Mastering CakePHP Associations: Defining Relationships
If you’re a web developer using CakePHP, you know how powerful and flexible the framework is. One of its key features that make CakePHP stand out is its support for defining associations and relationships between models. These associations allow you to link and query related data effortlessly. In this blog post, we will delve into CakePHP associations and learn how to define relationships between models, enabling you to build more sophisticated and efficient applications.
1. Understanding CakePHP Associations
1.1 What are Associations?
Associations in CakePHP are a way to define relationships between models in your application. Models represent database tables, and associations allow you to link one model to another, establishing a connection between their data. This linkage enables you to retrieve related data easily and efficiently, without writing complex SQL queries manually.
1.2 Why are Associations Important?
Associations simplify database queries by abstracting the underlying SQL, making your code more readable and maintainable. They also help you follow the Don’t Repeat Yourself (DRY) principle, as you don’t need to write redundant code for querying related data. Additionally, using associations enhances code reusability and ensures consistency in your application’s data handling.
1.3 Types of Associations in CakePHP
CakePHP supports four types of associations:
- BelongsTo: Represents a “parent-to-child” relationship. For example, if you have an “Article” model and a “Category” model, each article belongs to a single category.
- HasOne: Represents a “one-to-one” relationship. For instance, an “Order” model can have one associated “ShippingAddress” model.
- HasMany: Represents a “one-to-many” relationship. For example, a “User” model can have multiple “Post” models associated with it.
- BelongsToMany: Represents a “many-to-many” relationship. Suppose you have a “Product” model and a “Tag” model; each product can be associated with multiple tags, and each tag can be associated with multiple products.
2. Defining Associations in CakePHP
In CakePHP, defining associations involves setting up relationships between models. Let’s explore each association type with examples:
2.1 BelongsTo Association
The BelongsTo association is used to define a “parent-to-child” relationship. To establish this association, you need to add a foreign key to the “child” model, referencing the “parent” model’s primary key.
php // In User.php (Child Model) class User extends AppModel { public $belongsTo = array( 'Role' => array( 'className' => 'Role', 'foreignKey' => 'role_id' ) ); } php Copy code // In Role.php (Parent Model) class Role extends AppModel { public $hasMany = array( 'User' => array( 'className' => 'User', 'foreignKey' => 'role_id' ) ); }
With this setup, each user belongs to a specific role, and each role can have multiple associated users.
2.2 HasOne Association
The HasOne association defines a “one-to-one” relationship between models. It is used when each record in the first model corresponds to exactly one record in the second model.
php // In Profile.php (Model) class Profile extends AppModel { public $hasOne = array( 'User' => array( 'className' => 'User', 'foreignKey' => 'profile_id' ) ); } php // In User.php (Model) class User extends AppModel { public $belongsTo = array( 'Profile' => array( 'className' => 'Profile', 'foreignKey' => 'profile_id' ) ); }
In this example, each user has one profile, and each profile belongs to a single user.
2.3 HasMany Association
The HasMany association defines a “one-to-many” relationship between models. It is used when each record in the first model can be associated with multiple records in the second model.
php // In Author.php (Model) class Author extends AppModel { public $hasMany = array( 'Book' => array( 'className' => 'Book', 'foreignKey' => 'author_id' ) ); } php // In Book.php (Model) class Book extends AppModel { public $belongsTo = array( 'Author' => array( 'className' => 'Author', 'foreignKey' => 'author_id' ) ); }
With this setup, each author can have multiple books, and each book belongs to a specific author.
2.4 BelongsToMany Association
The BelongsToMany association establishes a “many-to-many” relationship between models. This association is used when each record in the first model can be associated with multiple records in the second model, and vice versa.
php // In Product.php (Model) class Product extends AppModel { public $hasAndBelongsToMany = array( 'Tag' => array( 'className' => 'Tag', 'joinTable' => 'products_tags', 'foreignKey' => 'product_id', 'associationForeignKey' => 'tag_id' ) ); } php // In Tag.php (Model) class Tag extends AppModel { public $hasAndBelongsToMany = array( 'Product' => array( 'className' => 'Product', 'joinTable' => 'products_tags', 'foreignKey' => 'tag_id', 'associationForeignKey' => 'product_id' ) ); }
In this example, each product can have multiple tags, and each tag can be associated with multiple products.
3. Setting Up the Database and Models
Before you can define associations, you need to set up your database tables and model classes. Let’s go through the steps required to get everything ready:
3.1 Creating Database Tables
Suppose we want to create a simple blog application with two models: “Posts” and “Categories.” The “posts” table will store information about blog posts, while the “categories” table will contain data about different blog categories.
sql -- posts table CREATE TABLE posts ( id INT PRIMARY KEY AUTO_INCREMENT, title VARCHAR(255), body TEXT, category_id INT ); -- categories table CREATE TABLE categories ( id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(100) );
3.2 Defining Model Classes
After creating the database tables, you need to define the model classes in CakePHP. Each model class represents a database table and will contain the associations.
php // In Post.php (Model) class Post extends AppModel { public $belongsTo = array( 'Category' => array( 'className' => 'Category', 'foreignKey' => 'category_id' ) ); } php // In Category.php (Model) class Category extends AppModel { public $hasMany = array( 'Post' => array( 'className' => 'Post', 'foreignKey' => 'category_id' ) ); }
3.3 Setting up Relationships
Finally, in the bootstrap or any other appropriate configuration file, you must load the model classes and set up the database connection.
php // In Config/bootstrap.php CakePlugin::loadAll(); ConnectionManager::config('default', array( 'datasource' => 'Database/Mysql', 'persistent' => false, 'host' => 'localhost', 'login' => 'username', 'password' => 'password', 'database' => 'database_name', 'prefix' => '', 'encoding' => 'utf8' )); App::uses('AppModel', 'Model'); App::uses('AppController', 'Controller');
With the database and models set up, we can now move on to fetching related data using associations.
