlHelper: For generating HTML tags, links, and image tags.
- FormHelper: For creating HTML forms and form elements.
- PaginatorHelper: For handling pagination of records in views.
- SessionHelper: For managing session data and flash messages.
- TimeHelper: For formatting and displaying dates and times.
- NumberHelper: For formatting and displaying numbers.
Now, let’s explore the need for custom helpers and why they are essential in certain scenarios.
2. The Need for Custom Helpers:
2.1 Encapsulation of Reusable Logic:
As your CakePHP application grows, you might find yourself repeating certain view-related logic across different views. Custom helpers provide an opportunity to encapsulate this logic within helper methods, allowing you to reuse it in various parts of your application without duplicating code.
2.2 Handling Complex View Rendering:
In some cases, the standard CakePHP helpers may not entirely fulfill your view rendering requirements. For instance, if you need to generate complex HTML structures, interact with external APIs, or perform custom data processing before rendering the view, a custom helper becomes the ideal solution to handle these complex tasks gracefully.
3. Creating Custom Helpers:
3.1 Structure and Naming Conventions:
Custom helpers in CakePHP should be placed inside the src/View/Helper directory of your application. The naming convention for a custom helper is as follows: <Name>Helper.php. For example, a helper named MyCustomHelper should be defined in a file named MyCustomHelper.php.
3.2 Helper Class Basics:
A custom helper class should extend the Helper class provided by CakePHP. This base class includes essential functionalities and magic methods that facilitate rendering and integration with the view layer. To access the base helper’s features, you must call parent::__construct($View, $settings); within your custom helper’s constructor.
3.3 Registering Custom Helpers:
Before you can use a custom helper, you need to register it with the view. This can be achieved in multiple ways:
- In the Controller: Inside a specific controller where you intend to use the helper, add the following line in the controller’s initialize() method:
php $this->loadHelper('MyCustom');
- In AppView: If you want to use the custom helper throughout your application, register it in src/View/AppView.php:
php public function initialize(): void { parent::initialize(); $this->loadHelper('MyCustom'); }
With the basics covered, let’s move on to writing a custom helper and delve into an example scenario where we’ll build a PaginatorHelper.
4. Writing a Custom Helper:
4.1 Implementing Helper Methods:
Let’s start by creating our custom PaginatorHelper class. Inside the src/View/Helper directory, create a new file named PaginatorHelper.php and define the class as follows:
php // src/View/Helper/PaginatorHelper.php namespace App\View\Helper; use Cake\View\Helper; class PaginatorHelper extends Helper { public function pagination($totalPages, $currentPage) { // Implement your custom pagination logic here // Return the HTML for pagination links } }
In this example, our PaginatorHelper will have a method called pagination(), which will generate pagination links based on the total number of pages and the current page.
4.2 Using HTML and Form Helpers within Custom Helpers:
Since custom helpers are PHP classes and not view templates, you cannot directly use the built-in HtmlHelper and FormHelper methods within them. To access these functionalities, you can utilize the $this->_View property within your custom helper. This property provides an instance of the View class, which has the Html and Form helpers available.
For example, if you want to generate a link within the pagination() method, you can do so as follows:
php public function pagination($totalPages, $currentPage) { // Implement your custom pagination logic here // Return the HTML for pagination links $link = $this->_View->Html->link('Next', ['controller' => 'Posts', 'action' => 'index', 'page' => $currentPage + 1]); return $link; }
Now that we understand how to write a custom helper, let’s proceed to an example scenario of building a PaginatorHelper.
5. An Example Scenario: Building a Paginator Helper:
5.1 Understanding the Requirements:
Our goal is to create a custom PaginatorHelper that generates pagination links for a blog application. The helper will take the total number of pages and the current page as input and return the HTML for the pagination links, including links to the previous and next pages.
5.2 Creating the Paginator Helper:
Let’s implement the PaginatorHelper with the required functionality:
php // src/View/Helper/PaginatorHelper.php namespace App\View\Helper; use Cake\View\Helper; class PaginatorHelper extends Helper { public function pagination($totalPages, $currentPage) { if ($currentPage <= 1) { $prevLink = '<span class="disabled">Previous</span>'; } else { $prevLink = $this->_View->Html->link('Previous', ['controller' => 'Posts', 'action' => 'index', 'page' => $currentPage - 1]); } if ($currentPage >= $totalPages) { $nextLink = '<span class="disabled">Next</span>'; } else { $nextLink = $this->_View->Html->link('Next', ['controller' => 'Posts', 'action' => 'index', 'page' => $currentPage + 1]); } $pagination = '<div class="pagination">' . $prevLink . ' | ' . $nextLink . '</div>'; return $pagination; } }
In this example, we first check if the current page is the first or last page and appropriately disable the “Previous” or “Next” links. We then generate the links using the HtmlHelper and return the formatted pagination as a string.
5.3 Utilizing the Paginator Helper in the View:
Now, let’s use our custom PaginatorHelper in a view template, such as the index.ctp template for listing blog posts:
php // src/Template/Posts/index.ctp <h1>Blog Posts</h1> <!-- Display blog posts here --> <?= $this->Paginator->pagination($totalPages, $currentPage) ?>
By calling $this->Paginator->pagination($totalPages, $currentPage) in the view template, we render the pagination links using our custom helper.
Conclusion
Custom helpers are a powerful tool in CakePHP that allows you to extend the view rendering capabilities of your application. By encapsulating reusable view logic and handling complex rendering tasks, custom helpers promote code reusability, readability, and maintainability.
In this blog, we explored the fundamentals of CakePHP helpers, the need for custom helpers, and the process of creating and utilizing custom helpers. Additionally, we implemented a practical example of building a PaginatorHelper for generating pagination links in a blog application.
As you continue to develop your CakePHP applications, remember to leverage custom helpers whenever you encounter repetitive or intricate view rendering tasks. With the right helpers in your toolkit, you can make your views cleaner, more efficient, and easier to maintain, ultimately leading to a more robust and user-friendly web application.
Table of Contents
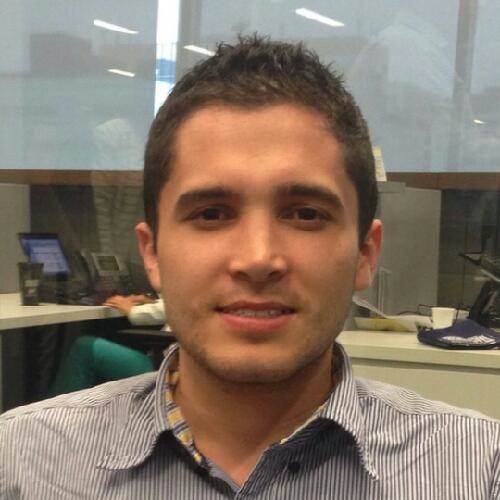
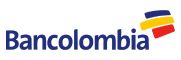