Using CakePHP’s DebugKit: Profiling and Debugging Tools
Debugging and profiling are essential aspects of software development that help identify and resolve issues, optimize performance, and ensure the smooth functioning of applications. When working with the CakePHP framework, one powerful tool at your disposal is the DebugKit. DebugKit is a comprehensive debugging and profiling plugin that provides valuable insights into the behavior of your CakePHP application, helping you identify bottlenecks, optimize queries, and streamline your development process. In this blog post, we’ll explore how to leverage CakePHP’s DebugKit to enhance your development experience by diving into its profiling and debugging tools.
Table of Contents
1. What is DebugKit?
Before we delve into the details, let’s briefly understand what DebugKit is. DebugKit is a developer-oriented plugin specifically designed for CakePHP applications. It offers a suite of tools that aid in debugging, profiling, and understanding the inner workings of your application. DebugKit is not included in production environments, making it a powerful tool for development and troubleshooting purposes without affecting your live application’s performance.
2. Installing DebugKit
Getting started with DebugKit is a straightforward process. Assuming you already have a CakePHP application up and running, follow these steps to install and configure DebugKit:
- Open a terminal window and navigate to your CakePHP project’s root directory.
- Run the following Composer command to install DebugKit:
bash composer require --dev cakephp/debug_kit
3. Once the installation is complete, load the DebugKit plugin by adding the following line to your config/bootstrap.php file:
php Plugin::load('DebugKit');
4. Make sure that the Debug toolbar is enabled in your development environment. Open the config/app.php file and ensure that the ‘debug’ key is set to true:
php 'debug' => true,
5. Finally, clear your application’s cache to ensure that DebugKit is properly integrated:
bash bin/cake cache clear_all
With DebugKit installed and configured, you’re now ready to explore its powerful features.
3. Profiling Your Application
Profiling is the process of analyzing your application’s performance to identify areas that require optimization. DebugKit’s profiling tools provide you with valuable insights into the execution time, memory usage, and database queries of your CakePHP application.
3.1. Using the Toolbar
The DebugKit toolbar is the heart of the plugin. It appears at the top of your application’s pages when you’re in the development environment. The toolbar displays essential information, such as the elapsed time, memory usage, and number of database queries made during the request.
To use the toolbar effectively, follow these steps:
- Load a page of your CakePHP application in your browser while in the development environment.
- Observe the DebugKit toolbar at the top of the page. It displays various tabs, including Timer, Memory, Logs, and Sql Log.
- Click on each tab to access detailed information about the request’s execution time, memory consumption, application logs, and executed SQL queries.
3.2. Analyzing SQL Queries
One of the key features of DebugKit is its ability to capture and display executed SQL queries, helping you identify potential performance bottlenecks and inefficient queries. To analyze SQL queries using DebugKit:
- Load a page of your application that involves database interactions.
- Click on the Sql Log tab in the DebugKit toolbar.
- Review the list of executed SQL queries, including their execution time and the number of rows returned.
- Identify any queries that take a significant amount of time or return a large number of rows. These are potential candidates for optimization.
3.3. Understanding Execution Time and Memory Usage
The Timer and Memory tabs in the DebugKit toolbar provide valuable insights into the time and memory consumption of your application’s requests. This information is crucial for identifying performance bottlenecks and memory-intensive operations.
To interpret the data from these tabs:
- Load a page of your application.
- Click on the Timer tab to see a breakdown of the time spent on various aspects of the request, such as the controller action, rendering, and database queries.
- Click on the Memory tab to view the memory usage during the request’s lifecycle, including the peak memory consumption.
- Identify any significant spikes in execution time or memory usage, as these could indicate areas for optimization.
4. Debugging with DebugKit
Debugging is a critical part of the development process, helping you identify and fix issues within your application’s code. DebugKit offers various debugging tools that facilitate the process of locating and resolving errors.
4.1. Accessing Logs
CakePHP’s Logs tab within the DebugKit toolbar allows you to access logs generated during the request. These logs provide valuable information about the application’s behavior and can help pinpoint errors and unexpected behavior.
To access logs using DebugKit:
- Load a page of your application.
- Click on the Logs tab in the DebugKit toolbar.
- Review the logs to identify any warning messages, errors, or unexpected behavior.
- Use the log entries to diagnose and address issues within your application.
4.2. Custom Debug Messages
DebugKit also allows you to add custom debug messages to your application’s pages, helping you print variables, messages, and other information for troubleshooting purposes.
To add custom debug messages:
- Within your controller or view, use the DebugKit\DebugTimer::start() and DebugKit\DebugTimer::stop() methods to measure the execution time of specific code blocks.
php // Start the timer DebugKit\DebugTimer::start('myCustomTimer'); // Code to be measured // Stop the timer DebugKit\DebugTimer::stop('myCustomTimer');
2. To add custom debug messages, use the DebugKit\Debugger::dump() method:
php // Dump a variable with a custom label DebugKit\Debugger::dump($myVariable, 'My Custom Variable');
4.3. Debugging Queries
DebugKit’s Sql Log tab is not only useful for profiling but also for debugging database queries. It allows you to examine the executed SQL queries in detail, helping you identify issues with your database interactions.
To debug queries using DebugKit:
- Load a page of your application involving database interactions.
- Click on the Sql Log tab in the DebugKit toolbar.
- Review the executed SQL queries and their associated execution times.
- Identify any queries that seem incorrect or are not producing the expected results.
- Use the information from the Sql Log to refine your queries and resolve any database-related issues.
Conclusion
Debugging and profiling are integral components of the development process, ensuring the reliability, performance, and functionality of your CakePHP applications. DebugKit offers a powerful set of tools that make the debugging and profiling process more efficient and effective. By utilizing the DebugKit toolbar, analyzing SQL queries, understanding execution time and memory usage, and leveraging the various debugging features, you can streamline your development workflow, identify and resolve issues, and create high-quality CakePHP applications.
In this blog post, we’ve only scratched the surface of what DebugKit has to offer. As you continue to work with CakePHP and explore more complex scenarios, DebugKit will prove to be an indispensable companion in your journey to building robust and optimized applications. So, don your developer’s cape and start supercharging your CakePHP development with the power of DebugKit!
Table of Contents
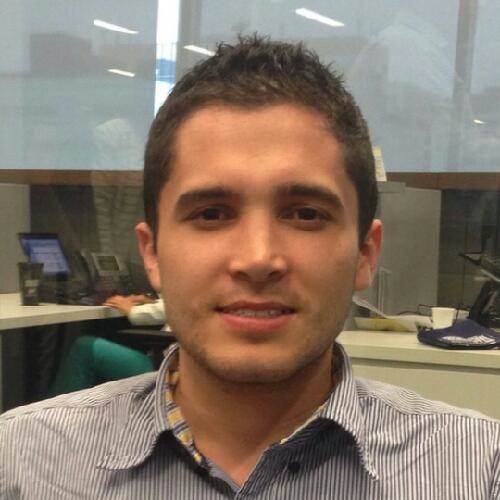
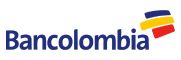