Creating Custom CakePHP View Helpers
CakePHP is a popular PHP framework known for its flexibility and rapid development capabilities. One of its standout features is the ability to create custom view helpers, which can simplify your code, improve reusability, and enhance the user experience of your web applications. In this guide, we will explore the world of custom CakePHP view helpers, from understanding their significance to creating and implementing them effectively.
Table of Contents
1. Why Use Custom View Helpers?
Before delving into the process of creating custom view helpers in CakePHP, let’s understand why they are essential and how they can benefit your application development.
1.1. Code Reusability
Custom view helpers allow you to encapsulate pieces of code that you use frequently throughout your application. By doing so, you can avoid code duplication and simplify your views, making them easier to maintain.
1.2. Enhance Readability
Separating complex logic from your views and controllers improves code readability. Custom view helpers enable you to organize code logically and create clean, concise templates.
1.3. Promote Consistency
View helpers help maintain consistency in your application’s user interface. You can ensure that UI elements, such as form inputs or buttons, adhere to a specific style or behavior across your entire application.
1.4. Testing Made Easier
By isolating functionality within view helpers, you can write unit tests for these components, ensuring they work as expected. This simplifies the testing process and increases the reliability of your application.
Now that we understand the benefits of custom view helpers, let’s explore how to create them in CakePHP.
2. Creating a Custom View Helper
Creating a custom view helper in CakePHP involves a few key steps. We’ll walk you through each of them to help you get started.
2.1. Create the Helper File
The first step is to create the helper file itself. You should place this file in the src/View/Helper directory of your CakePHP application. The file name should follow the convention of MyCustomHelper.php, where “MyCustomHelper” is the name you choose for your helper.
Here’s a basic structure for your custom helper file:
php // src/View/Helper/MyCustomHelper.php namespace App\View\Helper; use Cake\View\Helper; class MyCustomHelper extends Helper { // Helper code goes here }
2.2. Define Helper Methods
Inside your custom helper class, you can define methods that encapsulate the functionality you want to provide. These methods can be used in your views to simplify common tasks.
Let’s create a simple example where we’ll create a custom helper for formatting dates:
php namespace App\View\Helper; use Cake\View\Helper; class MyCustomHelper extends Helper { // Format a date public function formatDate($date) { return date('F j, Y', strtotime($date)); } }
In this example, we’ve created a formatDate method that takes a date as input and returns a formatted date string. You can define any number of methods in your custom helper to suit your application’s needs.
2.3. Loading the Helper
To make your custom helper available in your views and controllers, you need to load it. You can do this in your controller’s initialize method or in your AppView class.
2.3.1. Loading in a Controller
To load your custom helper in a controller, open the controller’s initialize method and add the following code:
php use App\View\Helper\MyCustomHelper; // ... public function initialize() { parent::initialize(); $this->loadHelper('MyCustom'); }
In this code, we use the loadHelper method to load our custom helper named “MyCustom.”
2.3.2. Loading in AppView
If you want to make your custom helper available globally throughout your application, you can load it in the AppView class, located at src/View/AppView.php. Add the following code to the initialize method of your AppView class:
php use App\View\Helper\MyCustomHelper; // ... public function initialize() { parent::initialize(); $this->loadHelper('MyCustom'); }
Now, your custom helper is accessible in all views and controllers within your CakePHP application.
2.4. Using the Custom Helper in Views
With your custom helper loaded, you can now use its methods in your views. Let’s see how we can use the formatDate method we defined earlier:
php <?= $this->MyCustom->formatDate($article['published_date']) ?>
In this example, we use the helper’s formatDate method to format the published_date of an article.
3. Advanced Usage: Helper Configuration
While the basic steps outlined above are sufficient for creating simple custom helpers, you may encounter scenarios where you need more advanced functionality or configuration options. CakePHP provides several features to accommodate these needs.
3.1. Helper Configuration Array
You can pass a configuration array to your custom helper when loading it. This array can contain various options that your helper may need. For example, if your helper needs to fetch data from an external API, you can provide the API endpoint as a configuration option.
