Creating Custom CakePHP Shell Commands for Task Automation
In today’s fast-paced development environment, automation plays a crucial role in increasing productivity and efficiency. CakePHP, a popular PHP framework, offers a powerful shell feature that allows developers to automate repetitive tasks, perform data migrations, and more. In this blog post, we will explore how to create custom CakePHP shell commands for task automation, enabling you to streamline your development workflow and save valuable time.
Table of Contents
1. Introduction to Shell Commands
1.1. What are Shell Commands?
In the context of CakePHP, shell commands are PHP scripts that can be executed through the command line. These scripts are a powerful way to interact with your application and perform various tasks, such as generating code, running scheduled tasks, or performing data migrations. By using shell commands, developers can automate repetitive tasks, making the development process smoother and more efficient.
1.2. Benefits of Custom Shell Commands
Creating custom shell commands for your CakePHP application offers several advantages:
- Task Automation: Automate repetitive tasks to save time and reduce human errors.
- Customization: Tailor the commands to suit your specific project requirements.
- Complex Operations: Perform complex operations that may not be practical through web requests.
- Background Processing: Execute tasks in the background, freeing up web server resources.
- Increased Efficiency: Improve developer productivity and speed up the development process.
2. Setting Up a CakePHP Environment
Before we dive into creating custom shell commands, ensure you have a working CakePHP environment set up on your system. Follow these steps:
- Install Composer: If you haven’t already, install Composer to manage CakePHP dependencies.
- Create a New CakePHP Project: Open a terminal and run the following command to create a new CakePHP project (replace my_project with your desired project name):
bash composer create-project --prefer-dist cakephp/app my_project
3. Navigate to the Project Directory: Change to the newly created project directory:
bash cd my_project
With the environment set up, we’re ready to create our first custom shell command.
3. Creating Your First Custom Shell Command
3.1. Creating the Shell File
In CakePHP, all shell commands are stored in the src/Shell directory. To create our custom shell command, let’s generate a new file named ExampleShell.php:
bash touch src/Shell/ExampleShell.php
Open the newly created file in your preferred text editor.
3.2. Defining the Command
A CakePHP shell command is a PHP class that extends the Shell class. Let’s define our basic ExampleShell class:
php // src/Shell/ExampleShell.php namespace App\Shell; use Cake\Console\Shell; class ExampleShell extends Shell { public function main() { $this->out('Hello, this is your custom CakePHP shell command!'); } }
The main method is the default method called when you run a shell command without specifying any arguments. In our case, it outputs a simple greeting message.
3.3. Executing the Command
To test our custom shell command, run the following command from the project root:
bash bin/cake example
You should see the following output:
kotlin Hello, this is your custom CakePHP shell command!
Congratulations! You’ve created and executed your first custom CakePHP shell command.
4. Command Arguments and Options
4.1. Adding Arguments
Shell commands often require additional information to perform specific tasks. Arguments are one way to pass this information. Let’s modify our ExampleShell to accept an argument:
php // src/Shell/ExampleShell.php // ... (previous code) public function main($name = null) { if ($name) { $this->out('Hello, ' . $name . '!'); } else { $this->out('Hello, this is your custom CakePHP shell command!'); } }
In this updated version, we added a $name parameter to the main method. The command will now greet the user with their name if the argument is provided.
To test the argument functionality, run the following command:
bash bin/cake example John
You should see the following output:
Hello, John!
If you run the command without providing an argument, it will revert to the default greeting.
4.2. Implementing Options
In addition to arguments, shell commands can accept options, which are typically flags that modify the command’s behavior. To add an option to our ExampleShell, follow these steps:
php // src/Shell/ExampleShell.php // ... (previous code) public function getOptionParser() { $parser = parent::getOptionParser(); $parser->addOption('shout', [ 'short' => 's', 'help' => 'Shout the greeting message', 'boolean' => true, ]); return $parser; } public function main($name = null) { if ($name) { $greeting = 'Hello, ' . $name . '!'; } else { $greeting = 'Hello, this is your custom CakePHP shell command!'; } if ($this->params['shout']) { $greeting = strtoupper($greeting); } $this->out($greeting); }
In the updated code, we have added an option –shout (or -s for short). If the –shout option is present when executing the command, the greeting message will be displayed in uppercase.
