Creating Custom CakePHP Route Classes
CakePHP is a popular PHP framework known for its flexibility and ease of use when it comes to building web applications. One of its key features is its powerful routing system, which allows you to define how URLs are mapped to controller actions. While CakePHP provides a robust set of default routing options, there are times when you may need to create custom route classes to handle complex routing requirements.
Table of Contents
In this blog post, we’ll explore the concept of creating custom CakePHP route classes. We’ll cover everything from understanding the basics of routing in CakePHP to building and using custom route classes to handle unique URL patterns and requirements. So, let’s dive in!
1. Understanding CakePHP Routing Basics
Before we delve into creating custom route classes, it’s essential to have a solid understanding of how routing works in CakePHP. At its core, routing in CakePHP is about defining rules that map URLs to controller actions. The routing process generally involves the following steps:
1.1. Parsing the URL
When a user makes a request to your CakePHP application, the framework first needs to parse the incoming URL to determine which controller and action should handle the request. This is where the routing system comes into play.
1.2. Matching Routes
CakePHP’s default router tries to match the parsed URL against the defined routes in your application. These routes are usually specified in the routes.php file located in the config directory of your CakePHP project.
1.3. Dispatching to Controller Actions
Once a matching route is found, CakePHP dispatches the request to the appropriate controller action, passing along any additional parameters extracted from the URL.
1.4. Generating URLs
On the reverse side, CakePHP can also generate URLs based on the defined routes, making it easy to create links and redirects within your application.
Now that you have a basic understanding of CakePHP routing, let’s move on to creating custom route classes.
2. Why Create Custom Route Classes?
While CakePHP’s default routing system is powerful and covers many common use cases, there are scenarios where you may need more flexibility and control over your application’s routing. Here are some common reasons to create custom route classes:
2.1. Handling Complex URL Patterns
You might have unique URL patterns in your application that cannot be easily expressed using the default route configuration. Custom route classes allow you to define complex routing rules.
2.2. Implementing Custom Routing Logic
In some cases, you may need to implement custom logic to determine which controller and action should handle a specific URL. Custom route classes enable you to do this.
2.3. Centralizing Route Logic
If you find yourself repeating the same routing logic across multiple routes, creating a custom route class can help centralize that logic, making your application more maintainable.
2.4. Enhancing Reusability
Custom route classes can be designed to be reusable across different parts of your application or even in multiple projects, promoting code reusability.
Now that you know why custom route classes can be beneficial, let’s walk through the process of creating one.
3. Creating a Custom CakePHP Route Class
Creating a custom route class in CakePHP involves a few key steps. We’ll break down each step and provide code examples to illustrate the process.
3.1. Extend the Cake\Routing\Route\Route Class
To create a custom route class, you need to create a new PHP class that extends CakePHP’s Cake\Routing\Route\Route class. This base class provides the structure and methods necessary for a route class to work within the CakePHP routing system.
Here’s an example of a basic custom route class:
php // src/Routing/Route/CustomRoute.php namespace App\Routing\Route; use Cake\Routing\Route\Route; class CustomRoute extends Route { // Your custom routing logic goes here }
3.2. Implement the match() Method
The heart of any route class is the match() method. This method is responsible for determining if the route matches the given URL and, if it does, extracting the relevant route parameters.
Here’s a simplified example of a match() method:
php public function match(array $url, array $context = []) { // Your matching logic here // Return an array of route parameters or false if no match is found }
3.3. Implement the parse() Method
The parse() method is responsible for generating a URL based on the provided route parameters. It’s the reverse of the match() method.
Here’s a basic example of a parse() method:
php public function parse($url, $context) { // Your URL generation logic here // Return the generated URL as a string }
3.4. Register Your Custom Route Class
To make your custom route class available to the CakePHP routing system, you need to register it in your application’s config/routes.php file. You can do this using the Router::addRoute() method:
php // config/routes.php use Cake\Routing\Router; Router::addRoute( 'custom', ['_name' => 'custom', '_method' => ['GET'], 'routeClass' => 'App\Routing\Route\CustomRoute'] );
In this example, we’ve added a custom route named ‘custom’ that uses our CustomRoute class. You can customize the route name, HTTP methods, and other options as needed.
3.5. Define Your Custom Routing Logic
Now that you have the structure of your custom route class in place and have registered it with the CakePHP routing system, you can define your custom routing logic in the match() and parse() methods.
Let’s look at a real-world example to see how this all comes together.
4. Example: Creating a Slug Route
Imagine you have a blog application where each blog post is identified by a unique slug in the URL, like this: example.com/posts/my-awesome-blog-post. To achieve this, you can create a custom route class that matches and parses these URLs.
php // src/Routing/Route/SlugRoute.php namespace App\Routing\Route; use Cake\Routing\Route\Route; class SlugRoute extends Route { public function match(array $url, array $context = []) { // Match URLs like '/posts/my-awesome-blog-post' if (isset($url['controller']) && $url['controller'] === 'Posts') { // Extract the slug and set it as a route parameter $slug = $url['action']; return ['slug' => $slug]; } // No match return false; } public function parse($url, $context) { // Generate URLs based on the 'slug' route parameter if (isset($url['slug'])) { return ['controller' => 'Posts', 'action' => $url['slug']]; } // No match return false; } }
With this custom SlugRoute class, you can now define routes in your config/routes.php file that use this route class to handle blog post URLs.
php // config/routes.php use Cake\Routing\Router; Router::addRoute( 'blog-post', ['_name' => 'blog-post', '_method' => ['GET'], 'routeClass' => 'App\Routing\Route\SlugRoute'] );
Now, you can generate and match URLs like example.com/posts/my-awesome-blog-post using the blog-post route.
Conclusion
Creating custom route classes in CakePHP gives you the flexibility and control needed to handle complex routing requirements in your web applications. By following the steps outlined in this blog post, you can define your own routing logic and make your application’s URLs more user-friendly and SEO-friendly.
Whether you need to implement custom URL patterns, centralize route logic, or enhance code reusability, custom route classes are a powerful tool in your CakePHP development toolkit. So, start exploring the world of custom routing and unlock new possibilities for your web applications!
Table of Contents
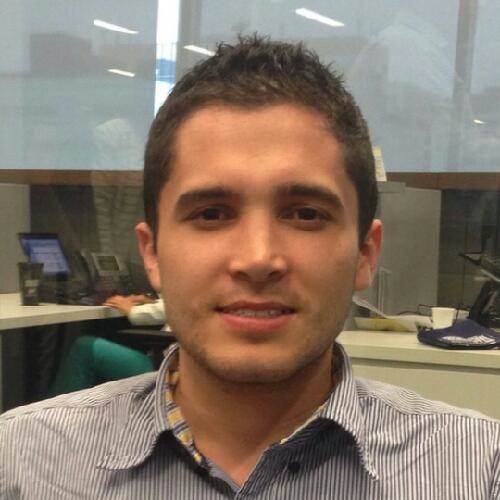
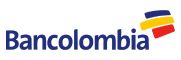