Creating Custom CakePHP Validators for Data Validation
When developing web applications, data validation is a crucial aspect to ensure the integrity and reliability of the information being processed. CakePHP, a popular PHP framework, offers a wide range of built-in validation rules. However, there might be scenarios where these default rules don’t cover your specific requirements. In such cases, creating custom validators in CakePHP can be a powerful solution. In this tutorial, we’ll walk you through the process of creating custom validators step by step, complete with code samples and examples.
Table of Contents
1. Why Custom Validators?
CakePHP comes with a comprehensive set of built-in validation rules that cater to common data validation needs. These built-in rules cover scenarios such as required fields, email validation, numeric values, and more. However, every application is unique, and there might be instances where you need to validate data according to specific business logic or complex criteria.
Creating custom validators allows you to tailor the validation process to your application’s needs. Whether you’re dealing with unconventional data formats, intricate validation logic, or specialized input requirements, custom validators can handle it all.
2. The Basics of Validators in CakePHP
Before diving into creating custom validators, let’s have a quick refresher on how validators work in CakePHP. Validators are classes responsible for defining validation rules for specific fields in your models. These rules dictate how the data entered into those fields should be validated.
In CakePHP, validators are typically defined in the validationDefault method of your model class. This method is automatically called by CakePHP during the validation process. Each validation rule is associated with a field name and consists of one or more validation checks.
3. Built-in Validation Example
Let’s start with a simple example of using a built-in validation rule in CakePHP. Consider a scenario where you want to validate a user’s email address using the built-in email rule:
php // src/Model/Table/UsersTable.php namespace App\Model\Table; use Cake\ORM\Table; class UsersTable extends Table { public function validationDefault(Validator $validator) { $validator ->email('email', 'Please enter a valid email address.'); return $validator; } }
In this example, we’re using the email validation rule to ensure that the email field contains a valid email address format.
4. Creating Custom Validators
Now, let’s move on to creating custom validators. The process involves creating a new validator class and defining your custom validation logic within it. Follow these steps to create a custom validator in CakePHP:
Step 1: Create the Validator Class
Start by creating a new PHP file for your custom validator. The naming convention for validator classes in CakePHP follows the format CustomRuleValidator.php.
php // src/Validation/CustomRuleValidator.php namespace App\Validation; use Cake\Validation\Validator; class CustomRuleValidator extends Validator { }
Step 2: Define the Validation Logic
Within your custom validator class, you can define your validation logic by creating methods that represent your custom rules. Each validation method should accept at least two arguments: the field being validated and an array of options. The options array can be used to pass additional parameters to your validation rules.
php // src/Validation/CustomRuleValidator.php namespace App\Validation; use Cake\Validation\Validator; class CustomRuleValidator extends Validator { public function isValidUsername($value, array $context) { // Custom validation logic for a valid username // Return true if validation passes, false otherwise } }
Step 3: Add Error Messages
To provide meaningful error messages when validation fails, you can use the addError() method within your custom validation methods.
php // src/Validation/CustomRuleValidator.php namespace App\Validation; use Cake\Validation\Validator; class CustomRuleValidator extends Validator { public function isValidUsername($value, array $context) { if (/* validation fails */) { $this->addError('username', 'The username is not valid.'); return false; } return true; } }
Step 4: Using the Custom Validator
Once you’ve defined your custom validator, you can use it in your model’s validationDefault method just like built-in validation rules.
php // src/Model/Table/UsersTable.php namespace App\Model\Table; use Cake\ORM\Table; class UsersTable extends Table { public function validationDefault(Validator $validator) { $validator ->add('username', 'custom', [ 'rule' => 'isValidUsername', 'message' => 'This username is not allowed.' ]); return $validator; } }
In this example, we’re adding a custom validation rule named ‘custom’ to the username field. The ‘rule’ option points to the isValidUsername method in our custom validator class.
Conclusion
Custom validators in CakePHP provide you with the flexibility to validate data according to your application’s unique requirements. By following the steps outlined in this tutorial, you can create custom validation rules that seamlessly integrate with CakePHP’s existing validation framework. Remember that custom validators should be designed to improve the accuracy and reliability of your application’s data while adhering to your specific business logic.
Incorporating custom validators not only enhances data validation but also contributes to the overall robustness and stability of your web application. As you continue to develop and refine your application, having the ability to create tailored validation rules will prove to be a valuable asset in maintaining data integrity.
So go ahead, leverage the power of custom validators, and ensure that your CakePHP application’s data remains accurate and dependable. Happy coding!
Table of Contents
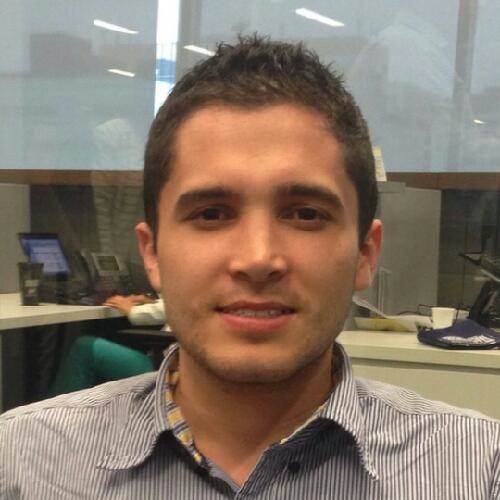
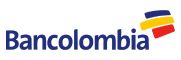