To test the option functionality, run the following commands:
bash bin/cake example --shout John
Output:
HELLO, JOHN!
bash bin/cake example John
Output:
Hello, John!
bash bin/cake example
Output:
kotlin Hello, this is your custom CakePHP shell command!
5. Interactive Shell Commands
5.1. Requesting User Input
In some cases, you may need to interactively request information from the user during command execution. CakePHP provides a simple method for handling user input using the in() method. Let’s modify our ExampleShell to ask for the user’s name:
php // src/Shell/ExampleShell.php // ... (previous code) public function main() { $name = $this->in('Please enter your name:'); $this->out('Hello, ' . $name . '!'); }
5.2. Handling User Responses
When you run the command now, it will prompt you to enter your name:
bash bin/cake example
Output:
yaml Please enter your name: John Hello, John!
The in() method allows you to ask questions and receive responses from the user during command execution. This is particularly useful for commands that require dynamic or user-specific information.
6. Running Shell Commands in Background
By default, shell commands run synchronously, blocking the command line until they complete. However, there are situations where you may want to run commands asynchronously in the background. To achieve this, you can use the exec() function in PHP. Let’s see how we can run a CakePHP shell command in the background:
php // src/Shell/ExampleShell.php // ... (previous code) public function main() { $this->out('Starting the background process...'); $command = 'nohup bin/cake example John > /dev/null 2>&1 & echo $!'; $pid = exec($command); $this->out('Background process started with PID: ' . $pid); }
In this example, we use the nohup command, which allows the shell command to keep running even if the terminal session is closed. The output is redirected to /dev/null to discard any output, and & is used to run the command in the background.
Note that $pid stores the process ID of the background task, allowing you to track and manage it later if needed.
To test the background execution, run the following command:
bash bin/cake example
Output:
arduino Starting the background process... Background process started with PID: 12345
Replace 12345 with the actual process ID of your background task.
7. Best Practices for Custom Shell Commands
When creating custom shell commands, keep the following best practices in mind:
7.1. Code Reusability
If you find yourself performing similar tasks in multiple shell commands, consider creating helper methods or abstract classes to encapsulate common functionality. This practice promotes code reusability and simplifies maintenance.
7.2. Proper Error Handling
Always implement proper error handling in your shell commands. Handle exceptions gracefully, log errors, and provide meaningful error messages to users when something goes wrong.
Conclusion
In this blog post, we explored the world of custom CakePHP shell commands for task automation. We learned how to set up a CakePHP environment, create custom shell commands, add arguments and options, request user input, and run commands in the background. By leveraging shell commands, you can significantly enhance your CakePHP application’s productivity and efficiency, automating repetitive tasks and focusing on the core aspects of development.
Automating tasks through custom shell commands is a valuable skill that will save you time and effort throughout your development journey. Whether you’re managing database migrations, generating code, or running scheduled tasks, custom shell commands will undoubtedly become an indispensable part of your CakePHP toolkit.
Now it’s time to put your newfound knowledge into practice and start automating your CakePHP applications with custom shell commands. Happy coding!
Table of Contents
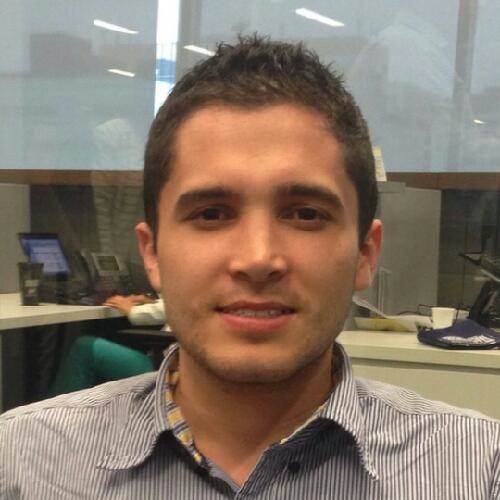
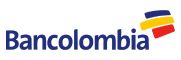