Here’s how to load your custom helper with a configuration array:
php $this->loadHelper('MyCustom', ['apiEndpoint' => 'https://example.com/api/data']);
Inside your custom helper, you can access this configuration like so:
php namespace App\View\Helper; use Cake\View\Helper; class MyCustomHelper extends Helper { protected $_defaultConfig = [ 'apiEndpoint' => 'https://example.com/api/data', ]; public function initialize(array $config): void { parent::initialize($config); // Access the configuration option $apiEndpoint = $this->getConfig('apiEndpoint'); } // Helper code goes here }
By providing a configuration array, you make your custom helper more flexible and adaptable to different scenarios.
3.2. Helper Events
CakePHP also allows you to define events within your custom helper, which can be triggered at specific points in your application’s lifecycle. This is useful when you want to execute certain code before or after rendering a view.
Here’s an example of defining an event in your custom helper:
php namespace App\View\Helper; use Cake\Event\EventInterface; use Cake\View\Helper; class MyCustomHelper extends Helper { public function beforeRender(EventInterface $event) { // Code to execute before rendering } public function afterRender(EventInterface $event) { // Code to execute after rendering } // Helper code goes here }
You can then attach your custom helper’s events in your controller or view to execute the defined code at the appropriate times.
3.3. Helper Dependencies
In some cases, your custom helper may depend on other helpers or components. You can specify these dependencies in your helper’s initialize method using the setConfig method:
php public function initialize(array $config): void { parent::initialize($config); // Set dependencies $this->setConfig('helpers', ['Html', 'Form']); }
By setting dependencies, you ensure that the required helpers or components are loaded when your custom helper is used.
4. Best Practices for Custom Helpers
As you work with custom helpers in CakePHP, it’s important to follow best practices to ensure maintainable and efficient code.
4.1. Keep It Focused
Each custom helper should have a specific purpose and focus on a particular set of related functionality. Avoid creating monolithic helpers that try to do too much.
4.2. Use Dependency Injection
When possible, use dependency injection to inject dependencies (other helpers, components, or services) into your custom helper rather than hardcoding them.
4.3. Write Unit Tests
To ensure the reliability of your custom helper, write unit tests for its methods. CakePHP provides a testing framework that makes it easy to write and run tests.
4.4. Document Your Helpers
Properly document your custom helpers by adding comments and descriptions for each method. This makes it easier for other developers (including your future self) to understand and use your helpers.
4.5. Reuse Existing Helpers
Before creating a custom helper, check if CakePHP already provides a built-in helper or component that can fulfill your needs. Reusing existing functionality can save you time and effort.
Conclusion
Custom view helpers are a powerful tool in CakePHP that can greatly enhance the maintainability and functionality of your web applications. By encapsulating reusable code, promoting consistency, and improving readability, custom helpers can streamline your development process.
In this guide, we’ve explored the process of creating custom view helpers in CakePHP, from defining helper methods to loading and using them in your application. We’ve also covered advanced topics such as helper configuration, events, and dependencies, along with best practices to follow when working with custom helpers.
As you continue to develop your CakePHP applications, consider how custom view helpers can help you build cleaner, more maintainable code, and make the most of this powerful feature offered by the framework.
Table of Contents
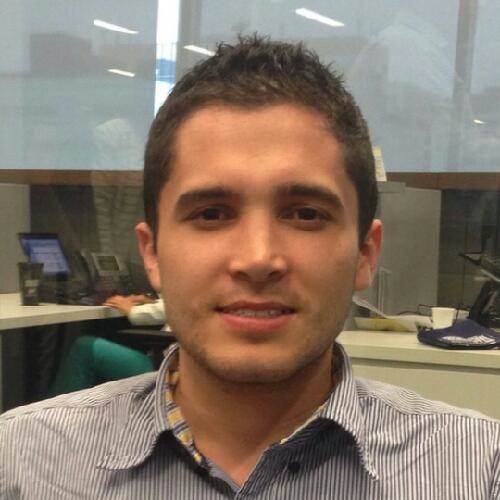
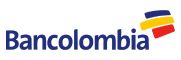