CakePHP Database Operations: CRUD Made Easy
When it comes to web development, database operations are an essential part of any application. Performing CRUD (Create, Read, Update, Delete) operations on the database is a common task that developers encounter regularly. However, managing these operations efficiently can be complex and time-consuming. This is where CakePHP, a popular PHP framework, comes to the rescue with its powerful database handling capabilities. In this article, we will explore how CakePHP simplifies CRUD operations, making database management a breeze.
What is CakePHP?
CakePHP is an open-source web application framework written in PHP, designed to facilitate rapid development and maintenance of web applications. It follows the Model-View-Controller (MVC) architectural pattern, providing a solid foundation for building robust and scalable applications. One of the standout features of CakePHP is its built-in ORM (Object-Relational Mapping) system, which greatly simplifies database interactions.
Understanding the ORM
CakePHP’s ORM acts as a bridge between your application code and the database, abstracting away the complexities of SQL queries. With the ORM, you can interact with the database using object-oriented syntax, making your code more readable and maintainable. The ORM automatically generates SQL queries based on your code, allowing you to perform CRUD operations without having to write raw SQL statements.
CRUD Operations in CakePHP
1. Creating Data (Insert)
Creating new records in the database is a fundamental CRUD operation. With CakePHP, inserting data into the database is a straightforward process. Let’s take a look at an example:
php // Create a new entity $article = $this->Articles->newEntity(); // Set the entity's properties $article->title = 'New Article'; $article->body = 'This is the content of the new article.'; // Save the entity if ($this->Articles->save($article)) { echo 'Article saved successfully.'; } else { echo 'Failed to save the article.'; }
In the code snippet above, we create a new entity using the newEntity() method. We then set the properties of the entity, such as the title and body. Finally, we use the save() method to persist the entity into the database. If the save operation is successful, a success message is displayed; otherwise, an error message is shown.
2. Reading Data (Retrieve)
Retrieving data from the database is a common task in almost every application. CakePHP provides several ways to fetch data from the database, including finding a single record, finding multiple records, and custom queries.
To find a single record, you can use the get() method:
php // Retrieve a single article by its ID $article = $this->Articles->get($id);
To find multiple records based on specific conditions, you can use the find() method:
php // Find all published articles $articles = $this->Articles->find('all', [ 'conditions' => ['published' => true] ]);
You can also execute custom queries using the query() method:
php // Execute a custom query $query = $this->Articles->query('SELECT * FROM articles WHERE published = true'); $articles = $query->fetchAll('assoc');
3. Updating Data
Updating existing records in the database is another essential CRUD operation. CakePHP provides a simple and intuitive way to update data. Here’s an example:
php // Get the article by its ID $article = $this->Articles->get($id); // Update the article's properties $article->title = 'Updated Title'; $article->body = 'This is the updated content of the article.'; // Save the changes if ($this->Articles->save($article)) { echo 'Article updated successfully.'; } else { echo 'Failed to update the article.'; }
In the code snippet above, we retrieve the article using the get() method, update its properties, and save the changes using the save() method. The save() method automatically detects changes and updates the corresponding record in the database.
4. Deleting Data
Deleting data from the database is a crucial operation in any application. With CakePHP, deleting records is a breeze. Here’s how it’s done:
php // Get the article by its ID $article = $this->Articles->get($id); // Delete the article if ($this->Articles->delete($article)) { echo 'Article deleted successfully.'; } else { echo 'Failed to delete the article.'; }
In the code snippet above, we retrieve the article using the get() method and delete it using the delete() method. The delete() method removes the corresponding record from the database.
Conclusion
CakePHP simplifies database operations by providing a robust ORM system and built-in functionalities for performing CRUD operations. The framework’s intuitive syntax and powerful features make it easy to manage data in your applications without the need for writing complex SQL queries. Whether you’re creating, reading, updating, or deleting data, CakePHP streamlines the process, allowing you to focus on building your application’s logic. By leveraging the power of CakePHP’s database handling capabilities, you can save time and effort while building scalable and maintainable web applications.
Table of Contents
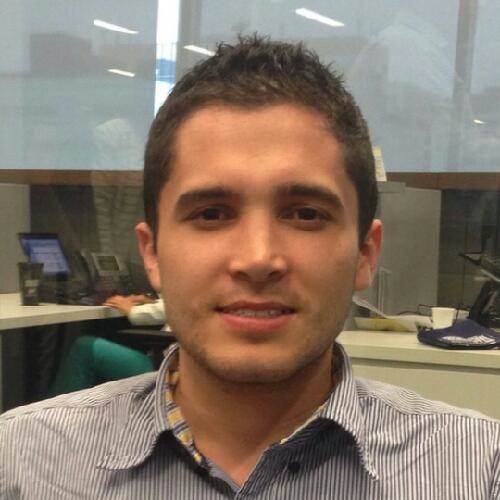
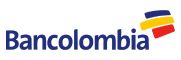