4. Fetching Related Data with Associations
CakePHP provides two approaches for fetching related data: Containable Behavior and Eager Loading (via find() options). Additionally, associations support Lazy Loading, where related data is loaded only when accessed.
4.1 Containable Behavior
The Containable Behavior allows you to specify the depth of associated data you want to retrieve when querying. This feature helps you control the amount of data retrieved and optimize your queries.
php // In PostsController.php public function view($id) { $this->Post->Behaviors->load('Containable'); $post = $this->Post->find('first', array( 'conditions' => array('Post.id' => $id), 'contain' => array('Category') )); $this->set('post', $post); }
4.2 Eager Loading
Eager Loading is another approach to fetch related data in a single query. It is often more efficient than lazy loading as it reduces the number of database queries needed.
php // In PostsController.php public function view($id) { $post = $this->Post->find('first', array( 'conditions' => array('Post.id' => $id), 'recursive' => 1 )); $this->set('post', $post); }
In this example, the recursive option of find() is set to 1, which fetches associated data.
4.3 Lazy Loading
Lazy Loading is the default behavior in CakePHP. When you load a model, its associations are not fetched immediately. Instead, they are retrieved from the database only when accessed.
php // In PostsController.php public function view($id) { $post = $this->Post->findById($id); $category = $post['Category']; // Lazy loading occurs here $this->set('post', $post); }
5. Working with Cascade Operations
Cascade operations are operations performed on associated records when certain events occur, such as deleting a parent record or updating a foreign key value.
5.1 Cascading Deletes
Cascading deletes allow you to delete associated records when the parent record is deleted. This is useful to maintain data integrity and prevent orphaned records.
php // In User.php (Model) class User extends AppModel { public $hasMany = array( 'Post' => array( 'className' => 'Post', 'foreignKey' => 'user_id', 'dependent' => true // Cascading delete ) ); }
In this example, when a user is deleted, all associated posts will also be deleted.
5.2 Cascading Updates
Cascading updates allow you to automatically update associated records when the parent record is updated.
php // In User.php (Model) class User extends AppModel { public $hasMany = array( 'Post' => array( 'className' => 'Post', 'foreignKey' => 'user_id', 'cascadeCallbacks' => true // Cascading update ) ); }
With this setup, if the user’s primary key is updated, all associated posts will have their foreign key updated accordingly.
6. Additional Association Options
CakePHP provides several additional options to customize associations further.
6.1 Customizing Association Keys
By default, CakePHP uses naming conventions for foreign keys and association keys. However, you can manually set these keys if your database follows a different naming pattern.
php // In Book.php (Model) class Book extends AppModel { public $belongsTo = array( 'Author' => array( 'className' => 'Author', 'foreignKey' => 'writer_id', 'associationForeignKey' => 'creator_id' ) ); }
6.2 Conditions and Fields
You can use the conditions and fields options to filter and limit the associated data fetched from the database.
php // In Category.php (Model) class Category extends AppModel { public $hasMany = array( 'Post' => array( 'className' => 'Post', 'foreignKey' => 'category_id', 'conditions' => array('Post.published' => true), 'fields' => array('Post.id', 'Post.title') ) ); }
In this example, only published posts will be fetched, and only the id and title fields of posts will be retrieved.
6.3 Polymorphic Associations
Polymorphic associations allow a model to belong to more than one other model on a single association key. This is useful when multiple models need to be linked to a common model.
php // In Comment.php (Model) class Comment extends AppModel { public $belongsTo = array( 'Commentable' => array( 'polymorphic' => true ) ); }
With this setup, the Comment model can belong to multiple models like Post, User, etc., using the same Commentable association key.
Conclusion
Mastering CakePHP associations and defining relationships between models is a fundamental skill for building robust and efficient applications. With the power of associations, you can easily query and manipulate related data without writing complex SQL queries. In this blog post, we explored the four types of associations in CakePHP: BelongsTo, HasOne, HasMany, and BelongsToMany. We also learned how to set up database tables and model classes to establish relationships. Additionally, we looked into fetching related data using Containable Behavior and Eager Loading, and we covered cascade operations and additional association options.
Now that you have a solid understanding of CakePHP associations, you can leverage this knowledge to design more sophisticated and interconnected applications with ease. Happy coding!
Table of Contents
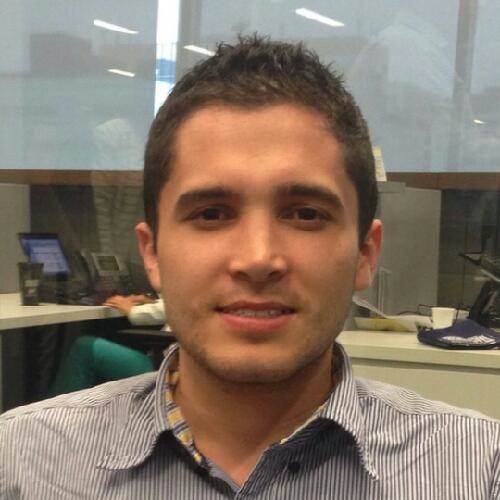
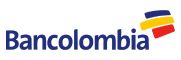